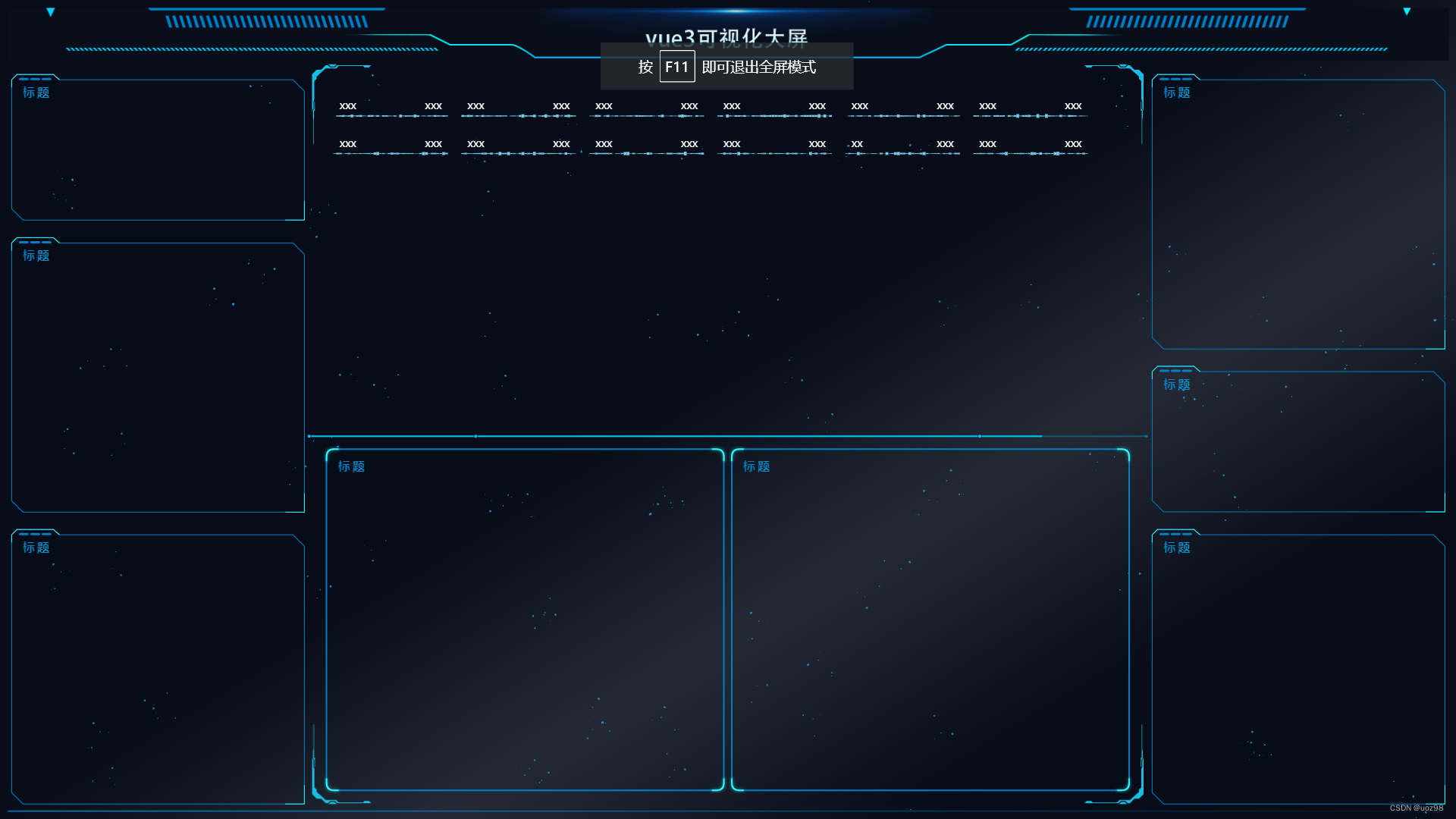
vue3+Echarts+DataV可视化大屏显示
vue
vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
项目地址:https://gitcode.com/gh_mirrors/vu/vue

·
未全屏时:
全屏时:
使用两个插件:
1.Echarts:Examples - Apache ECharts;安装:npm install echarts --save;
2.DataV:边框 | DataV;安装:npm install @jiaminghi/data-view;
先编写两个重要的组件:
第一个是全屏时撑满屏幕的组件(命名为:AdaptiveView.vue):
<template>
<div class="dataScreen-container">
<div ref="dataScreenRef" class="dataScreen-content">
<slot />
</div>
</div>
</template>
<script setup name="dataScreen">
import { ref, onMounted, onBeforeUnmount } from "vue";
const dataScreenRef = ref(null);
onMounted(() => {
if (dataScreenRef.value) {
dataScreenRef.value.style.transform = `scale(${getScale()}) translate(-50%, -50%)`;
dataScreenRef.value.style.width = `1920px`;
dataScreenRef.value.style.height = `1080px`;
}
window.addEventListener("resize", resize);
});
// 设置响应式
const resize = () => {
if (dataScreenRef.value) {
console.log("dataScreenRef.value", dataScreenRef.value);
dataScreenRef.value.style.transform = `scale(${getScale()}) translate(-50%, -50%)`;
}
};
// 根据浏览器大小推断缩放比例
const getScale = (width = 1920, height = 1080) => {
const ww = window.innerWidth / width;
const wh = window.innerHeight / height;
return ww < wh ? ww : wh;
};
onBeforeUnmount(() => {
window.removeEventListener("resize", resize);
});
</script>
<style lang="less" scoped>
.dataScreen-container {
width: 100%;
height: 100%;
.dataScreen-content {
position: fixed;
top: 50%;
left: 50%;
z-index: 999;
display: flex;
flex-direction: column;
overflow: hidden;
transition: all 0.3s;
transform-origin: left top;
}
}
</style>
第二个是包裹每一个chart的边框组件,边框组件编写了两个不同的样式。
第一个边框组件命名为:itemPage.vue:
<template>
<div>
<dv-border-box-13 class="dv-border-box" :color="['#007fc8', '#2cf7fe']" :style="{height:props.height+'px'}">
<p class="visual-texttitle">{{ props.boxTitle }}</p>
<slot></slot>
</dv-border-box-13>
</div>
</template>
<script setup>
const props = defineProps({
boxTitle: {
type: String,
default: "请输入标题",
},
height: {
type: Number,
default: 310,
},
});
</script>
<style lang="less" scoped>
