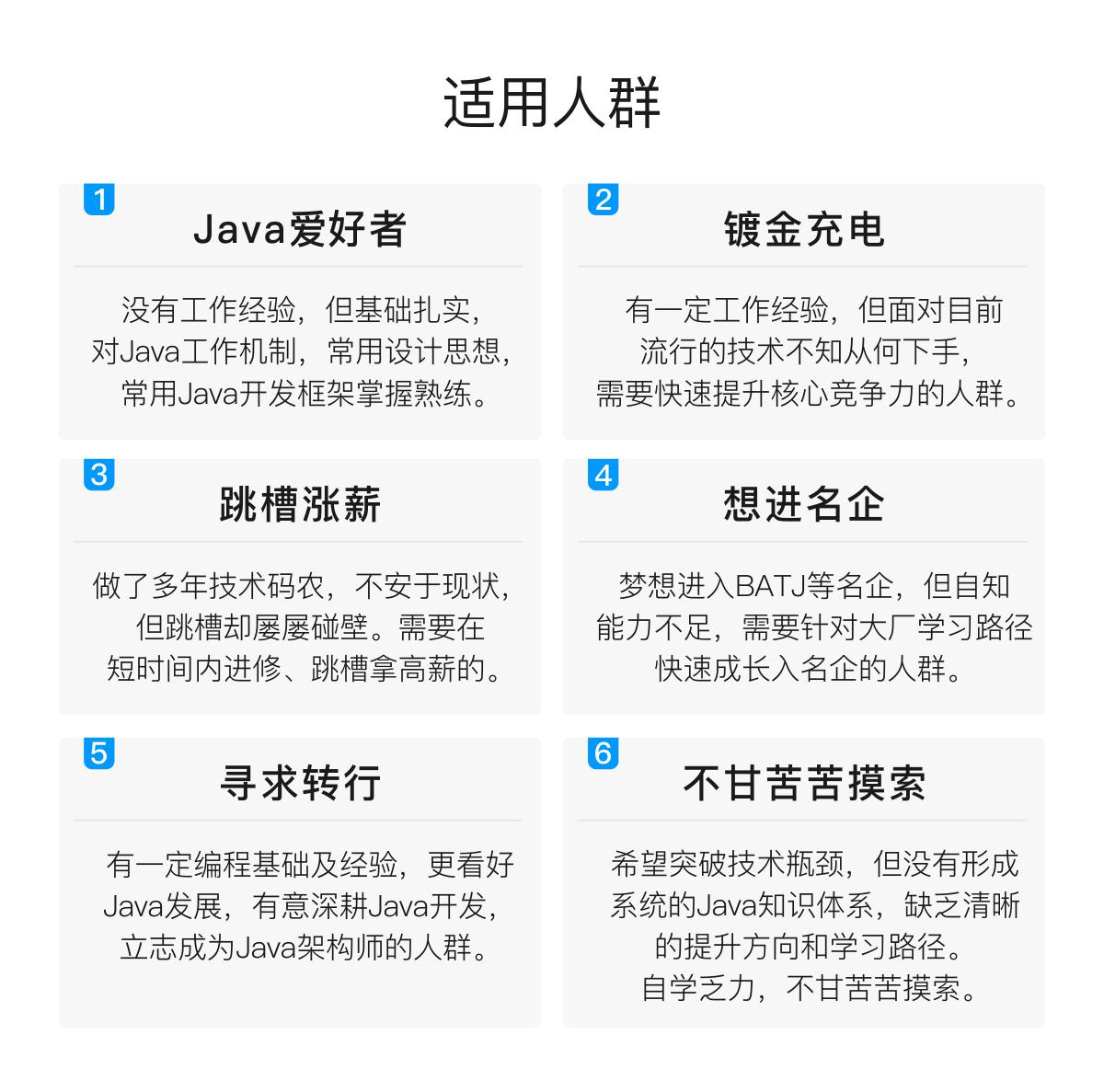
用Java实现JVM第五章《指令集和解释器》
return new DUP_X1();
case 0x5b:
return new DUP_X2();
case 0x5c:
return new DUP2();
case 0x5d:
return new DUP2_X1();
case 0x5e:
return new DUP2_X2();
case 0x5f:
return new SWAP();
case 0x60:
return new IADD();
case 0x61:
return new LADD();
case 0x62:
return new FADD();
case 0x63:
return new DADD();
case 0x64:
return new ISUB();
case 0x65:
return new LSUB();
case 0x66:
return new FSUB();
case 0x67:
return new DSUB();
case 0x68:
return new IMUL();
case 0x69:
return new LMUL();
case 0x6a:
return new FMUL();
case 0x6b:
return new DMUL();
case 0x6c:
return new IDIV();
case 0x6d:
return new LDIV();
case 0x6e:
return new FDIV();
case 0x6f:
return new DDIV();
case 0x70:
return new IREM();
case 0x71:
return new LREM();
case 0x72:
return new FREM();
case 0x73:
return new DREM();
case 0x74:
return new INEG();
case 0x75:
return new LNEG();
case 0x76:
return new FNEG();
case 0x77:
return new DNEG();
case 0x78:
return new ISHL();
case 0x79:
return new LSHL();
case 0x7a:
return new ISHR();
case 0x7b:
return new LSHR();
case 0x7c:
return new IUSHR();
case 0x7d:
return new LUSHR();
case 0x7e:
return new IAND();
case 0x7f:
return new LAND();
case (byte) 0x80:
return new IOR();
case (byte) 0x81:
return new LOR();
case (byte) 0x82:
return new IXOR();
case (byte) 0x83:
return new LXOR();
case (byte) 0x84:
return new IINC();
case (byte) 0x85:
return new I2L();
case (byte) 0x86:
return new I2F();
case (byte) 0x87:
return new I2D();
case (byte) 0x88:
return new L2I();
case (byte) 0x89:
return new L2F();
case (byte) 0x8a:
return new L2D();
case (byte) 0x8b:
return new F2I();
case (byte) 0x8c:
return new F2L();
case (byte) 0x8d:
return new F2D();
case (byte) 0x8e:
return new D2I();
case (byte) 0x8f:
return new D2L();
case (byte) 0x90:
return new D2F();
case (byte) 0x91:
return new I2B();
case (byte) 0x92:
return new I2C();
case (byte) 0x93:
return new I2S();
case (byte) 0x94:
return new LCMP();
case (byte) 0x95:
return new FCMPL();
case (byte) 0x96:
return new FCMPG();
case (byte) 0x97:
return new DCMPL();
case (byte) 0x98:
return new DCMPG();
case (byte) 0x99:
return new IFEQ();
case (byte) 0x9a:
return new IFNE();
case (byte) 0x9b:
return new IFLT();
case (byte) 0x9c:
return new IFGE();
case (byte) 0x9d:
