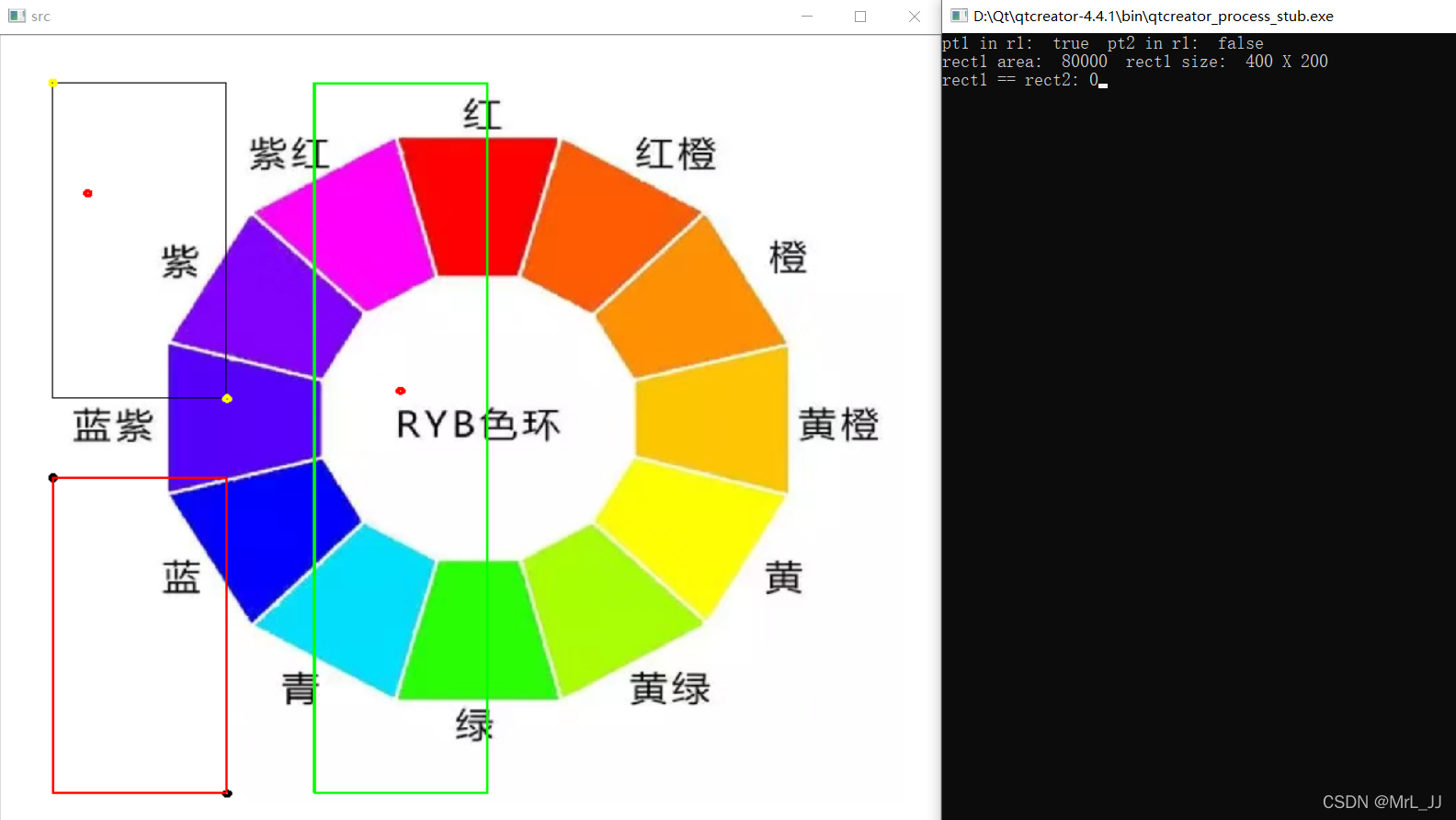
opencv 矩形cv::Rect数据结构详解
opencv
OpenCV: 开源计算机视觉库
项目地址:https://gitcode.com/gh_mirrors/opencv31/opencv

·
一、源码
template<typename _Tp> class Rect_
{
public:
typedef _Tp value_type;
//! default constructor
Rect_();
Rect_(_Tp _x, _Tp _y, _Tp _width, _Tp _height);
Rect_(const Rect_& r);
Rect_(Rect_&& r) CV_NOEXCEPT;
Rect_(const Point_<_Tp>& org, const Size_<_Tp>& sz);
Rect_(const Point_<_Tp>& pt1, const Point_<_Tp>& pt2);
Rect_& operator = ( const Rect_& r );
Rect_& operator = ( Rect_&& r ) CV_NOEXCEPT;
//! the top-left corner
Point_<_Tp> tl() const;
//! the bottom-right corner
Point_<_Tp> br() const;
//! size (width, height) of the rectangle
Size_<_Tp> size() const;
//! area (width*height) of the rectangle
_Tp area() const;
//! true if empty
bool empty() const;
//! conversion to another data type
template<typename _Tp2> operator Rect_<_Tp2>() const;
//! checks whether the rectangle contains the point
bool contains(const Point_<_Tp>& pt) const;
_Tp x; //!< x coordinate of the top-left corner
_Tp y; //!< y coordinate of the top-left corner
_Tp width; //!< width of the rectangle
_Tp height; //!< height of the rectangle
};
typedef Rect_<int> Rect2i;
typedef Rect_<float> Rect2f;
typedef Rect_<double> Rect2d;
typedef Rect2i Rect;
Rect_是一个模板类,矩形由点、长和宽基本属性组成,opencv内部通过
typedef关键字分别以int 、float 和double三种基础类型命名了模板类Rect_的类型别名Rect2i,Rect2f,Rect2d,而Rect又是Rect2i 的类型别名。
二、cv::Rect的成员和属性
1.成员
rect.tl()//矩形左上角点的坐标
rect.br()//矩形右下角点的坐标
rect.size()//矩形的大小
rect.area()//矩形的面积
rect.empty() //判断矩形是否为空
rect.contains() //判断一个点是否在矩形区域内
2.属性
rect.x; //表示左上角点x的坐标
rect.y; //表示左上角点y的坐标
rect.width; //表示矩形的宽度
rect.height; //表示矩形的高度
三、代码示例
void test_Rect()
{
cv::Mat src;
src = cv::imread("D:\\QtProject\\Opencv_Example\\rect\\rect.jpg", cv::IMREAD_COLOR);