.dv-border-box {
padding: 17px 10px;
margin-top: 15px;
}
.visual-texttitle {
font-size: 16px;
font-weight: 900;
letter-spacing: 3px;
background: linear-gradient(
92deg,
#007fc8 0%,
#2cf7fe 48.85253906%,
#2669a0 100%
);
-webkit-background-clip: text;
-webkit-text-fill-color: transparent;
margin: 0 10px 5px 10px;
text-align: left;
}
</style>
第二个边框组件命名为:itemPageFlex.vue:
<template>
<div>
<dv-border-box-12 class="dv-border-box" :color="['#007fc8', '#2cf7fe']">
<p class="visual-texttitle">{{ props.boxTitle }}</p>
<slot></slot>
</dv-border-box-12>
</div>
</template>
<script setup>
const props = defineProps({
boxTitle: {
type: String,
default: "",
},
});
</script>
<style lang="less" scoped>
.dv-border-box {
padding: 17px 10px;
height: 460px;
}
.visual-texttitle {
font-size: 16px;
font-weight: 900;
letter-spacing: 3px;
background: linear-gradient(
92deg,
#007fc8 0%,
#2cf7fe 48.85253906%,
#2669a0 100%
);
-webkit-background-clip: text;
-webkit-text-fill-color: transparent;
margin: 0 10px 5px 10px;
text-align: left;
}
</style>
接下来把所有组件组装起来(命名为:visualIndex.vue):
<template>
<AdaptiveView>
<div class="container">
<div class="visual-header">
<div class="header-img"></div>
</div>
<a-row class="visual-main flowing_light_lines">
<a-col :span="5">
<itemPage :boxTitle="'标题'" :height="200">
<!-- 放置编写好的组件 -->
</itemPage>
<itemPage :boxTitle="'标题'" :height="370">
<!-- 放置编写好的组件 -->
</itemPage>
<itemPage :boxTitle="'标题'" :height="370">
<!-- 放置编写好的组件 -->
</itemPage>
</a-col>
<a-col :span="14">
<dv-border-box-1 :color="['#007fc8', '#2cf7fe']">
<centerInfo />
<dv-decoration-10 class="center_decoration_line" style="height: 5px;" />
<a-row class="center_pie_flex">
<a-col :span="12">
<itemPageFlex
:boxTitle="'标题'"
:color="['#007fc8', '#2cf7fe']"
>
<!-- 放置编写好的组件 -->
</itemPageFlex>
</a-col>
<a-col :span="12">
<itemPageFlex
:boxTitle="'标题'"
:color="['#007fc8', '#2cf7fe']"
>
<!-- 放置编写好的组件 -->
</itemPageFlex>
</a-col>
</a-row>
</dv-border-box-1>
</a-col>
<a-col :span="5">
<itemPage :boxTitle="'标题'" :height="370">
<!-- 放置编写好的组件 -->
</itemPage>
<itemPage :boxTitle="'标题'" :height="200">
