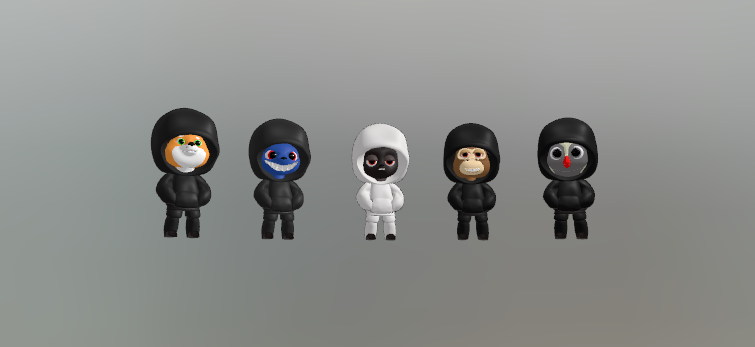
Three.js引入和加载3D 模型
·
在Three.js开发中,我们通常会导入各种不同格式的3D模型(GLTF、FBX、OBJ…)本文以vue3项目为例 引入和加载 GLTF格式模型
找到需要导入的GLTF模型,通常会有下面4个文件,将它们全部添加到自己的项目中(本文放到public文件夹中,这样的好处是在引入加载路径的时候只需要 ./xxx.gltf
就行了)
模型引入之后就可以直接在项目中加载模型了,具体实现代码如下:
<template>
<div ref="webgl"></div>
</template>
<script setup>
import { ref, onMounted } from 'vue'
import * as THREE from 'three'
import { OrbitControls } from 'three/examples/jsm/controls/OrbitControls'
import { GLTFLoader } from 'three/examples/jsm/loaders/GLTFLoader'
const webgl = ref()
// 本文为方便展示实现方式,全部写在onMounted中,可根据自己需求进行优化
onMounted(() => {
// 创建场景
const scene = new THREE.Scene()
scene.background = new THREE.Color(0xffffff)
// 创建相机
const camera = new THREE.PerspectiveCamera(90, window.innerWidth / window.innerHeight, 0.1, 1000)
// 创建渲染器
const renderer = new THREE.WebGLRenderer()
renderer.setSize(window.innerWidth, window.innerHeight)
webgl.value?.appendChild(renderer.domElement)
// 添加环境光
const ambientLight = new THREE.AmbientLight(0x404040)
scene.add(ambientLight)
// 添加平行光
const directionalLight = new THREE.DirectionalLight(0xffffff, 10)
directionalLight.position.set(1, 1, 1).normalize()
scene.add(directionalLight)
// 添加控制器
const controls = new OrbitControls(camera, renderer.domElement)
controls.enableDamping = true
controls.dampingFactor = 0.05
controls.screenSpacePanning = false
controls.minDistance = 2
controls.maxDistance = 10
controls.maxPolarAngle = Math.PI / 2
// 加载模型
const loader = new GLTFLoader()
loader.load('./scene.gltf', gltf => { // ./scene.gltf 需换成自己的文件名
console.log('gltf---', gltf)
scene.add(gltf.scene)
})
camera.position.set(0, 0, 5)
camera.lookAt(0, 0, 0)
function animate() {
requestAnimationFrame(animate)
renderer.render(scene, camera)
}
animate()
function onWindowResize() {
camera.aspect = window.innerWidth / window.innerHeight;
camera.updateProjectionMatrix();
renderer.setSize(window.innerWidth, window.innerHeight);
}
window.addEventListener('resize', onWindowResize);
})
</script>
通过上面的代码就可以实现最基础的加载外部3D模型了,效果图如下:
更多推荐
所有评论(0)