cJSON源码解析
cJSON
Ultralightweight JSON parser in ANSI C
项目地址:https://gitcode.com/gh_mirrors/cj/cJSON

·
对于c语言来说是没有字典这样的结构的,所以对于解析json格式的数据来说不是那么好解析,但是有些时候又会需要处理这样的数据格式,这里就有一个解析json的函数库可以给我们使用了。
cJSON从名字可知,整个项目都是以极标准的C来写的,意思说,可以跨各种平台使用了。cJSON是一个超轻巧,携带方便,单文件,简单的可以作为ANSI-C标准的JSON解析器。
- cJSON.h
#ifndef cJSON__h
#define cJSON__h
#ifdef __cplusplus
extern "C"
//extern "C"的主要作用就是为了能够正确实现C++代码调用其他C语言代码。加上extern "C"后,
//会指示编译器这部分代码按C语言的进行编译,而不是C++的。由于C++支持函数重载,因此编译
//器编译函数的过程中会将函数的参数类型也加到编译后的代码中,而不仅仅是函数名;而C语言
//并不支持函数重载,因此编译C语言代码的函数时不会带上函数的参数类型
{
#endif
/* Json中item的类型*/
#define cJSON_False 0
#define cJSON_True 1
#define cJSON_NULL 2
#define cJSON_Number 3
#define cJSON_String 4
#define cJSON_Array 5
#define cJSON_Object 6
#define cJSON_IsReference 256
#define cJSON_StringIsConst 512
/* The cJSON structure: */
typedef struct cJSON {
struct cJSON* next, * prev; /*遍历数组或对象链的前向或后向链表指针*/
struct cJSON* child; /*数组或对象的孩子节点*/
int type; /*item的类型*/
char* valuestring; /*item的类型是cJSON_String,valuestring存储其值*/
int valueint; /*整数值*/
double valuedouble; /*浮点数值*/
char* string; /* key的名字*/
} cJSON;
typedef struct cJSON_Hooks {
void* (*malloc_fn)(size_t sz);
void (*free_fn)(void* ptr);
} cJSON_Hooks;
/*向cJSON提供malloc和free函数 */
extern void cJSON_InitHooks(cJSON_Hooks* hooks);
/*提供一个JSON块,然后返回一个可以查询的cJSON对象。完成后调用cJSON_Delete。*/
extern cJSON* cJSON_Parse(const char* value);
/* Render a cJSON entity to text for transfer/storage. Free the char* when finished. */
extern char* cJSON_Print(cJSON* item);
/* Render a cJSON entity to text for transfer/storage without any formatting. Free the char* when finished. */
extern char* cJSON_PrintUnformatted(cJSON* item);
/* Render a cJSON entity to text using a buffered strategy. prebuffer is a guess at the final size. guessing well reduces reallocation. fmt=0 gives unformatted, =1 gives formatted */
extern char* cJSON_PrintBuffered(cJSON* item, int prebuffer, int fmt);
/* Delete a cJSON entity and all subentities. */
extern void cJSON_Delete(cJSON* c);
/* Returns the number of items in an array (or object). */
extern int cJSON_GetArraySize(cJSON* array);
/* Retrieve item number "item" from array "array". Returns NULL if unsuccessful. */
extern cJSON* cJSON_GetArrayItem(cJSON* array, int item);
/* Get item "string" from object. Case insensitive. */
extern cJSON* cJSON_GetObjectItem(cJSON* object, const char* string);
/* For analysing failed parses. This returns a pointer to the parse error. You'll probably need to look a few chars back to make sense of it. Defined when cJSON_Parse() returns 0. 0 when cJSON_Parse() succeeds. */
extern const char* cJSON_GetErrorPtr(void);
/* These calls create a cJSON item of the appropriate type. */
extern cJSON* cJSON_CreateNull(void);
extern cJSON* cJSON_CreateTrue(void);
extern cJSON* cJSON_CreateFalse(void);
extern cJSON* cJSON_CreateBool(int b);
extern cJSON* cJSON_CreateNumber(double num);
extern cJSON* cJSON_CreateString(const char* string);
extern cJSON* cJSON_CreateArray(void);
extern cJSON* cJSON_CreateObject(void);
/* These utilities create an Array of count items. */
extern cJSON* cJSON_CreateIntArray(const int* numbers, int count);
extern cJSON* cJSON_CreateFloatArray(const float* numbers, int count);
extern cJSON* cJSON_CreateDoubleArray(const double* numbers, int count);
