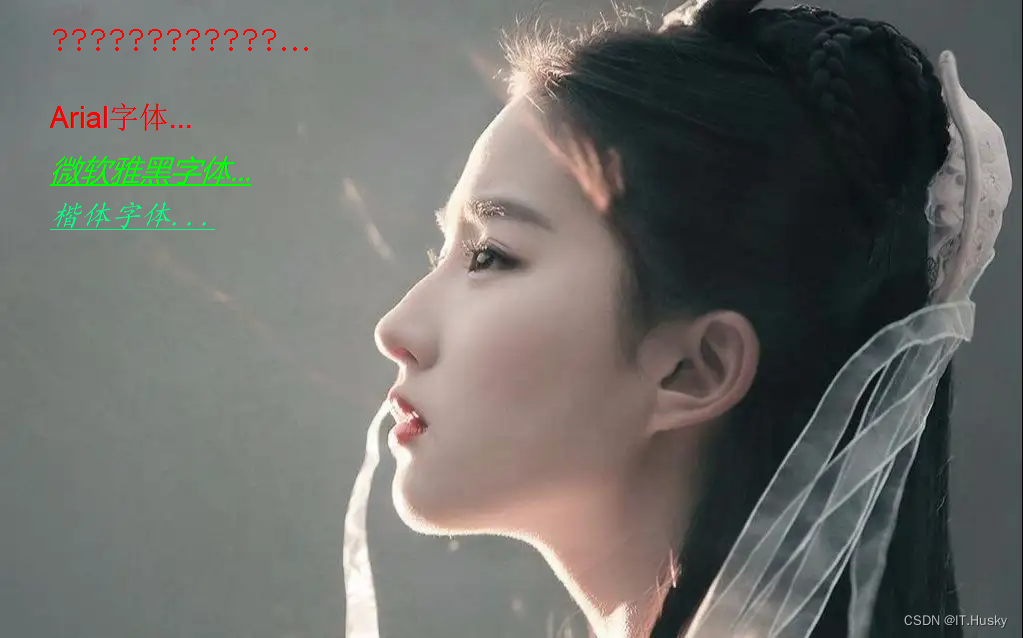
C++ OpenCV【解决putText不能显示中文】
opencv
OpenCV: 开源计算机视觉库
项目地址:https://gitcode.com/gh_mirrors/opencv31/opencv

·
使用cv::putText写中文字符时输出结果为"??????"。。。。。。这怎么能忍?
python方法中可以将opencv图片转化为PIL,写中文之后再转回opencv格式。
C++方法中通常利用freetype库来实现,freetype打包的win32静态库可以在C#通过dll引用进行调用,这需要其版本跟CvxText对应,否则会出现错误,其在x64平台也一样。
本文采用windows的GDI显示系统的TrueType字体,没有封装,就两个函数,分成h和cpp文件,可以自己编辑文件名和函数名,也可以直接将cpp的代码复制到你需要的程序中。
【putText.h】
#ifndef PUTTEXT_H_
#define PUTTEXT_H_
#include <windows.h>
#include <string>
#include <opencv2/opencv.hpp>
using namespace cv;
void GetStringSize(HDC hDC, const char* str, int* w, int* h);
void putTextHusky(Mat &dst, const char* str, Point org, Scalar color, int fontSize,
const char *fn = "Arial", bool italic = false, bool underline = false);
#endif // PUTTEXT_H_
【putText.cpp】
#include "putText.h"
void GetStringSize(HDC hDC, const char* str, int* w, int* h)
{
SIZE size;
GetTextExtentPoint32A(hDC, str, strlen(str), &size);
if (w != 0) *w = size.cx;
if (h != 0) *h = size.cy;
}
void putTextHusky(Mat &dst, const char* str, Point org, Scalar color, int fontSize, const char* fn, bool italic, bool underline)//后俩参数:斜体,下划线
{
CV_Assert(dst.data != 0 && (dst.channels() == 1 || dst.channels() == 3));
int x, y, r, b;
if (org.x > dst.cols || org.y > dst.rows) return;
x = org.x < 0 ? -org.x : 0;
y = org.y < 0 ? -org.y : 0;
LOGFONTA lf;
lf.lfHeight = -fontSize;
lf.lfWidth = 0;
lf.lfEscapement = 0;
lf.lfOrientation = 0;
lf.lfWeight = 5;
lf.lfItalic = italic; //斜体
lf.lfUnderline = underline; //下划线
lf.lfStrikeOut = 0;
lf.lfCharSet = DEFAULT_CHARSET;
lf.lfOutPrecision = 0;
lf.lfClipPrecision = 0;
lf.lfQuality = PROOF_QUALITY;
lf.lfPitchAndFamily = 0;
strcpy_s(lf.lfFaceName, fn);
HFONT hf = CreateFontIndirectA(&lf);
HDC hDC = CreateCompatibleDC(0);
HFONT hOldFont = (HFONT)SelectObject(hDC, hf);
int strBaseW = 0, strBaseH = 0;
int singleRow = 0;
char buf[1 << 12];
strcpy_s(buf, str);
char *bufT[1 << 12]; // 这个用于分隔字符串后剩余的字符,可能会超出。
//处理多行
{
int nnh = 0;
int cw, ch;
const char* ln = strtok_s(buf, "\n", bufT);
while (ln != 0)
{
GetStringSize(hDC, ln, &cw, &ch);
strBaseW = max(strBaseW, cw);
strBaseH = max(strBaseH, ch);
ln = strtok_s(0, "\n", bufT);
nnh++;
}
singleRow = strBaseH;
strBaseH *= nnh;
}
if (org.x + strBaseW < 0 || org.y + strBaseH < 0)
{
SelectObject(hDC, hOldFont);
DeleteObject(hf);
DeleteObject(hDC);
return;
}
r = org.x + strBaseW > dst.cols ? dst.cols - org.x - 1 : strBaseW - 1;
b = org.y + strBaseH > dst.rows ? dst.rows - org.y - 1 : strBaseH - 1;
org.x = org.x < 0 ? 0 : org.x;
org.y = org.y < 0 ? 0 : org.y;
BITMAPINFO bmp = { 0 };
