docker---java调用Harbor API
harbor
Harbor 是一个开源的容器镜像仓库,用于存储和管理 Docker 镜像和其他容器镜像。 * 容器镜像仓库、存储和管理 Docker 镜像和其他容器镜像 * 有什么特点:支持多种镜像格式、易于使用、安全性和访问控制
项目地址:https://gitcode.com/gh_mirrors/ha/harbor

·
注:sringBoot项目调用Harbor API
1.pom文件
<!-- apache httpclient组件 -->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.5</version>
</dependency>
2.yml文件
#私服仓库配置
harbor:
host: harbor的ip
url: http://harbor的ip
login_address: Harbor的登录地址
username: xx
password: xxx
encoding: UTF-8
connect_timeout: 6000
socket_timeout: 6000
user_agent: Mozilla/5.0 (Windows NT 6.1) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/80.0.3987.163 Safari/537.36
3.工具类
import org.apache.commons.lang.StringUtils;
import org.apache.http.HttpStatus;
import org.apache.http.NameValuePair;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.*;
import org.apache.http.client.utils.URIBuilder;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* httpClient工具类
*/
@Component
public class HttpClientUtils {
//编码格式
@Value("${harbor.encoding}")
private String ENCODING;
//设置连接超时,单位毫秒
@Value("${harbor.connect_timeout}")
private int CONNECT_TIMEOUT;
//设置读取数据超时时间,单位毫秒
@Value("${harbor.socket_timeout}")
private int SOCKET_TIMEOUT;
//Harbor url
@Value("${harbor.url}")
private String HOST_PORT;
//Harbor的登录地址
@Value("${harbor.login_address}")
private String HARBOR_LOGIN_ADDRESS;
//请求头 用户代理
@Value("${harbor.user_agent}")
private String USER_AGENT;
//Harbor登录用户名
@Value("${harbor.username}")
private String HARBOR_USERNAME;
//Harbor登录密码
@Value("${harbor.password}")
private String HARBOR_PASSWORD;
/**
* 登录操作
*
* @throws Exception
*/
public CloseableHttpClient login() throws Exception {
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(HARBOR_LOGIN_ADDRESS);
httpPost.setHeader("User_agent", USER_AGENT);
//登录表单提交的数据
List<NameValuePair> list = new ArrayList<NameValuePair>();
list.add(new BasicNameValuePair("principal", HARBOR_USERNAME));
list.add(new BasicNameValuePair("password", HARBOR_PASSWORD));
UrlEncodedFormEntity entity = new UrlEncodedFormEntity(list, ENCODING);
httpPost.setEntity(entity);
//相当于按下登录按钮
CloseableHttpResponse response = httpClient.execute(httpPost);
return httpClient;
}
/**
* 释放资源
*
* @param response
* @param httpClient
* @throws Exception
*/
public void release(CloseableHttpResponse response, CloseableHttpClient httpClient) throws Exception {
if (response != null) {
response.close();
}
if (httpClient != null) {
httpClient.close();
}
}
/**
* 执行请求并封装结果
*
* @param httpResponse
* @param httpClient
* @param httpMethod
* @return
* @throws Exception
*/
public ResultObject getHttpClientResult(CloseableHttpResponse httpResponse, CloseableHttpClient httpClient, HttpRequestBase httpMethod) throws Exception {
//执行请求
httpResponse = httpClient.execute(httpMethod);
Map<String, Object> map = new HashMap<>();
//封装结果
if (httpResponse != null && httpResponse.getStatusLine() != null) {
String records = EntityUtils.toString(httpResponse.getEntity(), ENCODING);
if (StringUtils.isNotBlank(records)) {
map.put("records",records);
}
return new ResultObject(httpResponse.getStatusLine().getStatusCode(), "", map);
}
return new ResultObject(HttpStatus.SC_INTERNAL_SERVER_ERROR, "请求失败", map);
}
/**
* 发送get请求
*
* @param uri
* @param params
* @throws Exception
*/
public ResultObject get(String uri, Map<String, String> params) throws Exception {
CloseableHttpClient httpClient = login();
URIBuilder uriBuilder = new URIBuilder(HOST_PORT + uri);
if (params.size() > 0) {
for (Map.Entry<String, String> m : params.entrySet()) {
uriBuilder.setParameter(m.getKey(), m.getValue());
}
}
HttpGet get = new HttpGet(uriBuilder.build());
RequestConfig requestCongfig = RequestConfig.custom().setConnectionRequestTimeout(CONNECT_TIMEOUT).setSocketTimeout(SOCKET_TIMEOUT).build();
get.setConfig(requestCongfig);
CloseableHttpResponse response = null;
ResultObject resultObject = getHttpClientResult(response, httpClient, get);
release(response, httpClient);
return resultObject;
}
/**
* 发送 post请求
*
* @param uri
* @param params
* @throws Exception
*/
public ResultObject post(String uri, Map<String, String> params) throws Exception {
CloseableHttpClient httpClient = login();
HttpPost post = new HttpPost(HOST_PORT + uri);
RequestConfig requestCongfig = RequestConfig.custom().setConnectionRequestTimeout(CONNECT_TIMEOUT).setSocketTimeout(SOCKET_TIMEOUT).build();
post.setConfig(requestCongfig);
String json = JSONObject.toJSONString(params);
//post Request Payload
StringEntity stringEntity = new StringEntity(json, "application/json", ENCODING);
post.setEntity(stringEntity);
CloseableHttpResponse response = null;
ResultObject resultObject = getHttpClientResult(response, httpClient, post);
release(response, httpClient);
return resultObject;
}
/**
* 发送delete请求
*
* @param uri
* @param params
* @throws Exception
*/
