
monaco-editor基本使用以及monaco-editor封装成vue组件
vue
vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
项目地址:https://gitcode.com/gh_mirrors/vu/vue

·
一、monaco-editor基本使用
以vue2项目为例
安装依赖
npm i monaco-editor
npm i monaco-editor-webpack-plugin
配置vue.config.js
const MonacoWebpackPlugin = require('monaco-editor-webpack-plugin')
module.exports = {
configureWebpack: {
plugins: [
new MonacoWebpackPlugin()
]
}
}
使用monaco-editor前,需要先准备一个容器来挂载monaco-editor实例
<div id="monacoEditorContainer" style="height:300px;"></div>
创建monaco-editor实例
使用monaco.editor.create方法创建monaco-editor实例,create方法的第一个参数接收一个dom元素,第二个参数可选,接收一个IStandaloneEditorConstructionOptions配置对象
关于IStandaloneEditorConstructionOptions的信息可查阅monaco-editor api文档 https://microsoft.github.io/monaco-editor/docs.html#interfaces/editor.IStandaloneEditorConstructionOptions.html
例如:
<script>
import * as monaco from 'monaco-editor'
export default {
data() {
return {
standaloneEditorConstructionOptions: {
value: '', // 编辑器的值
language: 'javascript', //语言
theme: 'vs-dark', // 编辑器主题:vs, hc-black, or vs-dark
autoIndent: true, // 自动缩进
readOnly: false, // 是否只读
}
}
},
mounted() {
this.createMonacoEditor()
},
methods: {
createMonacoEditor() {
const container = document.getElementById('monacoEditorContainer')
this.monacoEditor = monaco.editor.create(container, this.standaloneEditorConstructionOptions)
}
}
}
</script>
完整代码
<template>
<div>
<div id="monacoEditorContainer" style="height:300px;"></div>
</div>
</template>
<script>
import * as monaco from 'monaco-editor'
export default {
data() {
return {
standaloneEditorConstructionOptions: {
value: '', // 编辑器的值
language: 'javascript', //语言
theme: 'vs-dark', // 编辑器主题:vs, hc-black, or vs-dark
autoIndent: true, // 自动缩进
readOnly: false, // 是否只读
}
}
},
mounted() {
this.createMonacoEditor()
},
methods: {
createMonacoEditor() {
const container = document.getElementById('monacoEditorContainer')
this.monacoEditor = monaco.editor.create(container, this.standaloneEditorConstructionOptions)
}
}
}
</script>
<style scoped lang="less">
</style>
二、monaco-editor封装成vue组件
my-monaco-editor.vue
<!--
my-monaco-editor:
基于monaco-editor封装的vue组件,支持尺寸、配置的响应式
-->
<template>
<div :style="{height, width}" class="my-monaco-editor"></div>
</template>
<script>
import * as monaco from 'monaco-editor'
export default {
props: {
options: {
type: Object,
default: () => {
}
},
height: {
type: String,
default: '300px'
},
width: {
type: String,
default: '100%'
},
code: {
type: String,
default: ''
}
},
data() {
return {
defaultOptions: {
value: this.code, // 编辑器的值
language: 'javascript', //语言
theme: 'vs-dark', // 编辑器主题:vs, hc-black, or vs-dark
autoIndent: true, // 自动缩进
readOnly: false, // 是否只读
}
}
},
computed: {
standaloneEditorConstructionOptions() {
return Object.assign(this.defaultOptions, this.options)
}
},
methods: {
createMonacoEditor() {
this.monacoEditor = monaco.editor.create(this.$el, this.standaloneEditorConstructionOptions)
this.monacoEditor.onDidChangeModelContent(event => {
this.$emit('update:code', this.monacoEditor.getValue())
})
},
reSize() {
this.$nextTick(() => {
this.monacoEditor.layout()
})
}
},
mounted() {
this.createMonacoEditor()
},
watch: {
height() {
this.reSize()
},
width() {
this.reSize()
},
options: {
handler() {
this.$nextTick(() => {
this.monacoEditor.updateOptions(this.standaloneEditorConstructionOptions)
})
},
deep: true
}
}
}
</script>
<style lang="less" scoped>
</style>
使用my-monaco-editor
<template>
<div class="monaco-editor-view">
<!--演示尺寸响应式-->
<el-form>
<el-form-item label="height">
<el-input v-model="inputHeight" @change="val => height = val"></el-input>
</el-form-item>
<el-form-item label="width">
<el-input v-model="inputWidth" @change="val => width = val"></el-input>
</el-form-item>
</el-form>
<my-monaco-editor :code.sync="code" :height="height" :options="options" :width="width"></my-monaco-editor>
</div>
</template>
<script>
import MyMonacoEditor from '@/components/my-monaco-editor'
export default {
components: {
MyMonacoEditor
},
data() {
return {
code: 'ok',
inputHeight: '',
inputWidth: '',
height: '100px',
width: '500px',
options: {}
}
},
created() {
this.inputHeight = this.height
this.inputWidth = this.width
setTimeout(() => {
//演示配置响应式
this.changeOptions()
}, 2000)
},
methods: {
changeOptions() {
this.$set(this.options, 'fontSize', 40)
}
}
}
</script>
<style lang="less" scoped>
</style>
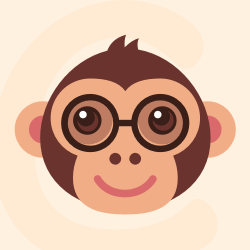



vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
最近提交(Master分支:6 个月前 )
9e887079
[skip ci] 4 个月前
73486cb5
* chore: fix link broken
Signed-off-by: snoppy <michaleli@foxmail.com>
* Update packages/template-compiler/README.md [skip ci]
---------
Signed-off-by: snoppy <michaleli@foxmail.com>
Co-authored-by: Eduardo San Martin Morote <posva@users.noreply.github.com> 8 个月前
更多推荐
所有评论(0)