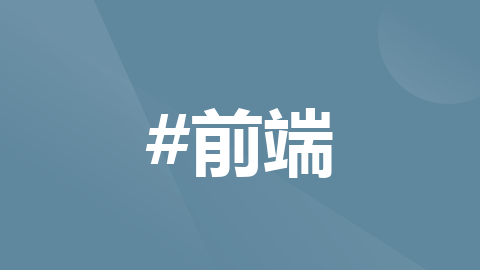
Vue3+Ant Design表格排序
vue
vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
项目地址:https://gitcode.com/gh_mirrors/vu/vue

·
最近在公司做有关报表的项目时,遇到最多的问题-表格排序,刚开始看到UI设计图的时候,还有些纳闷这个排序如何做,其实实际上并没有想象中的那么难,如果说单纯的排序的话ant design这个组件里的表格有自带的排序和筛选功能,但是数据来源于后端,所以操作起来可能不能只考虑前端的功能,强烈推荐需要做有关后台系统这块项目的小伙伴可以去使用这个组件,他有react版本的及Vue版本的,可自行依据使用的框架进行选择。
一,安装ant design
我这里使用的是Vue框架,所以就根据Vue来进行以下步骤,特别提醒在安装这个插件之前一定要先安装nodejs。@后面是安装具体的版本号,这个依据自身需要酌情选择即可。
npm i --save ant-design-vue@4.x
二、全局注册
以下代码便完成了 Antd 的全局注册。需要注意的是,样式文件需要单独引入。
import { createApp } from 'vue';
import Antd from 'ant-design-vue';
import App from './App';
import 'ant-design-vue/dist/reset.css';
const app = createApp(App);
app.use(Antd).mount('#app');
三、项目中引用Antd、并实现排序功能
这里以使用表格为例
(1)组件部分
<template>
<div class="operat-tab">
<a-table
:columns="columns"
:dataSource="tableData"
:pagination="false"
bordered
@change="handleChange"
:scroll="{ y: 'calc(100vh - 520px)' }"
/>
<a-pagination
v-model:current="page.currentPage"
v-model:page-size="page.pageSize"
show-quick-jumper
show-size-changer
:total="pageData.total"
:show-total="(total, range) => `共 ${props.pageData.total || 0} 条`"
/>
</div>
</template>
(2)script部分
<script setup>
// 需要排序某个字段
const flag = ref()
// 排序标识
const sort = ref()
// 分页
const page = ref({})
// 表格头部信息
const columns = ref([
{
title: '序号',
dataIndex: 'index',
align: 'center',
key: 'index'
},
{
title: '等级',
dataIndex: 'level',
align: 'center',
key: 'level'
},
{
title: '路段名称',
dataIndex: 'road',
align: 'center',
key: 'road'
},
{
title: '桩号',
dataIndex: 'stake',
align: 'center',
key: 'stake'
},
{
title: '营运单位',
dataIndex: 'address',
align: 'center',
key: 'address'
},
{
title: '开始时间',
dataIndex: 'startTime',
align: 'center',
key: '1',//利用key值来获取需要排序的字段名
sorter: true
},
{
title: '时长(分钟)',
dataIndex: 'time',
align: 'center',
key: '2',
sorter: true
},
{
title: '长度(米)',
dataIndex: 'length',
align: 'center',
key: '3',
sorter: true
},
{
title: '速度(千米/小时)',
dataIndex: 'speed',
align: 'center',
key: '4',
sorter: true
}
])
// 改变asc dsc
const changeTable = (pagination, filters, sorter) => {
if (sorter.order === 'ascend') {
flag.value = sorter.columnKey
sort.value = 'ASC'
const params = {
sort: sort.value,
page: page.value
}
getCongestedData(params)
} else {
flag.value = sorter.columnKey
sort.value = 'DESC'
const params = {
flag: flag.value,
sort: sort.value,
page: page.value
}
getCongestedData(params)
}
}
// 获取表格数据,说明:数据来源于后端,这里仅限于模拟,params是需要传的参数
const getCongestedData = params => {
return new Promise((resolve, reject) => {
getCongestedTab(params)
.then(res => {
dataSource.value = []
if (res.code === 200 && res.data) {
const dataList = res.data.list
dataList.forEach((item, index) => {
dataSource.value.push({
index: index + 1,
level: item.congestDesc,
road: item.roadName,
stake: item.stake,
address: item.zoneName,
startTime: item.startTime,
time: item.duration,
length: item.length,
speed: item.speed
})
})
pageData.value = {
...pageData.value,
total: res.data.page.totalNum,
showTotal: () => `共 ${res.data.page.totalNum || 0} 条`,
current: res.data.page.currentPage
}
// console.log(res, '这个是')
} else {
dataSource.value = []
}
resolve(res.data)
})
.catch(error => {
reject(error)
})
})
}
</script>
(3)css部分
<style lang="less" scoped>
.operat-tab {
.ant-table-wrapper {
height: calc(100% - 70px);
}
.ant-pagination {
font-size: 14px;
margin-top: 10px;
}
.ant-pagination-item {
min-width: 32px;
height: 32px;
margin-right: 8px;
line-height: 30px;
}
.ant-pagination-prev,
.ant-pagination-next,
.ant-select-selector,
.ant-pagination-options-quick-jumper {
min-width: 28px;
height: 28px;
margin-top: 10px;
line-height: 28px;
font-size: 14px;
align-items: center;
input {
position: relative;
display: inline-block;
width: 100%;
min-width: 0;
padding: 4px 11px;
color: rgba(0, 0, 0, 0.85);
font-size: 14px;
line-height: 1.5715;
background-color: #fff;
background-image: none;
border: 1px solid #d9d9d9;
border-radius: 2px;
transition: all 0.3s;
width: 50px;
height: 32px;
margin: 0 8px;
}
}
::v-deep .ant-table {
max-height: calc(100vh - 3.4vw - 25px - 128px - 76px - 100px);
background: #f0f5ff;
}
::v-deep .ant-table-thead > tr > th {
background-color: #dee9ff;
}
::v-deep .ant-table-tbody tr:nth-child(2n) {
background-color: #fff;
}
::v-deep .ant-pagination.mini .ant-pagination-total-text {
flex: 1;
}
::v-deep .ant-pagination.mini .ant-pagination-item {
margin-left: 0.42vw;
}
}
</style>
四、展示
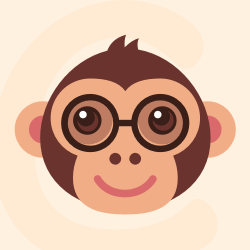



vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
最近提交(Master分支:2 个月前 )
73486cb5
* chore: fix link broken
Signed-off-by: snoppy <michaleli@foxmail.com>
* Update packages/template-compiler/README.md [skip ci]
---------
Signed-off-by: snoppy <michaleli@foxmail.com>
Co-authored-by: Eduardo San Martin Morote <posva@users.noreply.github.com> 4 个月前
e428d891
Updated Browser Compatibility reference. The previous currently returns HTTP 404. 5 个月前
更多推荐
所有评论(0)