if (src.empty()) {
cout << "Cannot load image" << endl;
return;
}
//show(src);
// 绘制矩形框
cv::Rect rect1(60, 60, 200, 400);
cv::rectangle(src, rect1, cv::Scalar(0, 0, 0), 1, 8, 0);
// tl(): 矩形左上角点的坐标 br():矩形右下角点的坐标
cv::circle(src, rect1.tl(), 3, cv::Scalar(0, 255, 255), 3, 8, 0);
cv::circle(src, rect1.br(), 3, cv::Scalar(0, 255, 255), 3, 8, 0);
// 判定一个点是否在矩形内
cv::Point pt1(100, 200);
cv::Point pt2(459, 450);
cv::circle(src, pt1, 3, cv::Scalar(0, 0, 255), 3, 8, 0);
cv::circle(src, pt2, 3, cv::Scalar(0, 0, 255), 3, 8, 0);
qDebug()<< "pt1 in r1: " << rect1.contains(pt1) << " pt2 in r1: " << rect1.contains(pt2) <<'\t';
qDebug()<< "rect1 area: " << rect1.area() << " rect1 size: " << rect1.size().height<<"X"<<rect1.size().width<<'\t';
// 平移rect1 到 movePt
cv::Point movePt(0, 500); //向Y轴移动500
cv::Rect rect2 = rect1 + movePt;
// tl(): 矩形左上角点的坐标 br():矩形右下角点的坐标
cv::circle(src, rect2.tl(), 3, cv::Scalar(0, 0, 0), 3, 8, 0);
cv::circle(src, rect2.br(), 3, cv::Scalar(0, 0, 0), 3, 8, 0);
cv::rectangle(src, rect2, cv::Scalar(0, 0, 255), 2, 8, 0);
//比较两个矩形
std::cout << "rect1 == rect2: "<< std::to_string(rect1 == rect2);
//求两个矩形的并
cv::Rect rect3 = rect1 | rect2;
cv::Point movePt2(300, 0); //向Y轴移动500
cv::Rect rect4 = rect3 + movePt2;
cv::rectangle(src, rect4, cv::Scalar(0, 255, 0), 2, 8, 0);
imshow("src",src);
//排序矩形
//std::vector<cv::Rect> Rect;
//Rect.push_back(rect1);
//Rect.push_back(rect2);
//Rect.push_back(rect3);
//std::sort(Rect.begin(), Rect.end(), compareRect_y);
//for (cv::Rect rect : Rect) {
//std::cout << "sorted rect: "<<rect.tl() << " rect.area: " << rect.area()<<std::endl;
//}
}
四、运行效果
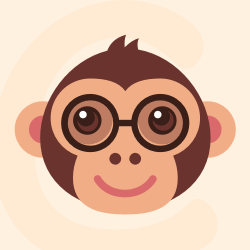



OpenCV: 开源计算机视觉库
最近提交(Master分支:2 个月前 )
c3747a68
Added Universal Windows Package build to CI. 1 天前
9b635da5 - 1 天前
更多推荐
所有评论(0)