cJSON的结构与常用函数说明

cJSON结构:
typedef struct cJSON {
struct cJSON *next,*prev; /* next/prev allow you to walk array/object chains. Alternatively, use GetArraySize/GetArrayItem/GetObjectItem */
struct cJSON *child; /* An array or object item will have a child pointer pointing to a chain of the items in the array/object. */
int type; /* The type of the item, as above. */
char *valuestring; /* The item's string, if type==cJSON_String */
int valueint; /* The item's number, if type==cJSON_Number */
double valuedouble; /* The item's number, if type==cJSON_Number */
char *string; /* The item's name string, if this item is the child of, or is in the list of subitems of an object. */
} cJSON;
/* Supply a block ofJSON, and this returns a cJSON object you can interrogate. Call cJSON_Deletewhen finished. */
extern cJSON *cJSON_Parse(const char *value);//从 给定的json字符串中得到cjson对象
/* Render a cJSONentity to text for transfer/storage. Free the char* when finished. */
extern char *cJSON_Print(cJSON *item);//从cjson对象中获取有格式的json对象
/* Render a cJSONentity to text for transfer/storage without any formatting. Free the char* whenfinished. */
extern char *cJSON_PrintUnformatted(cJSON *item);//从cjson对象中获取无格式的json对象
/* Delete a cJSONentity and all subentities. */
extern void cJSON_Delete(cJSON *c);//删除cjson对象,释放链表占用的内存空间
/* Returns thenumber of items in an array (or object). */
extern int cJSON_GetArraySize(cJSON *array);//获取cjson对象数组成员的个数
/* Retrieve itemnumber "item" from array "array". Returns NULL ifunsuccessful. */
extern cJSON *cJSON_GetArrayItem(cJSON*array,int item);//根据下标获取cjosn对象数组中的对象
/* Get item"string" from object. Case insensitive. */
extern cJSON *cJSON_GetObjectItem(cJSON*object,const char *string);//根据键获取对应的值(cjson对象)
/* For analysingfailed parses. This returns a pointer to the parse error. You'll probably needto look a few chars back to make sense of it. Defined when cJSON_Parse()returns 0. 0 when cJSON_Parse() succeeds. */
extern const char *cJSON_GetErrorPtr(void);//获取错误字符串
示例:
//解析json字符串
#include<stdlib.h>
#include<stdio.h>
#include <string.h>
#include <iostream>
#include "cJSON.h"
int main()
{
char* json = "{\"id\":\"1200000000\",\"number\":1}";
cJSON * root_base = cJSON_Parse(json);//change to json object
if(root_base )
{ printf("root_base is not null!\n ");
cJSON * cParse1 = cJSON_GetObjectItem(root_base,"id");
cJSON * cParse2 = cJSON_GetObjectItem(root_base,"number");
//get the value
std::string id = cParse->valuestring;
std::string number= cParse->valueint;
printf("id : %s\n",id.c_str());
printf("number: %d\n",number);
cJSON_Delete(root_base );//用完需要释放
}
return 0;
}
组json的常用函数:
cJSON * root = cJSON_CreateObject(); //创建JSON结构体
cJSON_AddNumberToObject(root, "number", 0); //添加键值对
cJSON_AddStringToObject(root, "name", "i") ; //添加键值对
cJSON * root_arry = cJSON_CreateArray();//创建json数组型结构体
cJSON_AddItemToArray(root_arry,root); //为数组添加对象
cJSON_AddItemToObject(root_base, "array", root_array);//为对象添加数组
需要使用cjson时 可在工程中直接将以下文件作为源文件使用,官网可下载,应该也有相应的生成库,以下是我上传的文件链接:
文件: 点击打开链接
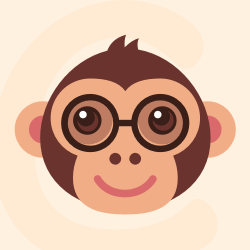



更多推荐
所有评论(0)