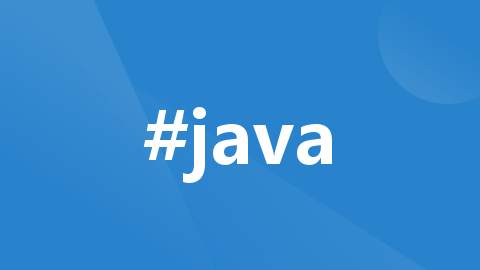
OpenAI开发中常见错误:OpenAIError和BadRequestError
·
错误1:openai.OpenAIError: The api_key client option must be set either by passing api_key.....
在通过openai创建客户端必须要设置api key,如果你事先已经在本机的环境中设置未起效可以手动设置,注意手动设置时不要用下面的形式
import openai
from openai import OpenAI
client = OpenAI()
openai.api_key = "YOUR API KEY"
如果你用的是client(OpenAI),这种方式不正确,正确的如下:
from openai import OpenAI
client = OpenAI(api_key="YOUR API KEY")
错误2: openai.BadRequestError: Error code: 400 - {'error': {'message':...
这个错误100%是因为你传入数据格式的原因,在这里需要参考文档来做,比如我实现的function calling,在这里需要描述函数,之前的描述为:
# 定义函数描述信息
function_description = {
"type": "function",
"function": "get_current_weather",
"description": "获取给定地点的当前天气",
"parameters": {
"type": "object",
"properties": {
"location": {
"type": "string",
"description": "城市名称,例如:“旧金山,CA”"
},
"unit": {
"type": "string",
"enum": ["celsius", "fahrenheit"]
}
},
"required": ["location"]
}
}
这时候无论怎么运行都会出错,即BadRequestError,但是其实是格式出错,如果按照下面代码:
# 定义函数描述信息
function_description = [{
"type": "function",
"function": {
"name": "get_current_weather",
"description": "获取给定地点的当前天气",
"parameters": {
"type": "object",
"properties": {
"location": {
"type": "string",
"description": "城市名称,例如:“旧金山,CA”"
},
"unit": {
"type": "string",
"enum": ["celsius", "fahrenheit"]
}
},
"required": ["location"]
}
}
}]
即正确,完整代码如下:
import json
from openai import OpenAI
def get_current_weather(location, unit="fahrenheit"):
# 实际为获取天气的接口
return json.dumps({
"location": location,
"weather": "rain",
"unit": unit,
})
if __name__ == '__main__':
# 定义客户端
client = OpenAI(api_key="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")
# 定义函数描述信息
function_description = [{
"type": "function",
"function": {
"name": "get_current_weather",
"description": "获取给定地点的当前天气",
"parameters": {
"type": "object",
"properties": {
"location": {
"type": "string",
"description": "城市名称,例如:“旧金山,CA”"
},
"unit": {
"type": "string",
"enum": ["celsius", "fahrenheit"]
}
},
"required": ["location"]
}
}
}]
# 发起调用
response = client.chat.completions.create(
model="gpt-3.5-turbo",
messages=[{"role": "user", "content": "旧金山当前的天气如何?"}],
tools=[function_description],
temperature=0
)
tool_calls = response.choices[0].message.tool_calls
# 如果模型确定要调用一个函数
if tool_calls:
# 获取模型生成的参数
arguments = json.loads(tool_calls[0].function.arguments)
# 调用本地函数
weather_info = get_current_weather(**arguments)
print(weather_info) # 我们可以在这里看到函数调用查询到的天气信息
输出:
{"location": "San Francisco, CA", "weather": "rain", "unit": "fahrenheit"}
更多推荐
所有评论(0)