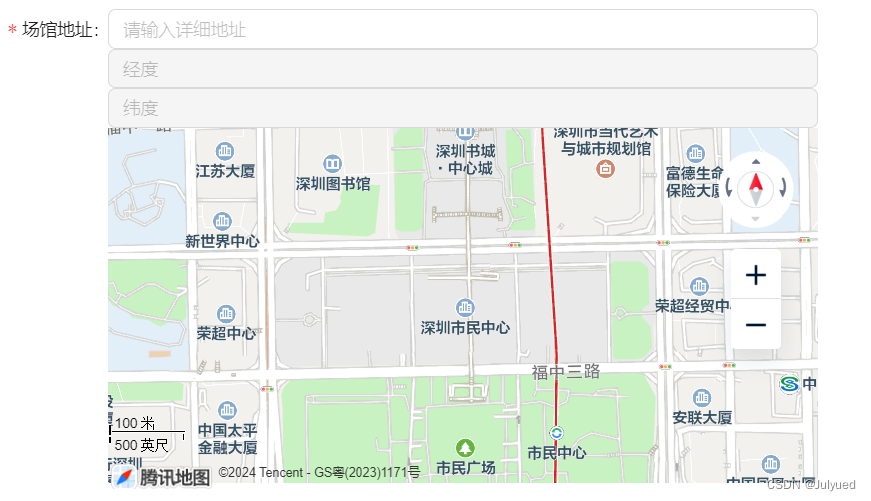
VUE3中antd引入腾讯地图,实现地点查询及获取经纬度
vue
vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
项目地址:https://gitcode.com/gh_mirrors/vu/vue

·
实现效果:
具体实现:1.申请腾讯开发者KEY
腾讯位置服务 - 立足生态,连接未来 注册登录后创建应用
2.在项目index.html中引入
<script charset="utf-8" src="https://map.qq.com/api/gljs?v=1.exp&key=申请的key值"></script>
3.页面中使用
表单输入框及地图绘制()
<a-form-item :name="['venue', 'address']" label="场馆地址" :rules="[{ required: true }]">
<a-auto-complete
v-model:value="formData.venue.address"
placeholder="请输入详细地址"
:options="mapList"
:trigger-on-focus="false"
@select="onSearch"
@search="querySearch"
value-key="title"
>
</a-auto-complete>
<a-input v-model:value="formData.venue.addressLongitude" placeholder="经度" width="100px" disabled/>
<a-input v-model:value="formData.venue.addressLatitude" placeholder="纬度" disabled/>
<div id="container" style="width: 100%; height: 100%"></div>
</a-form-item>
/**
* 表单字段
*/
const formData = reactive({
venue: {
address:''
addressLatitude: props.data?.addressLatitude,
addressLongitude:props.data?.addressLongitude
},
})
4.下载插件并引入 pnpm i vue-jsonp -S
import { jsonp } from "vue-jsonp";
5.方法及逻辑代码实现(如需求和我一样可直接复制使用)
const userlocation =reactive({
map: "",
markerLayer: "",
lng: "",
lat: "",
})
//地图开始----------
let suggestId = ref("suggestId")
let TMap = (window as any).TMap;
const initMap = () =>{
//定义地图中心点坐标
var center = new (window as any).TMap.LatLng(22.543731, 114.059589);
//定义map变量,调用 TMap.Map() 构造函数创建地图
userlocation.map = new (window as any).TMap.Map(document.getElementById("container"), {
center: center, //设置地图中心点坐标
zoom: 16, //设置地图缩放级别
viewMode: "3D",
})
// formData.venue.address = this.dialog.address;
addrToGetCoordinate(formData.venue.address);
(userlocation.map as any).on("click", clickHandler); // 绑定点击地图获取地理位置的事件
// this.addrToGetCoordinate(this.address_detail);
}
//标记地图
const markerLayer = (lat: any, lng: any)=> {
userlocation.markerLayer = new (window as any).TMap.MultiMarker({
map: userlocation.map, //指定地图容器
//样式定义
styles: {
//创建一个styleId为"myStyle"的样式(styles的子属性名即为styleId)
myStyle: new (window as any).TMap.MarkerStyle({
// width: 20, // 点标记样式宽度(像素)
// height: 30, // 点标记样式高度(像素)
// src: "https://mapapi.qq.com/web/lbs/javascriptGL/demo/img/marker-pink.png", //图片路径
//焦点在图片中的像素位置,一般大头针类似形式的图片以针尖位置做为焦点,圆形点以圆心位置为焦点
anchor: { x: 16, y: 32 },
}),
},
//点标记数据数组
geometries: [
{
id: "1", //点标记唯一标识,后续如果有删除、修改位置等操作,都需要此id
styleId: "myStyle", //指定样式id
position: new (window as any).TMap.LatLng(formData.venue.addressLatitude, formData.venue.addressLongitude), //点标记坐标位置
},
],
});
}
// 根据地址获取坐标
const addrToGetCoordinate =(address: any)=> {
const url = "https://apis.map.qq.com/ws/geocoder/v1/";
jsonp (url, {
key: "3WDBZ-HMUCX-NIE43-ZPLQ4-OOHAO-OKBES",
output: "jsonp",
address: address,
})
.then((res: { status: number; result: { location: { lat: number; lng: number; }; }; }) => {
console.log(res,'接口里面的数据??')
