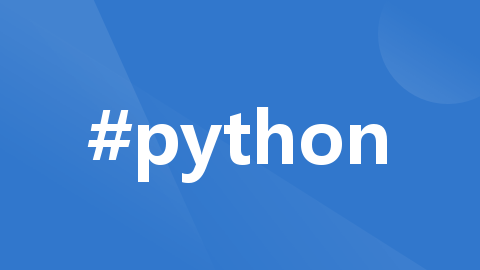
Python cv2(Opencv) 图像基本操作

简介:
OpenCV是一个基于Apache2.0许可(开源)发行的跨平台计算机视觉和机器学习软件库,可以运行在Linux、Windows、Android和Mac OS操作系统上。它轻量级而且高效,由一系列 C 函数和少量 C++ 类构成,同时提供了Python、Ruby、MATLAB等语言的接口,实现了图像处理和计算机视觉方面的很多通用算法。OpenCV用C++语言编写,它具有C ++,Python,Java和MATLAB接口,并支持Windows,Linux,Android和Mac OS,OpenCV主要倾向于实时视觉应用,并在可用时利用MMX和SSE指令, 如今也提供对于C#、Ch、Ruby,GO的支持。本文主要介绍Python 中使用cv2(Opencv) 进行图像的基本操作的方法及示例代码。
1、安装cv2(OpenCV)
1)安装numpy
pip install numpy
2)安装opencv-python
pip install opencv-python
3)安装opencv-contrib-python
pip install opencv-contrib-python
注意:opencv-python
和opencv-contrib-python
的版本需要使用相同的版本。
2、使用cv2(OpenCV)读取图像数据
cv2.IMREAD_COLOR
:彩色图像
cv2.IMREAD_GRAYSCALE
:灰度图像
使用OpenCV读取图像数据,代码如下,
import cv2 #opencv读取的格式是BGR
import matplotlib.pyplot as plt
import numpy as np
img=cv2.imread('cjavapy.jpg')
print(img)
#图像的显示,也可以创建多个窗口
cv2.imshow('image',img)
# 等待时间,毫秒级,0表示任意键终止
cv2.waitKey(0)
cv2.destroyAllWindows()
print(img.shape)
img=cv2.imread('cjavapy.jpg',cv2.IMREAD_GRAYSCALE)
print(img)
print(img.shape)
#图像的显示,也可以创建多个窗口
cv2.imshow('image',img)
# 等待时间,毫秒级,0表示任意键终止
cv2.waitKey(10000)
cv2.destroyAllWindows()
#保存
cv2.imwrite('mypic.png',img)
print(type(img)))
print(img.size)
print(img.dtype)
3、使用cv2(OpenCV)读取视频数据
使用cv2.VideoCapture()
可以捕获摄像头,用数字来控制不同的设备,例如,0
,1
。视频文件只需指定好路径即可。
import cv2 #opencv读取的格式是BGR
import matplotlib.pyplot as plt
import numpy as np
vc = cv2.VideoCapture('video.mp4')
# 检查是否打开正确
if vc.isOpened():
oepn, frame = vc.read()
else:
open = False
while open:
ret, frame = vc.read()
if frame is None:
break
if ret == True:
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
cv2.imshow('result', gray)
if cv2.waitKey(100) & 0xFF == 27:
break
vc.release()
cv2.destroyAllWindows()
4、使用cv2(OpenCV)截取部分图像数据
可以方便的对图片进行载取需要的数据,代码如下,
import cv2 #opencv读取的格式是BGR
import matplotlib.pyplot as plt
import numpy as np
img=cv2.imread('cjavapy.jpg')
print(img)
#图像的显示,也可以创建多个窗口
cv2.imshow('image',img)
part=img[0:50,0:200]
cv2.imshow("part",part)
# 等待时间,毫秒级,0表示任意键终止
cv2.waitKey(0)
cv2.destroyAllWindows()
5、使用cv2(OpenCV)提取颜色通道
可以使用cv2.split()
对颜色通道进行提取,代码如下,
import cv2 #opencv读取的格式是BGR
import matplotlib.pyplot as plt
import numpy as np
img=cv2.imread('cjavapy.jpg')
b,g,r=cv2.split(img)
print(r)
print(r.shape)
img=cv2.merge((b,g,r))
print(img.shape)
# 只保留R
cur_img = img.copy()
cur_img[:,:,0] = 0
cur_img[:,:,1] = 0
cv2.imshow("R",cur_img)
# 等待时间,毫秒级,0表示任意键终止
cv2.waitKey(0)
cv2.destroyAllWindows()
# 只保留G
cur_img = img.copy()
