Compose的环境配置(一)

一、前言
现在是2021年5月14日,今天着手开始进行Compose的学习,本觉得出来也很久了。各方面教程比较完善了,但是还是遇到了很多问题,这里将过程总结一下。在实际学习中发现Compose第一个正式版本其实还没有出来,所以等到后面正式版本出来后,不知道是否还需要进行更改。另外Compose预览这个功能现在还是Canary版本,正式版本的Android Studio是没法使用的,所以写完后如果想看效果只能安装下程序了
二、依赖配置
由于现在Compose还没有正式版本,因此,在有新版本时候需要及时的更新到最新版本,否则可能会导致程序无法编译
-
开发语言:
kotlin
,因为Compose没有Java版本,所以只能使用kotlin进行开发。 -
最低支持版本:21
defaultConfig {
...
minSdkVersion 21
...
}
- kotlin最低版本:1.5.10
buildscript {
ext.kotlin_version = "1.5.10"
repositories {
google()
jcenter()
}
dependencies {
classpath 'com.android.tools.build:gradle:4.2.2'
classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version"
}
}
以上是基本的版本配置,但是我在使用的时候用的是最新版本,另外还有一些其他的配置,所以完整配置如下:
./rootProject/build.gradle
// Top-level build file where you can add configuration options common to all sub-projects/modules.
buildscript {
ext.kotlin_version = "1.5.10"
repositories {
google()
jcenter()
}
dependencies {
classpath 'com.android.tools.build:gradle:4.2.2'
classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version"
}
}
allprojects {
repositories {
google()
jcenter()
}
}
task clean(type: Delete) {
delete rootProject.buildDir
}
./rootProject/gradle/wrapper/gradle-wrapper.properties
distributionUrl=https\://services.gradle.org/distributions/gradle-6.7.1-bin.zip
./rootProject/app/build.gradle
plugins {
id 'com.android.application'
id 'kotlin-android'
}
android {
compileSdkVersion 30
buildToolsVersion "30.0.3"
defaultConfig {
applicationId "com.test.compose"
minSdkVersion 21
targetSdkVersion 30
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildFeatures {
// Enables Jetpack Compose for this module
compose true
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
kotlinOptions {
jvmTarget = '1.8'
}
composeOptions {
//这里面的版本是相互匹配的,而这个编译版本又跟dependencies中的依赖版本匹配
kotlinCompilerExtensionVersion '1.0.0-rc01'
//这个版本必须写,否则使用的kotlin版本可能不是这个版本。就会编译失败
kotlinCompilerVersion "1.5.10"
}
//具体参考这个链接进行比较版本,[这里](https://developer.android.com/jetpack/androidx/releases/compose-kotlin)
}
dependencies {
implementation "org.jetbrains.kotlin:kotlin-stdlib:$kotlin_version"
implementation 'androidx.core:core-ktx:1.3.2'
implementation 'androidx.appcompat:appcompat:1.2.0'
implementation 'com.google.android.material:material:1.3.0'
implementation 'androidx.constraintlayout:constraintlayout:2.0.4'
testImplementation 'junit:junit:4.13.2'
androidTestImplementation 'androidx.test.ext:junit:1.1.2'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.3.0'
// Tooling support (Previews, etc.)
implementation 'androidx.compose.ui:ui-tooling:1.0.0-rc01'
// Foundation (Border, Background, Box, Image, Scroll, shapes, animations, etc.)
implementation 'androidx.compose.foundation:foundation:1.0.0-rc01'
// Material Design
implementation 'androidx.compose.material:material:1.0.0-rc01'
// Material design icons
implementation 'androidx.compose.material:material-icons-core:1.0.0-rc01'
implementation 'androidx.compose.material:material-icons-extended:1.0.0-rc01'
// Integration with activities
implementation 'androidx.activity:activity-compose:1.3.0-rc01'
// Integration with ViewModels
implementation 'androidx.lifecycle:lifecycle-viewmodel-compose:1.0.0-rc01'
// Integration with observables
implementation 'androidx.compose.runtime:runtime-livedata:1.0.0-rc01'
implementation 'androidx.compose.runtime:runtime-rxjava2:1.0.0-rc01'
// UI Tests
androidTestImplementation 'androidx.compose.ui:ui-test-junit4:1.0.0-rc01'
}
三、示例代码
MainActivity.kt
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// setContentView(R.layout.activity_main)
setContent {
content()
}
}
@Preview
@Composable
fun content(){
Text(
text = "你好啊"
)
}
}
@Preview
@Composable
fun ContentTop(){
Text(
text = "你好啊"
)
}
需要注意的是,如果使用@Preview
进行预览的话,必须要有默认构造函数或者在顶层代码里面,否则会出错,错误提示如下:
Preview must be a top level declarations or in a top level class with a default constructor.
比如如下代码就有问题,没法预览
class BottomDialog(val string: String) : BottomSheetDialogFragment() {
@Preview(showBackground = true)
@Composable
fun GetTest(){
Text(text = "你好啊")
}
}
需要修改为以下方式:
class BottomDialog : BottomSheetDialogFragment {
constructor(): super(){
}
@JvmOverloads
constructor(string: String): this(){
}
@Preview(showBackground = true)
@Composable
fun GetTest(){
Text(text = "你好啊")
}
}
或者将预览的代码移动到类外面去
class BottomDialog : BottomSheetDialogFragment {
...