if (res.status === 0) {
// 处理得到的经纬度
formData.venue.addressLatitude = res.result.location.lat.toFixed(6);
formData.venue.addressLongitude = res.result.location.lng.toFixed(6);
nextTick(() => {
markerLayer( formData.venue.addressLatitude, formData.venue.addressLongitude); // 标记地图
setInitChange();
});
}
// else {
// console.log(22222, this.userlocation.lat, this.userlocation.lng);
// // 处理得到的经纬度
// // this.userlocation.lat = res.result.location.lat.toFixed(6);
// // this.userlocation.lng = res.result.location.lng.toFixed(6);
// // this.$nextTick(() => {
// // this.markerLayer(this.userlocation.lat, this.userlocation.lng); // 标记地图
// // this.setInitChange();
// // });
// }
})
.catch((e: any) => {
console.log(e);
});
}
// 修改地图位置
const setInitChange =() => {
(userlocation.map as any).setCenter(
new (window as any).TMap.LatLng(formData.venue.addressLatitude, formData.venue.addressLongitude)
);
(userlocation.markerLayer as any).updateGeometries([
{
styleId: "myStyle",
id: "1",
position: new (window as any).TMap.LatLng(formData.venue.addressLatitude, formData.venue.addressLongitude),
},
]);
}
const mapList = ref([])
// 获取搜索经纬度
const querySearch = (queryString, callback) => {
console.log(queryString,'3333333333')
// 关键字查询
const url = "https://apis.map.qq.com/ws/place/v1/suggestion"; // 关键字查询
jsonp(url, {
key: "3WDBZ-HMUCX-NIE43-ZPLQ4-OOHAO-OKBES",
keyword: queryString,
output: "jsonp",
}).then((res) => {
if (res.status == 0) {
// mapList.value = res.data;
let listss
listss = res.data.map(domain => {
return {
value: domain.title ? domain.title + '--' + domain.address : domain.address,
location: domain.location
}
})
mapList.value = listss
// callback(mapList.value);
}
else {
message.warning(res.message);
}
});
}
// 重载地图
const reloadMap = () => {
(document.getElementById("container") as any).innerHTML = "";
userlocation.markerLayer = "";
let center = new (window as any).TMap.LatLng(formData.venue.addressLatitude, formData.venue.addressLongitude);
userlocation.map = new (window as any).TMap.Map(document.getElementById("container"), {
center: center, //设置地图中心点坐标
viewMode: "3D",
});
}
// 点击获取经纬度
const clickHandler = (evt: any)=> {
let lat = evt.latLng.getLat().toFixed(6);
let lng = evt.latLng.getLng().toFixed(6);
formData.venue.addressLatitude = lng;
formData.venue.addressLongitude = lat;
//这里的evt有模糊的坐标可以打印看看但是没有街道所以我这做了判断
//如果有大的目标就取大的目标否则就取街道;
if (evt.poi) {
formData.venue.address = evt.poi.name;
//bug,点击出现多个标点
// this.addrToGetCoordinate(this.address_detail);
} else {
//调用详细地址函数
// console.log(this.userlocation.lng, this.userlocation.lat);
// getAreaCode();
}
// userlocation.markerLayer.geometries = [];
userlocation.markerLayer = "";
//修改id为4的点标记的位置
(userlocation.markerLayer as any).updateGeometries([
{
styleId: "marker",
id: "1",
position: new (window as any).TMap.LatLng(lat, lng),
},
]);
}
const onSearch = (item,val) => {
formData.venue.addressLatitude = val.location.lat;
formData.venue.addressLongitude = val.location.lng;
setInitChange();
}
//地图结束---------
最后即可实现最终效果
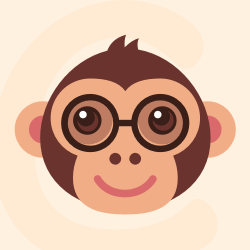



vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
最近提交(Master分支:1 个月前 )
73486cb5
* chore: fix link broken
Signed-off-by: snoppy <michaleli@foxmail.com>
* Update packages/template-compiler/README.md [skip ci]
---------
Signed-off-by: snoppy <michaleli@foxmail.com>
Co-authored-by: Eduardo San Martin Morote <posva@users.noreply.github.com> 3 个月前
e428d891
Updated Browser Compatibility reference. The previous currently returns HTTP 404. 3 个月前
更多推荐
所有评论(0)