<!-- 放置编写好的组件 -->
</itemPage>
<itemPage :boxTitle="'标题'" :height="370">
<!-- 放置编写好的组件 -->
</itemPage>
</a-col>
</a-row>
</div>
</AdaptiveView>
</template>
<script setup>
import { onMounted,onBeforeUnmount } from "vue";
import itemPage from "./components/itemPage.vue";
import centerInfo from "./components/centerInfo.vue";
import itemPageFlex from "./components/itemPageFlex.vue";
import AdaptiveView from "../components/AdaptiveView.vue";
onMounted(() => {
document.body.style.backgroundColor = "#000";
});
onBeforeUnmount(()=>{
document.body.style.backgroundColor = "";
})
</script>
<style scoped>
.container{
//bg3.png 背景图是本人自己用ps瞎搞的
background: url("@/assets/image/bg3.png") no-repeat;
background-repeat: no-repeat;
background-attachment: fixed;
background-position: center;
background-size: 100% 100%;
background-size: cover;
background-color: #070d18;
color: #ffffff;
width: 100%;
height: 100%;
padding: 10px;
}
.header-img {
min-height: 70px;
width: 100%;
//headerTest.png 标题图是本人自己找的源文件用ps瞎搞的
background: url("@/assets/image/headerTest.png");
background-size: cover;
background-repeat: no-repeat;
background-position: center;
}
.center_pie_flex {
margin: 10px 20px;
}
.flowing_light_lines {
position: relative;
text-align: center;
overflow: hidden;
padding-bottom: 5px;
}
.flowing_light_lines::after {
content: "";
position: absolute;
bottom: 0;
left: 0;
width: 100%;
height: 1.5px;
background: linear-gradient(
to right,
#007fc8,
rgba(255, 255, 255, 0) 50%,
#007fc8
);
background-size: 200% 100%;
animation: glow 2s infinite alternate ease-in-out;
}
@keyframes glow {
from {
background-position: 0 100%;
}
to {
background-position: 200% 100%;
}
}
</style>
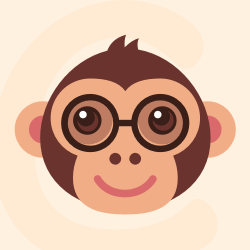



vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
最近提交(Master分支:6 个月前 )
9e887079
[skip ci] 4 个月前
73486cb5
* chore: fix link broken
Signed-off-by: snoppy <michaleli@foxmail.com>
* Update packages/template-compiler/README.md [skip ci]
---------
Signed-off-by: snoppy <michaleli@foxmail.com>
Co-authored-by: Eduardo San Martin Morote <posva@users.noreply.github.com> 8 个月前
更多推荐
所有评论(0)