使用cJSON库解析JSON字符串
cJSON
Ultralightweight JSON parser in ANSI C
项目地址:https://gitcode.com/gh_mirrors/cj/cJSON

·
一、四个常用的cJSON函数:
1 cJSON *cJSON_Parse(const char *value);
作用:将一个JSON
数据包,按照cJSON
结构体的结构序列化整个数据包,并在堆中开辟一块内存存储cJSON
结构体
返回值:成功返回一个指向内存块中的cJSON
的指针,失败返回NULL
2 cJSON *cJSON_GetObjectItem(cJSON *object,const char *string);
作用:获取JSON
字符串字段值
返回值:成功返回一个指向cJSON
类型的结构体指针,失败返回NULL
3 char *cJSON_Print(cJSON *item);
作用:将cJSON
数据解析成JSON
字符串,并在堆中开辟一块char*
的内存空间存储JSON
字符串
返回值:成功返回一个char*
指针该指针指向位于堆中JSON
字符串,失败返回NULL
4 void cJSON_Delete(cJSON *c);
作用:释放位于堆中cJSON
结构体内存
返回值:无
二、使用cJSON库解析JSON字符串
1.解析JSON格式1------解析简单的JSON字符串
/*解析JSON格式 */
#include <stdio.h>
#include "cJSON.h"
#include "string.h"
char jsonfomat[]="{\"firstName\":\"Brett\",\"value\": 23}";
int main()
{
cJSON *root=cJSON_Parse(jsonfomat);
cJSON *item=cJSON_GetObjectItem(root,"firstName");
printf("%s\n",(char *)item->valuestring);
item=cJSON_GetObjectItem(root,"value");
printf("%d\n",item->valueint);
cJSON_Delete(root);
}
下面按解析过程来描述一次:
(1) 首先调用cJSON_Parse()函数,解析JSON
数据包,并按照cJSON
结构体的结构序列化整个数据包。使用该函数会通过malloc()
函数在内存中开辟一个空间,使用完成需要手动释放。
cJSON*root=cJSON_Parse(json_string);
(2)调用cJSON_GetObjectItem()
函数,可从cJSON
结构体中查找某个子节点名称(键名称),如果查找成功可把该子节点序列化到cJSON
结构体中。
cJSON*item=cJSON_GetObjectItem(root,"firstName");
(3) 如果需要使用cJSON
结构体中的内容,可通过cJSON
结构体中的valueint
和valuestring
取出有价值的内容(即键的值)
本例子中,我们直接访问 item->valuestring
就获取到 "Brett"
的内容了。
(4) 通过cJSON_Delete()
,释放cJSON_Parse()
分配出来的内存空间。
cJSON_Delete(root);
2.解析JSON格式2-----解析嵌套对象的JSON格式
#include <stdio.h>
#include "cJSON.h"
#include "string.h"
typedef struct
{
char firstName[32];
char lastName[32];
char email[64];
int age;
float height;
} PERSON;
/*解析JSON格式
{
"person":
{
"firstName":"z",
"lastName":"shu",
"email":"www.baidu.com",
"age":8,
"height\":1.17
}
} */
char json_string[]= "{\"person\": { \"firstName\":\"z\", \"lastName\":\"shu\", \"email\":\"www.baidu.com\", \"age\":8, \"height\":1.17 } }";
int main()
{
cJSON* item;
PERSON person;
memset(&person,0,sizeof(person));
//调用cJSON_Parse()函数,解析JSON数据包
cJSON* root=cJSON_Parse(json_string);
//调用一次cJSON_GetObjectItem()函数,获取到对象person
cJSON *object=cJSON_GetObjectItem(root,"person");
//多次调用cJSON_GetObjectItem()函数,来获取对象的成员
item=cJSON_GetObjectItem(object,"firstName");
printf("firstName1=%s\n",(char *)item->valuestring);
memcpy(person.firstName,item->valuestring,strlen((char *)item->valuestring));
printf("firstName2=%s\n",(char *)person.firstName);
item=cJSON_GetObjectItem(object,"lastName");
memcpy(person.lastName,item->valuestring,strlen(item->valuestring));
printf("lastName=%s\n",person.lastName);
item=cJSON_GetObjectItem(object,"email");
memcpy(person.email,item->valuestring,strlen(item->valuestring));
printf("email=%s\n",person.email);
item=cJSON_GetObjectItem(object,"age");
person.age=item->valueint;
