Java OCR tesseract 图像智能字符识别技术 Java代码实现

pb.directory(imageFile.getParentFile());
cmd.set(1, imageFile.getName());
pb.command(cmd);
pb.redirectErrorStream(true);
Process process = pb.start();
// tesseract.exe 1.jpg 1 -l chi_sim
// Runtime.getRuntime().exec(“tesseract.exe 1.jpg 1 -l chi_sim”);
/**
-
the exit value of the process. By convention, 0 indicates normal
-
termination.
*/
// System.out.println(cmd.toString());
int w = process.waitFor();
if (w == 0)// 0代表正常退出
{
BufferedReader in = new BufferedReader(new InputStreamReader(
new FileInputStream(outputFile.getAbsolutePath() + “.txt”),
“UTF-8”));
String str;
while ((str = in.readLine()) != null)
{
strB.append(str).append(EOL);
}
in.close();
} else
{
String msg;
switch (w)
{
case 1:
msg = “Errors accessing files. There may be spaces in your image’s filename.”;
break;
case 29:
msg = “Cannot recognize the image or its selected region.”;
break;
case 31:
msg = “Unsupported image format.”;
break;
default:
msg = “Errors occurred.”;
}
throw new RuntimeException(msg);
}
new File(outputFile.getAbsolutePath() + “.txt”).delete();
return strB.toString().replaceAll(“\s*”, “”);
}
}
代码很简单,中间那部分ProcessBuilder其实就类似Runtime.getRuntime().exec(“tesseract.exe 1.jpg 1 -l chi_sim”),大家不习惯的可以使用Runtime。
测试代码:
package com.zhy.test;
import java.io.File;
public class Test
{
public static void main(String[] args)
{
try
{
File testDataDir = new File(“testdata”);
System.out.println(testDataDir.listFiles().length);
int i = 0 ;
for(File file :testDataDir.listFiles())
{
i++ ;
String recognizeText = new OCRHelper().recognizeText(file);
System.out.print(recognizeText+“\t”);
if( i % 5 == 0 )
{
System.out.println();
}
}
} catch (Exception e)
{
e.printStackTrace();
}
}
}
输出结果:
对比第一张图片,是不是很完美~哈哈 ,当然了如果你只需要实现验证码的读写,那么上面就足够了。下面继续普及图像处理的知识。
-------------------------------------------------------------------我的分割线--------------------------------------------------------------------
当然了,有时候图片被扭曲或者模糊的很厉害,很不容易识别,所以下面我给大家介绍一个去噪的辅助类,绝对碉堡了,先看下效果图。
来张特写:
一个类,不依赖任何jar,把图像中的干扰线消灭了,是不是很给力,然后再拿这样的图片去识别,会不会效果更好呢,嘿嘿,大家自己实验~
代码:
package com.zhy.test;
import java.awt.Color;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
public class ClearImageHelper
{
public static void main(String[] args) throws IOException
{
File testDataDir = new File(“testdata”);
final String destDir = testDataDir.getAbsolutePath()+“/tmp”;
for (File file : testDataDir.listFiles())
{
cleanImage(file, destDir);
}
}
/**
-
@param sfile
-
需要去噪的图像
-
@param destDir
-
去噪后的图像保存地址
-
@throws IOException
*/
public static void cleanImage(File sfile, String destDir)
throws IOException
{
File destF = new File(destDir);
if (!destF.exists())
{
destF.mkdirs();
}
BufferedImage bufferedImage = ImageIO.read(sfile);
int h = bufferedImage.getHeight();
int w = bufferedImage.getWidth();
// 灰度化
int[][] gray = new int[w][h];
for (int x = 0; x < w; x++)
{
for (int y = 0; y < h; y++)
{
int argb = bufferedImage.getRGB(x, y);
// 图像加亮(调整亮度识别率非常高)
int r = (int) (((argb >> 16) & 0xFF) * 1.1 + 30);
int g = (int) (((argb >> 8) & 0xFF) * 1.1 + 30);
int b = (int) (((argb >> 0) & 0xFF) * 1.1 + 30);
if (r >= 255)
{
r = 255;
}
if (g >= 255)
{
g = 255;
}
if (b >= 255)
{
b = 255;
}
gray[x][y] = (int) Math
.pow((Math.pow(r, 2.2) * 0.2973 + Math.pow(g, 2.2)
- 0.6274 + Math.pow(b, 2.2) * 0.0753), 1 / 2.2);
}
}
// 二值化
int threshold = ostu(gray, w, h);
BufferedImage binaryBufferedImage = new BufferedImage(w, h,
BufferedImage.TYPE_BYTE_BINARY);
for (int x = 0; x < w; x++)
{
for (int y = 0; y < h; y++)
{
if (gray[x][y] > threshold)
{
gray[x][y] |= 0x00FFFF;
} else
{
gray[x][y] &= 0xFF0000;
}
binaryBufferedImage.setRGB(x, y, gray[x][y]);
}
}
// 矩阵打印
for (int y = 0; y < h; y++)
{
for (int x = 0; x < w; x++)
{
if (isBlack(binaryBufferedImage.getRGB(x, y)))
{
System.out.print(“*”);
} else
{
System.out.print(" ");
}
}
System.out.println();
}
ImageIO.write(binaryBufferedImage, “jpg”, new File(destDir, sfile
.getName()));
}
public static boolean isBlack(int colorInt)
{
Color color = new Color(colorInt);
if (color.getRed() + color.getGreen() + color.getBlue() <= 300)
{
return true;
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数Java工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录大纲截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且后续会持续更新
如果你觉得这些内容对你有帮助,可以添加V获取:vip1024b (备注Java)
最后
文章中涉及到的知识点我都已经整理成了资料,录制了视频供大家下载学习,诚意满满,希望可以帮助在这个行业发展的朋友,在论坛博客等地方少花些时间找资料,把有限的时间,真正花在学习上,所以我把这些资料,分享出来。相信对于已经工作和遇到技术瓶颈的朋友们,在这份资料中一定都有你需要的内容。
47046426)]
[外链图片转存中…(img-EfRYdFbi-1711947046427)]
[外链图片转存中…(img-WGvb7JWA-1711947046427)]
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录大纲截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且后续会持续更新
如果你觉得这些内容对你有帮助,可以添加V获取:vip1024b (备注Java)
[外链图片转存中…(img-OZlfnYiB-1711947046427)]
最后
文章中涉及到的知识点我都已经整理成了资料,录制了视频供大家下载学习,诚意满满,希望可以帮助在这个行业发展的朋友,在论坛博客等地方少花些时间找资料,把有限的时间,真正花在学习上,所以我把这些资料,分享出来。相信对于已经工作和遇到技术瓶颈的朋友们,在这份资料中一定都有你需要的内容。
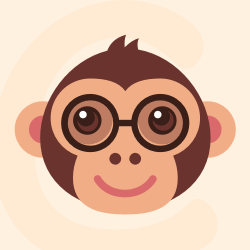



更多推荐
所有评论(0)