extern cJSON* cJSON_CreateStringArray(const char** strings, int count);
/* Append item to the specified array/object. */
extern void cJSON_AddItemToArray(cJSON* array, cJSON* item);
extern void cJSON_AddItemToObject(cJSON* object, const char* string, cJSON* item);
extern void cJSON_AddItemToObjectCS(cJSON* object, const char* string, cJSON* item); /* Use this when string is definitely const (i.e. a literal, or as good as), and will definitely survive the cJSON object */
/* Append reference to item to the specified array/object. Use this when you want to add an existing cJSON to a new cJSON, but don't want to corrupt your existing cJSON. */
extern void cJSON_AddItemReferenceToArray(cJSON* array, cJSON* item);
extern void cJSON_AddItemReferenceToObject(cJSON* object, const char* string, cJSON* item);
/* Remove/Detatch items from Arrays/Objects. */
extern cJSON* cJSON_DetachItemFromArray(cJSON* array, int which);
extern void cJSON_DeleteItemFromArray(cJSON* array, int which);
extern cJSON* cJSON_DetachItemFromObject(cJSON* object, const char* string);
extern void cJSON_DeleteItemFromObject(cJSON* object, const char* string);
/* Update array items. */
extern void cJSON_InsertItemInArray(cJSON* array, int which, cJSON* newitem); /* Shifts pre-existing items to the right. */
extern void cJSON_ReplaceItemInArray(cJSON* array, int which, cJSON* newitem);
extern void cJSON_ReplaceItemInObject(cJSON* object, const char* string, cJSON* newitem);
/* Duplicate a cJSON item */
extern cJSON* cJSON_Duplicate(cJSON* item, int recurse);
/* Duplicate will create a new, identical cJSON item to the one you pass, in new memory that will
need to be released. With recurse!=0, it will duplicate any children connected to the item.
The item->next and ->prev pointers are always zero on return from Duplicate. */
/* ParseWithOpts allows you to require (and check) that the JSON is null terminated, and to retrieve the pointer to the final byte parsed. */
extern cJSON* cJSON_ParseWithOpts(const char* value, const char** return_parse_end, int require_null_terminated);
extern void cJSON_Minify(char* json);
/* Macros for creating things quickly. */
#define cJSON_AddNullToObject(object,name) cJSON_AddItemToObject(object, name, cJSON_CreateNull())
#define cJSON_AddTrueToObject(object,name) cJSON_AddItemToObject(object, name, cJSON_CreateTrue())
#define cJSON_AddFalseToObject(object,name) cJSON_AddItemToObject(object, name, cJSON_CreateFalse())
#define cJSON_AddBoolToObject(object,name,b) cJSON_AddItemToObject(object, name, cJSON_CreateBool(b))
#define cJSON_AddNumberToObject(object,name,n) cJSON_AddItemToObject(object, name, cJSON_CreateNumber(n))
#define cJSON_AddStringToObject(object,name,s) cJSON_AddItemToObject(object, name, cJSON_CreateString(s))
/* When assigning an integer value, it needs to be propagated to valuedouble too. */
#define cJSON_SetIntValue(object,val) ((object)?(object)->valueint=(object)->valuedouble=(val):(val))
#define cJSON_SetNumberValue(object,val) ((object)?(object)->valueint=(object)->valuedouble=(val):(val))
#ifdef __cplusplus
}
#endif
#endif
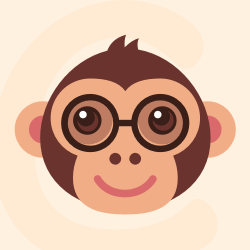



Ultralightweight JSON parser in ANSI C
最近提交(Master分支:2 个月前 )
424ce4ce
This reverts commit 5b502cdbfb21fbe5f6cf9ffbd2b96e4281a741e6.
Related to #860
4 个月前
32497300 - 5 个月前
更多推荐
所有评论(0)