mqtt发送cjson数据并解析
cJSON
Ultralightweight JSON parser in ANSI C
项目地址:https://gitcode.com/gh_mirrors/cj/cJSON

·
本文实现mqtt发送端发送cjson数据,接受端接收数据并使用cjson解析。
主要功能是发送端发送位姿,接收端接收位姿话题并解析使用
1.发送端程序
CMakeLists.txt
cmake_minimum_required(VERSION 2.8)
project(mqttdemopub)
add_executable(${PROJECT_NAME} main.cpp cJSON.c cJSON.h)
target_link_libraries(${PROJECT_NAME} mosquitto)
main.cpp
#include <stdio.h>
#include <stdlib.h>
#include <mosquitto.h>
#include <string.h>
#include "cJSON.h"
#include <unistd.h>
#define HOST "localhost"
#define PORT 1883
#define KEEP_ALIVE 60
#define MSG_MAX_SIZE 512
#define TOPIC_NUM 3
bool session = true;
int main()
{
struct mosquitto *mosq = NULL;
//libmosquitto 库初始化
mosquitto_lib_init();
//创建mosquitto客户端
mosq = mosquitto_new(NULL,session,NULL);
if(!mosq){
printf("create client failed..\n");
mosquitto_lib_cleanup();
return 1;
}
//连接服务器
if(mosquitto_connect(mosq, HOST, PORT, KEEP_ALIVE)){
fprintf(stderr, "Unable to connect.\n");
return 1;
}
//开启一个线程,在线程里不停的调用 mosquitto_loop() 来处理网络信息
int loop = mosquitto_loop_start(mosq);
if(loop != MOSQ_ERR_SUCCESS)
{
printf("mosquitto loop error\n");
return 1;
}
while(1)
{
/*发布消息*/
//1.fill cjson struct
cJSON *pose;
pose=cJSON_CreateObject();
//pose x=1,y=2,yaw=30
cJSON_AddNumberToObject(pose,"x",1.0);
cJSON_AddNumberToObject(pose,"y",2.0);
cJSON_AddNumberToObject(pose,"yaw",30.0);
//2.cjson to char*
char* buff = cJSON_Print(pose);
if(NULL == buff)
{
continue;
}
mosquitto_publish(mosq,NULL,"topic_pose ",strlen(buff)+1,buff,0,0);
printf("%s\n",buff);
cJSON_Delete(pose);
free(buff);
sleep(1);
}
mosquitto_destroy(mosq);
mosquitto_lib_cleanup();
return 0;
}
2.接收端程序
cmake_minimum_required(VERSION 2.8)
project(mqttdemosub)
add_executable(${PROJECT_NAME} main.cpp cJSON.c cJSON.h)
target_link_libraries(${PROJECT_NAME} mosquitto)
main.cpp
#include <stdio.h>
#include <stdlib.h>
#include <mosquitto.h>
#include <string.h>
#include "cJSON.h"
#include <unistd.h>
#define HOST "localhost"
#define PORT 1883
#define KEEP_ALIVE 60
#define MSG_MAX_SIZE 512
#define TOPIC_NUM 3
bool session = true;
void my_message_callback(struct mosquitto *mosq, void *userdata, const struct mosquitto_message *message)
{
///message->topic is topic name
///(char *)message->payload is the data
if(message->payloadlen){
//printf("%s %s", message->topic, (char *)message->payload);
//parse json here
char * data=(char *)message->payload;
cJSON* pose=cJSON_Parse(data);
cJSON *item_x = cJSON_GetObjectItem(pose,"x"); //获取这个对象成员
double pose_x=item_x->valuedouble;
printf("pose_x :%lf\n",pose_x);
cJSON *item_y = cJSON_GetObjectItem(pose,"y"); //获取这个对象成员
double pose_y=item_y->valuedouble;
printf("pose_y :%lf\n",pose_y);
cJSON *item_yaw = cJSON_GetObjectItem(pose,"yaw"); //获取这个对象成员
double pose_yaw=item_yaw->valuedouble;
printf("pose_yaw :%lf\n",pose_yaw);
}else{
printf("%s (null)\n", message->topic);
}
fflush(stdout);
}
void my_connect_callback(struct mosquitto *mosq, void *userdata, int result)
{
int i;
if(!result){
/* Subscribe to broker information topics on successful connect. */
mosquitto_subscribe(mosq, NULL, "topic_pose ", 2);
}else{
fprintf(stderr, "Connect failed\n");
}
}
void my_subscribe_callback(struct mosquitto *mosq, void *userdata, int mid, int qos_count, const int *granted_qos)
{
int i;
printf("Subscribed (mid: %d): %d", mid, granted_qos[0]);
for(i=1; i<qos_count; i++){
printf(", %d", granted_qos[i]);
}
printf("\n");
}
void my_log_callback(struct mosquitto *mosq, void *userdata, int level, const char *str)
{
/* Pring all log messages regardless of level. */
printf("%s\n", str);
}
int main()
{
struct mosquitto *mosq = NULL;
//libmosquitto 库初始化
mosquitto_lib_init();
//创建mosquitto客户端
mosq = mosquitto_new(NULL,session,NULL);
if(!mosq){
printf("create client failed..\n");
mosquitto_lib_cleanup();
return 1;
}
//设置回调函数,需要时可使用
///1.show log:Client mosq-UunBize2TyoN0P2ubI received
///PUBLISH (d0, q0, r0, m0, 'topic_pose ', ... (33 bytes))
//mosquitto_log_callback_set(mosq, my_log_callback);
///2.set sub topic name
mosquitto_connect_callback_set(mosq, my_connect_callback);
///3.get topic name and data
mosquitto_message_callback_set(mosq, my_message_callback);
//mosquitto_subscribe_callback_set(mosq, my_subscribe_callback);
//连接服务器
if(mosquitto_connect(mosq, HOST, PORT, KEEP_ALIVE)){
fprintf(stderr, "Unable to connect.\n");
return 1;
}
//开启一个线程,在线程里不停的调用 mosquitto_loop() 来处理网络信息
int loop = mosquitto_loop_start(mosq);
if(loop != MOSQ_ERR_SUCCESS)
{
printf("mosquitto loop error\n");
return 1;
}
while(1)
{
sleep(1);
}
mosquitto_destroy(mosq);
mosquitto_lib_cleanup();
return 0;
}
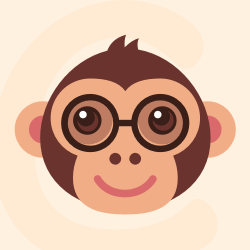



Ultralightweight JSON parser in ANSI C
最近提交(Master分支:1 个月前 )
424ce4ce
This reverts commit 5b502cdbfb21fbe5f6cf9ffbd2b96e4281a741e6.
Related to #860
2 个月前
32497300 - 4 个月前
更多推荐
所有评论(0)