cur_img[:,:,0] = 0
cur_img[:,:,2] = 0
cv2.imshow("G",cur_img)
# 等待时间,毫秒级,0表示任意键终止
cv2.waitKey(0)
cv2.destroyAllWindows()
# 只保留B
cur_img = img.copy()
cur_img[:,:,1] = 0
cur_img[:,:,2] = 0
cv2.imshow("B",cur_img)
# 等待时间,毫秒级,0表示任意键终止
cv2.waitKey(0)
cv2.destroyAllWindows()
6、使用cv2(OpenCV)进行边界填充
BORDER_REPLICATE
:复制法,也就是复制最边缘像素。
BORDER_REFLECT
:反射法,对感兴趣的图像中的像素在两边进行复制例如:fedcba|abcdefgh|hgfedcb
BORDER_REFLECT_101
:反射法,也就是以最边缘像素为轴,对称,gfedcb|abcdefgh|gfedcba
BORDER_WRAP
:外包装法cdefgh|abcdefgh|abcdefg
BORDER_CONSTANT
:常量法,常数值填充。
import cv2 #opencv读取的格式是BGR
import matplotlib.pyplot as plt
import numpy as np
img=cv2.imread('cjavapy.jpg')
top_size,bottom_size,left_size,right_size = (50,50,50,50)
replicate = cv2.copyMakeBorder(img, top_size, bottom_size, left_size, right_size, borderType=cv2.BORDER_REPLICATE)
reflect = cv2.copyMakeBorder(img, top_size, bottom_size, left_size, right_size,cv2.BORDER_REFLECT)
reflect101 = cv2.copyMakeBorder(img, top_size, bottom_size, left_size, right_size, cv2.BORDER_REFLECT_101)
wrap = cv2.copyMakeBorder(img, top_size, bottom_size, left_size, right_size, cv2.BORDER_WRAP)
constant = cv2.copyMakeBorder(img, top_size, bottom_size, left_size, right_size,cv2.BORDER_CONSTANT, value=0)
import matplotlib.pyplot as plt
plt.subplot(231), plt.imshow(img, 'gray'), plt.title('ORIGINAL')
plt.subplot(232), plt.imshow(replicate, 'gray'), plt.title('REPLICATE')
plt.subplot(233), plt.imshow(reflect, 'gray'), plt.title('REFLECT')
plt.subplot(234), plt.imshow(reflect101, 'gray'), plt.title('REFLECT_101')
plt.subplot(235), plt.imshow(wrap, 'gray'), plt.title('WRAP')
plt.subplot(236), plt.imshow(constant, 'gray'), plt.title('CONSTANT')
plt.show()
7、图像数值计算
两个图像可以进行数值计算,代码如下,
import cv2 #opencv读取的格式是BGR
import matplotlib.pyplot as plt
import numpy as np
img_1=cv2.imread('img1.jpg')
img=img_1 + 10
print(img_1[:5,:,0])
print(img[:5,:,0])
#相当于% 256
print((img + img_1)[:5,:,0])
#取最大值
print(cv2.add(img,img_1)[:5,:,0])
8、图像融合
使用cv2.addWeighted()
可以进行两个图像进行融合,代码如下,
import cv2 #opencv读取的格式是BGR
import matplotlib.pyplot as plt
import numpy as np
img_1=cv2.imread('img1.jpg')
img_2=cv2.imread('img2.jpg')
print(img_1.shape) #img_1尺寸:(400, 500, 3)
#查看img_1的尺寸,并设置成相同尺寸才能融合
img_2 = cv2.resize(img_2, (500, 400))
res = cv2.addWeighted(img_1, 0.4, img_2, 0.6, 0)
plt.imshow(res)
了解更多分析及数据抓取可查看:
http://data.yisurvey.com:8989/
特别说明:本文旨在技术交流,请勿将涉及的技术用于非法用途,否则一切后果自负。如果您觉得我们侵犯了您的合法权益,请联系我们予以处理。
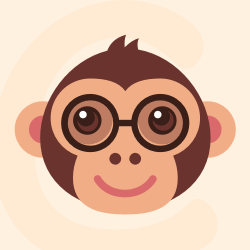



更多推荐
所有评论(0)