public ResultObject delete(String uri, Map<String, String> params) throws Exception {
CloseableHttpClient httpClient = login();
URIBuilder uriBuilder = new URIBuilder(HOST_PORT + uri);
if (params.size() > 0) {
for (Map.Entry<String, String> m : params.entrySet()) {
uriBuilder.setParameter(m.getKey(), m.getValue());
}
}
HttpDelete delete = new HttpDelete(uriBuilder.build());
RequestConfig requestCongfig = RequestConfig.custom().setConnectionRequestTimeout(CONNECT_TIMEOUT).setSocketTimeout(SOCKET_TIMEOUT).build();
delete.setConfig(requestCongfig);
CloseableHttpResponse response = null;
ResultObject resultObject = getHttpClientResult(response, httpClient, delete);
release(response, httpClient);
return resultObject;
}
/**
* 发送put请求
*
* @param uri
* @param params
* @throws Exception
*/
public ResultObject put(String uri, Map<String, String> params) throws Exception {
CloseableHttpClient httpClient = login();
URIBuilder uriBuilder = new URIBuilder(HOST_PORT + uri);
if (params.size() > 0) {
for (Map.Entry<String, String> m : params.entrySet()) {
uriBuilder.setParameter(m.getKey(), m.getValue());
}
}
HttpPut put = new HttpPut(uriBuilder.build());
RequestConfig requestCongfig = RequestConfig.custom().setConnectionRequestTimeout(CONNECT_TIMEOUT).setSocketTimeout(SOCKET_TIMEOUT).build();
put.setConfig(requestCongfig);
CloseableHttpResponse response = null;
ResultObject resultObject = getHttpClientResult(response, httpClient, put);
release(response, httpClient);
return resultObject;
}
}
4.controller
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import io.swagger.annotations.*;
import org.apache.commons.lang.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.*;
import java.io.File;
import java.math.BigDecimal;
import java.util.HashMap;
import java.util.Map;
/**
* harbor控制器
*/
@RestController
public class HarborController {
@Autowired
private HttpClientUtils httpClientUtils;
//Harbor host
@Value("${harbor.host}")
private String HOST_HOST;
@GetMapping("/deleteProjectByProjectID")
public ResultObject deleteProjects(Integer project_id) throws Exception {
String uri = "/api/projects/" + project_id;
ResultObject resultObject = httpClientUtils.get(uri, new HashMap<>());
if (resultObject.getData() != null) {
Map<String, Object> map = (Map<String, Object>) resultObject.getData();
if (StringUtils.isNotBlank((String) map.get("records"))) {
JSONObject jsonObject = JSONObject.parseObject((String) map.get("records"));
if ((int) jsonObject.get("repo_count") > 0) {
return ResultObject.error("项目中有镜像,要删除项目,需先删除镜像");
}
}
}
return httpClientUtils.delete(uri, new HashMap<>());
}
@PostMapping("/createProjects")
public ResultObject createProjects(String projectName) throws Exception {
String uri = "/api/projects";
ResultObject result = httpClientUtils.get(uri, new HashMap<>());
if (result.getData() != null && StringUtils.isNotBlank(projectName)) {
Map<String, Object> map = (Map<String, Object>) result.getData();
JSONArray jsonArray = JSONArray.parseArray((String) map.get("records"));
for (int i = 0; i < jsonArray.size(); i++) {
JSONObject jsonObject = (JSONObject) jsonArray.get(i);
if (projectName.equals(jsonObject.get("name"))) {
return ResultObject.error("项目名称已存在");
}
}
}
Map<String, String> params = new HashMap<>();
params.put("project_name", projectName);
return httpClientUtils.post(uri, params);
}
@GetMapping("/deleteImageByImageName")
public ResultObject deleteImageByImageName(String projectName, String imageName) throws Exception {
String uri = "/api/repositories/" + projectName + "/" + imageName;
return httpClientUtils.delete(uri, new HashMap<String, String>());
}
@GetMapping("/getImageDescByImageNameAndTag")
public ResultObject getImageDescByImageNameAndTag(String projectName, String imageName, String tag) throws Exception {
String uri = "/api/repositories/" + projectName + "/" + imageName + "/tags/" + tag;
return httpClientUtils.get(uri, new HashMap<>());
}
@GetMapping("/deleteImageByImageNameAndTag")
public ResultObject deleteImageByImageNameAndTag(String projectName, String imageName, String tag) throws Exception {
String uri = "/api/repositories/" + projectName + "/" + imageName + "/tags/" + tag;
return httpClientUtils.delete(uri, new HashMap<String, String>());
}
}
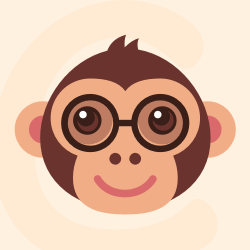



Harbor 是一个开源的容器镜像仓库,用于存储和管理 Docker 镜像和其他容器镜像。 * 容器镜像仓库、存储和管理 Docker 镜像和其他容器镜像 * 有什么特点:支持多种镜像格式、易于使用、安全性和访问控制
最近提交(Master分支:2 个月前 )
9e55afbb
pull image from registry.goharbor.io instead of dockerhub
Update testcase to support Docker Image Can Be Pulled With Credential
Change gitlab project name when user changed.
Update permissions count and permission count total
Change webhook_endpoint_ui
Signed-off-by: stonezdj <stone.zhang@broadcom.com>
Co-authored-by: Wang Yan <wangyan@vmware.com> 9 天前
3dbfd422
Signed-off-by: wang yan <wangyan@vmware.com> 10 天前
更多推荐
所有评论(0)