BITMAPINFOHEADER& bih = bmp.bmiHeader;
int strDrawLineStep = strBaseW * 3 % 4 == 0 ? strBaseW * 3 : (strBaseW * 3 + 4 - ((strBaseW * 3) % 4));
bih.biSize = sizeof(BITMAPINFOHEADER);
bih.biWidth = strBaseW;
bih.biHeight = strBaseH;
bih.biPlanes = 1;
bih.biBitCount = 24;
bih.biCompression = BI_RGB;
bih.biSizeImage = strBaseH * strDrawLineStep;
bih.biClrUsed = 0;
bih.biClrImportant = 0;
void* pDibData = 0;
HBITMAP hBmp = CreateDIBSection(hDC, &bmp, DIB_RGB_COLORS, &pDibData, 0, 0);
CV_Assert(pDibData != 0);
HBITMAP hOldBmp = (HBITMAP)SelectObject(hDC, hBmp);
//color.val[2], color.val[1], color.val[0]
SetTextColor(hDC, RGB(255, 255, 255));
SetBkColor(hDC, 0);
//SetStretchBltMode(hDC, COLORONCOLOR);
strcpy_s(buf, str);
const char* ln = strtok_s(buf, "\n", bufT);
int outTextY = 0;
while (ln != 0)
{
TextOutA(hDC, 0, outTextY, ln, strlen(ln));
outTextY += singleRow;
ln = strtok_s(0, "\n", bufT);
}
uchar* dstData = (uchar*)dst.data;
int dstStep = dst.step / sizeof(dstData[0]);
unsigned char* pImg = (unsigned char*)dst.data + org.x * dst.channels() + org.y * dstStep;
unsigned char* pStr = (unsigned char*)pDibData + x * 3;
for (int tty = y; tty <= b; ++tty)
{
unsigned char* subImg = pImg + (tty - y) * dstStep;
unsigned char* subStr = pStr + (strBaseH - tty - 1) * strDrawLineStep;
for (int ttx = x; ttx <= r; ++ttx)
{
for (int n = 0; n < dst.channels(); ++n) {
double vtxt = subStr[n] / 255.0;
int cvv = vtxt * color.val[n] + (1 - vtxt) * subImg[n];
subImg[n] = cvv > 255 ? 255 : (cvv < 0 ? 0 : cvv);
}
subStr += 3;
subImg += dst.channels();
}
}
SelectObject(hDC, hOldBmp);
SelectObject(hDC, hOldFont);
DeleteObject(hf);
DeleteObject(hBmp);
DeleteDC(hDC);
}
第二个函数putTextHusky(),默认使用Arial字体,也可以设置成操作系统中已经安装的字体,如“宋体”、“微软雅黑”、“Times New Roman”等;默认显示非斜体、非下划线。
【示例】
#include <opencv2/opencv.hpp>
#include "putText.h"
using namespace std;
using namespace cv;
int main()//自己写库解决putText无法显示中文字符问题
{
Mat img = imread("lyf.png");
putText(img, "中文无法显示...", Point(50, 50), 2, 1, Scalar(0, 0, 255), 1, 8, false);
putTextHusky(img, "Arial字体...", Point(50, 100), Scalar(0, 0, 255), 30, "Arial");
putTextHusky(img, "微软雅黑字体...", Point(50, 150), Scalar(0, 255, 0), 30, "微软雅黑", true, true);
putTextHusky(img, "楷体字体...\n", Point(50, 200), Scalar(128, 255, 0), 30, "楷体", true, true);
//imwrite("lyfWrite.png", img);
imshow("test", img);
waitKey(0);
system("pause");
return 0;
}
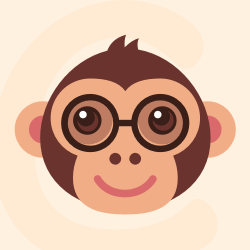



OpenCV: 开源计算机视觉库
最近提交(Master分支:2 个月前 )
ee95bfe2
Use generic SIMD in warpAffineBlocklineNN 18 小时前
ddc03c07
Disable SME2 branches in KleidiCV as it's incompatible with some CLang versions, e.g. NDK 28b1 1 天前
更多推荐
所有评论(0)