}
@Preview(showBackground = true)
@Composable
fun GetTest(){
Text(text = "你好啊")
}
四、总结
这里走了一些弯路,最大的问题就是官网上文档可能没跟得上更新,有些地方没办法使用。
1、各个依赖的版本问题,依赖版本需要和kotlin版本匹配,不匹配的话编译一直失败。
2、其次就是添加一个插件一直编译出错,后来发现不写也没事,
plugins {
id 'org.jetbrains.kotlin.android' version '1.5.10'
}
3、需要添加一句话,要不然依赖可能会使用不知道哪的版本,就会出现版本冲突
composeOptions {
...
//这个版本必须写,否则使用的kotlin版本可能不是这个版本。就会编译失败
kotlinCompilerVersion "1.5.10"
}
五、最新版本配置
当前时间为2024年1月12日
Android Studio版本:
Android Studio Hedgehog | 2023.1.1 Patch 1
Build #AI-231.9392.1.2311.11255304, built on December 27, 2023
Runtime version: 17.0.7+0-17.0.7b1000.6-10550314 aarch64
VM: OpenJDK 64-Bit Server VM by JetBrains s.r.o.
macOS 14.2.1
GC: G1 Young Generation, G1 Old Generation
Memory: 4096M
Cores: 8
Metal Rendering is ON
Registry:
external.system.auto.import.disabled=true
debugger.new.tool.window.layout=true
ide.text.editor.with.preview.show.floating.toolbar=false
ide.experimental.ui=true
ide.images.show.chessboard=true
Non-Bundled Plugins:
Gradle View (4.0.0)
com.github.gitofleonardo.simplesqlitebrowser (1.0.7)
idea.plugin.protoeditor (231.9225.5)
com.developerphil.adbidea (1.6.11)
项目根目录下面gradle配置:
buildscript {
ext {
compose_version = '1.5.4'
}
}// Top-level build file where you can add configuration options common to all sub-projects/modules.
plugins {
id 'com.android.application' version '8.2.1' apply false
id 'com.android.library' version '8.2.1' apply false
id 'org.jetbrains.kotlin.android' version '1.9.22' apply false
}
task clean(type: Delete) {
delete rootProject.buildDir
}
gradle-wrapper.properties配置
#Thu Jun 02 10:33:23 CST 2022
distributionBase=GRADLE_USER_HOME
distributionUrl=https\://services.gradle.org/distributions/gradle-8.2-bin.zip
distributionPath=wrapper/dists
zipStorePath=wrapper/dists
zipStoreBase=GRADLE_USER_HOME
app下吗gradle配置
plugins {
id 'com.android.application'
id 'org.jetbrains.kotlin.android'
}
android {
compileSdk 34
defaultConfig {
applicationId "com.design.compose"
minSdk 21
targetSdk 34
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
vectorDrawables {
useSupportLibrary true
}
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_18
targetCompatibility JavaVersion.VERSION_18
}
kotlinOptions {
jvmTarget = JavaVersion.VERSION_18
}
buildFeatures {
compose true
}
composeOptions {
kotlinCompilerExtensionVersion "1.5.8"
kotlinCompilerVersion "1.9.22"
}
packagingOptions {
resources {
excludes += '/META-INF/{AL2.0,LGPL2.1}'
}
}
namespace 'com.design.compose'
}
dependencies {
implementation 'androidx.core:core-ktx:1.12.0'
implementation "androidx.compose.ui:ui:$compose_version"
implementation "androidx.compose.material:material:$compose_version"
implementation "androidx.compose.material3:material3"
implementation "androidx.compose.ui:ui-tooling-preview:$compose_version"
implementation("androidx.activity:activity-compose:1.8.1")
implementation(platform("androidx.compose:compose-bom:2023.03.00"))
implementation("androidx.compose.ui:ui")
implementation("androidx.compose.ui:ui-graphics")
implementation("androidx.compose.ui:ui-tooling-preview")
implementation "androidx.paging:paging-compose:3.2.1"
implementation "androidx.paging:paging-compose-android:3.3.0-alpha02"
implementation "androidx.paging:paging-runtime-ktx:3.2.1"
implementation "androidx.paging:paging-common-ktx:3.2.1"
implementation "androidx.room:room-paging:2.6.1"
implementation 'androidx.lifecycle:lifecycle-runtime-ktx:2.6.2'
implementation 'androidx.activity:activity-compose:1.8.1'
testImplementation 'junit:junit:4.13.2'
androidTestImplementation 'androidx.test.ext:junit:1.1.5'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.5.1'
androidTestImplementation "androidx.compose.ui:ui-test-junit4:$compose_version"
debugImplementation "androidx.compose.ui:ui-tooling:$compose_version"
debugImplementation "androidx.compose.ui:ui-test-manifest:$compose_version"
}
六、参考链接:
- compose版本:https://developer.android.google.cn/jetpack/androidx/releases/compose?hl=en#versions
- Compose配置:https://developer.android.google.cn/jetpack/compose/setup
- Compose教程:https://developer.android.google.cn/courses/pathways/compose
- Google为Compose开发的拓展库https://google.github.io/accompanist/flowlayout/
- compose版本兼容性处理
- Jetpack Compose 博物馆,记录了所有的控件
- Compose学习-基础控件
- compose
- 沉浸式状态栏处理
- android Compose中沉浸式设计和导航栏的处理
- 三步走实现 Compose 沉浸式状态栏
- Compose 中的窗口边衬区
- dialog
- 源码中material3 的API
- compose 自身提供的组件UI示例
- material3的官网UI示例
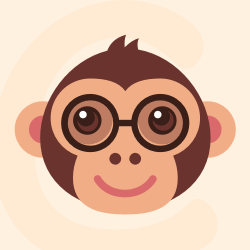



更多推荐
所有评论(0)