printf("age=%d\n",person.age);
item=cJSON_GetObjectItem(object,"height");
person.height=(float)item->valuedouble;
printf("height=%.2f\n",person.height);
//通过cJSON_Delete(),释放cJSON_Parse()分配出来的内存空间
cJSON_Delete(root);
return 0;
}
3.解析JSON格式3------解析嵌套数组的JSON字符串
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include "cJSON.h"
/*
{
"people":[
{"firstName":"z","lastName":"Jason","email":"bbbb@126.com","height":1.67},
{"lastName":"jadena","email":"jadena@126.com","age":8,"height":1.17},
{"email":"cccc@126.com","firstName":"z","lastName":"Juliet","age":36,"height":1.55}
]
}
*/
char json_string[]=
"{ \
\"people\":[ \
{\"firstName\":\"z\",\"lastName\":\"Jason\",\"email\":\"bbbb@126.com\",\"height\":1.67}, \
{\"lastName\":\"jadena\",\"email\":\"jadena@126.com\",\"age\":8,\"height\":1.17}, \
{\"email\":\"cccc@126.com\",\"firstName\":\"z\",\"lastName\":\"Juliet\",\"age\":36,\"height\":1.55} \
] \
}";
typedef struct
{
int id;
char firstName[32];
char lastName[32];
char email[64];
int age;
float height;
}people;
int main()
{
people worker[3]={{0}};
cJSON_to_struct_array(json_string, worker);
return 0;
}
//parse a key-value pair
int cJSON_to_str(char *json_string, char *str_val)
{
cJSON *root=cJSON_Parse(json_string);
if (!root)
{
printf("Error before: [%s]\n",cJSON_GetErrorPtr());
return -1;
}
else
{
cJSON *item=cJSON_GetObjectItem(root,"firstName");
if(item!=NULL)
{
printf("cJSON_GetObjectItem: type=%d, key is %s, value is %s\n",item->type,item->string,item->valuestring);
memcpy(str_val,item->valuestring,strlen(item->valuestring));
}
cJSON_Delete(root);
}
return 0;
}
//parse a object to struct
int cJSON_to_struct(char *json_string, people *person)
{
cJSON *item;
cJSON *root=cJSON_Parse(json_string);
if (!root)
{
printf("Error before: [%s]\n",cJSON_GetErrorPtr());
return -1;
}
else
{
cJSON *object=cJSON_GetObjectItem(root,"person");
if(object==NULL)
{
printf("Error before: [%s]\n",cJSON_GetErrorPtr());
cJSON_Delete(root);
return -1;
}
printf("cJSON_GetObjectItem: type=%d, key is %s, value is %s\n",object->type,object->string,object->valuestring);
if(object!=NULL)
{
item=cJSON_GetObjectItem(object,"firstName");
if(item!=NULL)
{
printf("cJSON_GetObjectItem: type=%d, string is %s, valuestring=%s\n",item->type,item->string,item->valuestring);
memcpy(person->firstName,item->valuestring,strlen(item->valuestring));
}
item=cJSON_GetObjectItem(object,"lastName");
if(item!=NULL)
{
printf("cJSON_GetObjectItem: type=%d, string is %s, valuestring=%s\n",item->type,item->string,item->valuestring);
memcpy(person->lastName,item->valuestring,strlen(item->valuestring));
}
item=cJSON_GetObjectItem(object,"email");
if(item!=NULL)
{
printf("cJSON_GetObjectItem: type=%d, string is %s, valuestring=%s\n",item->type,item->string,item->valuestring);
