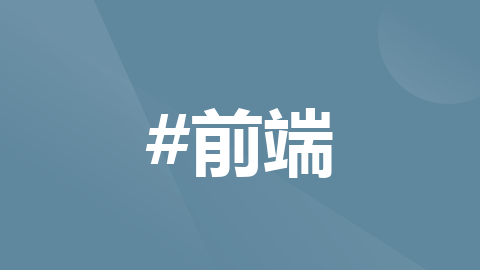
高德结合three.js实现景区大屏
·
在打造景区大屏时,结合高德地图和 three.js
可以实现一个交互性强、视觉效果好的大屏展示系统。这种系统可以用于展示景区地图、实时数据、3D 场景等。以下是实现步骤及代码示例:
实现步骤
- 初始化项目环境
- 集成高德地图 API
- 设置
three.js
3D 场景 - 将高德地图和
three.js
结合起来 - 添加交互和动态效果
- 优化和部署
1. 初始化项目环境
首先,确保你的项目中安装了 three.js
和有一个基本的 HTML 环境。
npm install three
在你的 HTML 文件中引入必要的库:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>智慧景区大屏</title>
<style>
body { margin: 0; }
#mapContainer { width: 100%; height: 100vh; position: absolute; top: 0; left: 0; }
#threeContainer { width: 100%; height: 100vh; position: absolute; top: 0; left: 0; }
</style>
</head>
<body>
<div id="mapContainer"></div>
<div id="threeContainer"></div>
<script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script>
<script src="https://webapi.amap.com/maps?v=1.4.15&key=你的高德地图 API 密钥"></script>
<script src="app.js"></script>
</body>
</html>
2. 集成高德地图 API
在 app.js
文件中,初始化高德地图并在页面上显示地图。
// app.js
// Initialize AMap
const map = new AMap.Map('mapContainer', {
center: [116.397428, 39.90923], // Default center
zoom: 13, // Default zoom level
viewMode: '3D' // Enable 3D view mode
});
3. 设置 three.js
3D 场景
创建一个 three.js
场景,并将其渲染到 threeContainer
中。
// Initialize Three.js
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.getElementById('threeContainer').appendChild(renderer.domElement);
camera.position.z = 5;
const geometry = new THREE.BoxGeometry();
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
const cube = new THREE.Mesh(geometry, material);
scene.add(cube);
function animate() {
requestAnimationFrame(animate);
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
renderer.render(scene, camera);
}
animate();
4. 将高德地图和 three.js
结合起来
为了将 three.js
画布与高德地图画布结合,你可以使用 CSS
进行布局,将两个画布叠加或并排显示。然后,使用 three.js
中的 Raycaster
或其他方法来实现地图和 3D 场景之间的交互。
// Example: Overlay Three.js on top of the AMap
const mapCanvas = document.querySelector('#mapContainer .amap-canvas');
mapCanvas.style.zIndex = '0'; // Set AMap canvas below Three.js canvas
document.querySelector('#threeContainer').style.zIndex = '1'; // Set Three.js canvas on top
5. 添加交互和动态效果
可以在 three.js
中实现与地图的交互。例如,可以将地图上的标记与 three.js
中的 3D 对象关联起来,或者根据地图的缩放和位置更新 3D 场景。
// Adding interaction (simplified example)
map.on('click', (e) => {
const coords = [e.lnglat.getLng(), e.lnglat.getLat()];
// Example: Update the position of a 3D object based on map click
cube.position.set(coords[0], coords[1], 0);
});
6. 优化和部署
最后,进行以下优化和部署步骤:
- 优化性能: 确保
three.js
场景和地图在大屏幕上流畅运行,减少不必要的计算。 - 适配大屏幕: 确保界面在大屏幕上显示良好,可能需要调整布局和样式。
- 部署: 将应用部署到 Web 服务器或大屏幕展示系统中。
完整代码示例
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>智慧景区大屏</title>
<style>
body { margin: 0; }
#mapContainer { width: 100%; height: 100vh; position: absolute; top: 0; left: 0; }
#threeContainer { width: 100%; height: 100vh; position: absolute; top: 0; left: 0; }
</style>
</head>
<body>
<div id="mapContainer"></div>
<div id="threeContainer"></div>
<script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script>
<script src="https://webapi.amap.com/maps?v=1.4.15&key=你的高德地图 API 密钥"></script>
<script>
// Initialize AMap
const map = new AMap.Map('mapContainer', {
center: [116.397428, 39.90923],
zoom: 13,
viewMode: '3D'
});
// Initialize Three.js
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.getElementById('threeContainer').appendChild(renderer.domElement);
camera.position.z = 5;
const geometry = new THREE.BoxGeometry();
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
const cube = new THREE.Mesh(geometry, material);
scene.add(cube);
function animate() {
requestAnimationFrame(animate);
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
renderer.render(scene, camera);
}
animate();
// Overlay Three.js on top of the AMap
const mapCanvas = document.querySelector('#mapContainer .amap-canvas');
mapCanvas.style.zIndex = '0';
document.querySelector('#threeContainer').style.zIndex = '1';
// Adding interaction example
map.on('click', (e) => {
const coords = [e.lnglat.getLng(), e.lnglat.getLat()];
cube.position.set(coords[0], coords[1], 0);
});
</script>
</body>
</html>
总结
通过上述步骤,你可以将高德地图与 three.js
结合,打造一个智慧景区大屏展示系统。这个系统可以显示 3D 场景、地图和动态数据,提供丰富的用户体验。在开发过程中,确保进行性能优化和用户测试,以实现最佳效果。
更多推荐
所有评论(0)