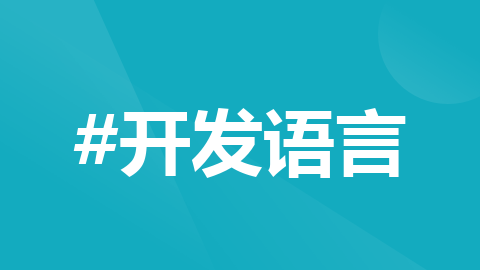
#if,#elif,#ifndef,#ifdef,#else,#endif等宏定义的详解
·
在C和C++编程中,预处理器指令(Preprocessor Directives)用于在编译之前对源代码进行处理。常见的预处理器指令包括#ifndef
,#ifdef
,#else
,#endif
,这些指令主要用于条件编译。下面将详细解释这些指令的含义和用法:
1. #ifndef
和 #ifdef
这两个指令用于检查某个宏是否已经定义。
#ifndef
:如果指定的宏没有被定义,则执行其后的代码块。#ifdef
:如果指定的宏已经被定义,则执行其后的代码块。
示例:
#define DEBUG
#ifndef RELEASE
// 代码块 A: 这个代码块将在宏 RELEASE 未定义时编译
printf("Release is not defined.\n");
#endif
#ifdef DEBUG
// 代码块 B: 这个代码块将在宏 DEBUG 定义时编译
printf("Debug mode is on.\n");
#endif
在这个例子中:
- 代码块 A 将被编译,因为
RELEASE
未被定义。 - 代码块 B 也将被编译,因为
DEBUG
已被定义。
2. #else
#else
用于指定在#if
、#ifdef
、#ifndef
条件不满足时执行的代码块。
示例:
#define FEATURE_X
#ifdef FEATURE_X
// 代码块 C: 这个代码块将在宏 FEATURE_X 定义时编译
printf("Feature X is enabled.\n");
#else
// 代码块 D: 这个代码块将在宏 FEATURE_X 未定义时编译
printf("Feature X is disabled.\n");
#endif
在这个例子中,如果 FEATURE_X
被定义,代码块 C 将被编译,否则代码块 D 将被编译。
3. #endif
#endif
用于结束一个条件编译块。每个 #if
、#ifdef
、#ifndef
块都必须有一个相应的 #endif
来结束。
示例:
#define ENABLE_FEATURE
#ifdef ENABLE_FEATURE
// 代码块 E: 这个代码块将在宏 ENABLE_FEATURE 定义时编译
printf("Feature is enabled.\n");
#endif
在这个例子中,#endif
标志着 #ifdef ENABLE_FEATURE
条件编译块的结束。
4. #if
和 #elif
除了#ifdef
和#ifndef
,还有更通用的条件编译指令#if
和#elif
:
#if
:用于在宏值的条件下编译代码。可以使用常量表达式来判断。#elif
:类似于else if
,用于在之前的#if
或#elif
条件不满足时,增加一个新的条件。
示例:
#define VALUE 10
#if VALUE > 5
// 代码块 F: 当 VALUE 大于 5 时编译
printf("Value is greater than 5.\n");
#elif VALUE == 5
// 代码块 G: 当 VALUE 等于 5 时编译
printf("Value is equal to 5.\n");
#else
// 代码块 H: 当 VALUE 小于 5 时编译
printf("Value is less than 5.\n");
#endif
在这个例子中,由于 VALUE
定义为 10,代码块 F 将被编译。
5. 综合示例
#define DEBUG
#define VERSION 2
#ifdef DEBUG
printf("Debug mode is active.\n");
#else
printf("Debug mode is not active.\n");
#endif
#if VERSION == 1
printf("Version 1.\n");
#elif VERSION == 2
printf("Version 2.\n");
#else
printf("Unknown version.\n");
#endif
在这个综合示例中:
- 因为
DEBUG
被定义了,所以打印Debug mode is active.
。 - 因为
VERSION
被定义为 2,所以打印Version 2.
。
条件编译的常见用途
-
避免重复包含头文件(Include Guards):防止头文件被多次包含,通常使用
#ifndef
和#define
。#ifndef MY_HEADER_H #define MY_HEADER_H // 头文件内容 #endif // MY_HEADER_H
-
调试代码:在开发和发布版本中编译不同的代码。
#ifdef DEBUG printf("Debugging info.\n"); #endif
-
根据配置编译不同的功能:根据配置选项选择编译哪些功能。
#ifdef FEATURE_X // 编译代码以支持 FEATURE_X #endif
-
跨平台代码:根据目标平台编译不同的代码。
#ifdef _WIN32 // Windows-specific code #elif defined(__linux__) // Linux-specific code #endif
使用这些预处理器指令,开发者可以灵活地控制源代码在不同条件下的编译行为,提高代码的可维护性和可移植性。
更多推荐
所有评论(0)