memcpy(person->email,item->valuestring,strlen(item->valuestring));
}
item=cJSON_GetObjectItem(object,"age");
if(item!=NULL)
{
printf("cJSON_GetObjectItem: type=%d, string is %s, valueint=%d\n",item->type,item->string,item->valueint);
person->age=item->valueint;
}
else
{
printf("cJSON_GetObjectItem: get age failed\n");
}
item=cJSON_GetObjectItem(object,"height");
if(item!=NULL)
{
printf("cJSON_GetObjectItem: type=%d, string is %s, valuedouble=%f\n",item->type,item->string,item->valuedouble);
person->height=item->valuedouble;
}
}
cJSON_Delete(root);
}
return 0;
}
//parse a struct array
int cJSON_to_struct_array(char *text, people worker[])
{
cJSON *json,*arrayItem,*item,*object;
int i;
//解析JSON数据包
json=cJSON_Parse(text);
if (!json)
{
printf("Error before: [%s]\n",cJSON_GetErrorPtr());
}
else
{
//获取到数组people
arrayItem=cJSON_GetObjectItem(json,"people");
//取出数组people,调用cJSON_GetArraySize()函数,来获取数组中对象的个数。然后,
//多次调用cJSON_GetArrayItem()函数,逐个读取数组中对象的内容。
if(arrayItem!=NULL)
{
//获取数组长度
int size=cJSON_GetArraySize(arrayItem);
printf("cJSON_GetArraySize: size=%d\n",size);
for(i=0;i<size;i++)
{
printf("第%d组\r\n",i);
//取数组成员
//取出array[i]的数据
object=cJSON_GetArrayItem(arrayItem,i);
item=cJSON_GetObjectItem(object,"firstName");
if(item!=NULL)
{
printf("cJSON_GetObjectItem: type=%d, string is %s\n",item->type,item->string);
memcpy(worker[i].firstName,item->valuestring,strlen(item->valuestring));
}
item=cJSON_GetObjectItem(object,"lastName");
if(item!=NULL)
{
printf("cJSON_GetObjectItem: type=%d, string is %s, valuestring=%s\n",item->type,item->string,item->valuestring);
memcpy(worker[i].lastName,item->valuestring,strlen(item->valuestring));
}
item=cJSON_GetObjectItem(object,"email");
if(item!=NULL)
{
printf("cJSON_GetObjectItem: type=%d, string is %s, valuestring=%s\n",item->type,item->string,item->valuestring);
memcpy(worker[i].email,item->valuestring,strlen(item->valuestring));
}
item=cJSON_GetObjectItem(object,"age");
if(item!=NULL)
{
printf("cJSON_GetObjectItem: type=%d, string is %s, valueint=%d\n",item->type,item->string,item->valueint);
worker[i].age=item->valueint;
}
else
{
printf("cJSON_GetObjectItem: get age failed\n");
}
item=cJSON_GetObjectItem(object,"height");
if(item!=NULL)
{
printf("cJSON_GetObjectItem: type=%d, string is %s, value=%f\n",item->type,item->string,item->valuedouble);
worker[i].height=item->valuedouble;
}
}
}
for(i=0;i<3;i++)
{
printf("i=%d, firstName=%s,lastName=%s,email=%s,age=%d,height=%f\n",
i,
worker[i].firstName,
worker[i].lastName,
worker[i].email,
worker[i].age,
worker[i].height);
}
cJSON_Delete(json);
}
return 0;
}
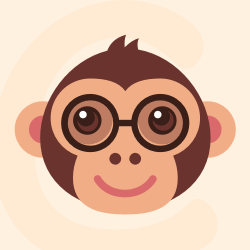



Ultralightweight JSON parser in ANSI C
最近提交(Master分支:2 个月前 )
424ce4ce
This reverts commit 5b502cdbfb21fbe5f6cf9ffbd2b96e4281a741e6.
Related to #860
4 个月前
32497300 - 5 个月前
更多推荐
所有评论(0)