Cjson简介与使用
cJSON
Ultralightweight JSON parser in ANSI C
项目地址:https://gitcode.com/gh_mirrors/cj/cJSON

·
json格式
- json元素类型
/* cJSON Types: */
#define cJSON_Invalid (0)
#define cJSON_False (1 << 0)
#define cJSON_True (1 << 1)
#define cJSON_NULL (1 << 2)
#define cJSON_Number (1 << 3)
#define cJSON_String (1 << 4)
#define cJSON_Array (1 << 5)
#define cJSON_Object (1 << 6)
#define cJSON_Raw (1 << 7) /* raw json */
- json数组
- 中括号[整形,字符串,布尔类型,json数组,josn对象]
- [123,123.4,true, false, [12,34,56,“hello”,“world”]]
- 细节:最后一个元素是没有逗号的
- json 对象
- { }中是一些键值对
- {“name”:“ZhangSan”,
“name”:“li4”} - key值:必须是字符串,且不重复
- value值:可以使json对象,json数组,布尔,整形,字符串
- {“name”:“ZhangSan”,
- { }中是一些键值对
- json数组对象
- json数组+json对象
- {“name”:“ZhangSan”,
“name”:“li4”,
“张三”:{
“别名”:“老王”,
“性别”:“男”,
“孩子”:"[“小红”,“小黑”,“小白”]"
}
}
- {“name”:“ZhangSan”,
Cjson
- 下载cjson-master.zip包,解压命令 unzipt CJSON-master.zip
- cJson对象
typedef struct cJSON
{
/* next/prev allow you to walk array/object chains. Alternatively, use GetArraySize/GetArrayItem/GetObjectItem */
struct cJSON *next;
struct cJSON *prev;
/* An array or object item will have a child pointer pointing to a chain of the items in the array/object. */
struct cJSON *child;
/* The type of the item, as above. */
int type;
/* The item's string, if type==cJSON_String and type == cJSON_Raw */
char *valuestring;
/* writing to valueint is DEPRECATED, use cJSON_SetNumberValue instead */
int valueint;
/* The item's number, if type==cJSON_Number */
double valuedouble;
/* The item's name string, if this item is the child of, or is in the list of subitems of an object. */
char *string;
} cJSON;
- 创建一个json对象
CJSON_PUBLIC(cJSON *) cJSON_CreateObject(void)
{
cJSON *item = cJSON_New_Item(&global_hooks);
if (item) {
item->type = cJSON_Object;
}
return item;
}
- 往json对象中添加数据成员
CJSON_PUBLIC(void) cJSON_AddItemToObject(
cJSON *object, //json对象,即上面创建的json对象
const char *string, //key
cJSON *item //value(int,string, array, obj)
);
- 创建一个整形值
cJSON *cJSON_CreateNumber(double num);
- 创建一个字符串
cJSON *cJSON_CreateString(const char *string);
写入json数据
{
//json数据
"奔驰":{
"factory":"北京奔驰",
"last":31,
"price":83,
"sell":49,
"other":[123,
true,
"hello",
{"梅赛德斯奔驰":"The best or nothing"}
]
}
}
int main()
{
//创建对象
cJSON *obj = cJSON_CreateObject();
//创建以子对象
cJSON *subobj=cJson_CreateObject();
cJSON_AddItemToObject(subobj,"factory",cJSON_CreateString("北京奔驰"));
cJSON_AddItemToObject(subobj,"last",cJSON_CreateNumber(31));
cJSON_AddItemToObject(subobj,"price",cJSON_CreateNUmer(83));
cJSON_AddItemToObject(subobj,"sell",cJSON_CreateNUmer(49));
//创建一个空数组
cJSON *array = cJSON_CreateArray();
//数组中添加元素
cJSON_AddItemToArray(array,cJSON_CreateNumber(123));
cJSON_AddItemToArray(array,cJSON_CreateBool(1));
cJSON_AddItemToArray(array,cJSON_CreateString("hello"));
//数组中的对象
cJSON *subsub = cJSON_CreateObject();
cJSON_AddItemToObject(subsub,"梅赛德斯奔驰",cJSON_CreateString("The best or Nothing"));
cJSON_AddItemToArray(array, subsub);
cJSON_AddItemToObject(subobj,"other",array);
//obj中添加键值对
cJson_addItemtoObj("obj","奔驰",subobj);
//数据格式化
char *data = cJSON_Print(obj);
FILE* fp = fopen("car.json","w");
fwrite(data, sizeof(char), strlen(data)+1, fp);
fclose(fp);
return 0;
}
- 错误:在函数‘print_number’中:cJSON.c:对‘floor’未定义的引用
将函数对应的库加入 编译时增加 -lm ,math库
读json数据
- 将字符串解析为json结构
//返回值需要使用cJSON_Delete释放
(cJSON *) cJSON_Parse(
const char *value;
);
- 根据json key查找
(cJSON *) cJSON_GetObjectItem(
const cJSON * const object, //当前json对象
const char * const string //key值
);
typedef struct cJSON
{
struct cJSON *next;
struct cJSON *prev;
struct cJSON *child;
//重点在下面4行===========================
int type; //The type of the item, as above.指向对顶端的类型
char *valuestring; //从这里取 字符串
int valueint; //从这里取 整形值
double valuedouble; //从这里取 浮点值
//=======================================
char *string;
} cJSON;
- 获取json数组中的元素的个数
int cJSON_GetArraySize(cJSON *array);
- 获取数组下标找到对应的数组元素
cJSON *cJSON_GetArrayItem(cJSON *array,int index);
- 判断是否有可以值对应的键值对
//如果有这样一个key, 如果有这样一个key值 ,就会有一个value值
cJSON *cJSON_HasObjectItem(cJSON *object,const char* string);
- 判断数据
json = cJSON_Parse(topic_info->payload);
json_id = cJSON_GetObjectItem(json,id);
if(json_id.type == cJSON_String) //判断id键值的type是否是cJSON_String类型
{
HAL_Printf("id:%s\r\n", json_id->valuestring);//获取valuestring并打印
}
json_params = cJSON_GetObjectItem(json, "params");
if(json_params)
{
json_led = cJSON_GetObjectItem(json_params, "led");
if(json_led->type == cJSON_Number) //判断led键值的type是否是cJSON_Number类型
{
HAL_Printf("LED:%d\r\n", json_led->valueint); //获取valueint并打印
}
}
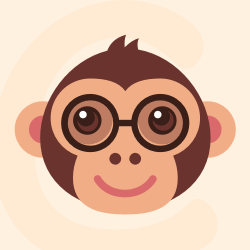



Ultralightweight JSON parser in ANSI C
最近提交(Master分支:2 个月前 )
424ce4ce
This reverts commit 5b502cdbfb21fbe5f6cf9ffbd2b96e4281a741e6.
Related to #860
4 个月前
32497300 - 5 个月前
更多推荐
所有评论(0)