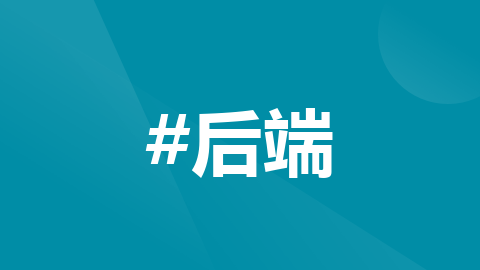
SpringBoot动态读取nacos配置(配置热更新)

在微服务架构中,配置的动态更新对于应用的灵活性和可维护性至关重要。Spring Cloud 提供了一种机制,即@RefreshScope
注解,用于实现配置的热更新。本文将介绍配置热更新的原理以及@RefreshScope
的作用。
配置热更新原理
配置热更新的原理基于 Spring Cloud Config 和 Spring Cloud Bus。
-
Spring Cloud Config: Spring Cloud Config 允许将应用的配置集中存储在远程版本控制仓库(如 Git、SVN)中,并通过 HTTP 或消息代理服务(如 RabbitMQ、Kafka)向应用提供配置信息。应用启动时会从配置服务器获取配置信息。
-
Spring Cloud Bus: Spring Cloud Bus 是一个事件总线,用于在分布式系统中传播状态更改。它基于消息代理服务,当应用的配置发生变化时,通过 Spring Cloud Bus 可以广播消息,通知所有相关的微服务实例进行配置更新。
结合 Spring Cloud Config 和 Spring Cloud Bus,实现配置热更新的流程如下:
- 开发人员或运维人员更新配置文件存储在配置服务器中。
- 配置服务器接收到配置更新后,通过 Spring Cloud Bus 向消息代理服务发送配置变更的消息。
- 订阅了消息代理服务的微服务实例接收到消息后,重新从配置服务器获取最新的配置信息,并应用到应用程序中。
@RefreshScope的作用
@RefreshScope
注解用于标记 Spring Bean,表明该 Bean 的属性在配置发生变化时需要进行动态更新。当配置更新后,Spring 容器会重新创建带有@RefreshScope
注解的 Bean,从而使得新的配置生效。
具体实现步骤如下:
-
Bean创建阶段: 当应用启动时,带有
@RefreshScope
注解的 Bean 被创建并初始化。这些 Bean 的属性值被填充为应用启动时配置的值。 -
配置更新阶段: 当配置发生变化时,Spring Cloud Bus 广播配置变更的消息。
-
动态更新阶段: 订阅了消息代理服务的微服务实例接收到消息后,Spring Cloud Config 会重新加载配置,而带有
@RefreshScope
注解的 Bean 会被标记为“脏”(dirty)。在 Bean 被访问时,Spring 容器会检测到该 Bean 是“脏”的,重新创建并初始化该 Bean,从而更新 Bean 的属性值为最新的配置值。
示例
以下是一个简单的示例,演示了如何在 Spring Boot 中使用@RefreshScope
实现配置的热更新:
import org.springframework.beans.factory.annotation.Value;
import org.springframework.cloud.context.config.annotation.RefreshScope;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RefreshScope
public class ConfigController {
@Value("${message:Hello World}")
private String message;
@GetMapping("/message")
public String getMessage() {
return message;
}
}
在这个示例中,message
属性被@Value
注解注入,并带有@RefreshScope
注解。当配置发生变化时,message
属性的值会动态更新。
实战案例
在程序中 我需要读取到动态配置的百分比比例值 但是不能每次更新都查询Mysql(造成IO浪费)
也不能每次更新项目(浪费时间)
所以选择使用@RefreshScope
import lombok.Data;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.cloud.context.config.annotation.RefreshScope;
import org.springframework.stereotype.Component;
/**
* @author zhangjiaxuan
*/
@Component
@ConfigurationProperties(prefix = "your.nacos.count.path")
@Data
@RefreshScope
public class YourClassName {
// 这里直接和nacos中参数对应就行
private Long day;
private Long year;
}
然后在需要动态读取参数的程序中引入YourClassName.class(这里自己定义名称)
@Resource
YourClassName yourClassName;
然后直接yourClassName.get
就可以实现动态读取nacos上的配置了
结论
通过 Spring Cloud Config 和 Spring Cloud Bus,以及@RefreshScope
注解,我们可以实现配置的热更新,从而提高了微服务架构下应用的灵活性和可维护性。配置热更新使得应用能够在运行时动态适应变化的环境,为持续集成和持续部署提供了基础支持。
通常使用方向:
程序开关/程序热更新数据/
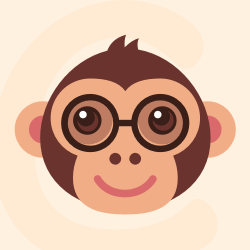



更多推荐
所有评论(0)