return new IFGT();
case (byte) 0x9e:
return new IFLE();
case (byte) 0x9f:
return new IF_ICMPEQ();
case (byte) 0xa0:
return new IF_ICMPNE();
case (byte) 0xa1:
return new IF_ICMPLT();
case (byte) 0xa2:
return new IF_ICMPGE();
case (byte) 0xa3:
return new IF_ICMPGT();
case (byte) 0xa4:
return new IF_ICMPLE();
case (byte) 0xa5:
return new IF_ACMPEQ();
case (byte) 0xa6:
return new IF_ACMPNE();
case (byte) 0xa7:
return new GOTO();
// case 0xa8:
// return &JSR{}
// case 0xa9:
// return &RET{}
case (byte) 0xaa:
return new TABLE_SWITCH();
case (byte) 0xab:
return new LOOKUP_SWITCH();
// case 0xac:
// return ireturn
// case 0xad:
// return lreturn
// case 0xae:
// return freturn
// case 0xaf:
// return dreturn
// case 0xb0:
// return areturn
// case 0xb1:
// return _return
// case 0xb2:
// return &GET_STATIC{}
// case 0xb3:
// return &PUT_STATIC{}
// case 0xb4:
// return &GET_FIELD{}
// case 0xb5:
// return &PUT_FIELD{}
// case 0xb6:
// return &INVOKE_VIRTUAL{}
// case 0xb7:
// return &INVOKE_SPECIAL{}
// case 0xb8:
// return &INVOKE_STATIC{}
// case 0xb9:
// return &INVOKE_INTERFACE{}
// case 0xba:
// return &INVOKE_DYNAMIC{}
// case 0xbb:
// return &NEW{}
// case 0xbc:
// return &NEW_ARRAY{}
// case 0xbd:
// return &ANEW_ARRAY{}
// case 0xbe:
// return arraylength
// case 0xbf:
// return athrow
// case 0xc0:
// return &CHECK_CAST{}
// case 0xc1:
// return &INSTANCE_OF{}
// case 0xc2:
// return monitorenter
// case 0xc3:
// return monitorexit
case (byte) 0xc4:
return new WIDE();
// case 0xc5:
// return &MULTI_ANEW_ARRAY{}
case (byte) 0xc6:
return new IFNULL();
case (byte) 0xc7:
return new IFNONNULL();
case (byte) 0xc8:
return new GOTO_W();
// case 0xc9:
// return &JSR_W{}
// case 0xca: breakpoint
// case 0xfe: impdep1
// case 0xff: impdep2
default:
return null;
}
}
}
Interpret.java
//指令集解释器
class Interpret {
Interpret(MemberInfo m) {
CodeAttribute codeAttr = m.codeAttribute();
int maxLocals = codeAttr.maxLocals();
int maxStack = codeAttr.maxStack();
byte[] byteCode = codeAttr.data();
Thread thread = new Thread();
Frame frame = thread.newFrame(maxLocals, maxStack);
thread.pushFrame(frame);
loop(thread, byteCode);
}
private void loop(Thread thread, byte[] byteCode) {
Frame frame = thread.popFrame();
BytecodeReader reader = new BytecodeReader();
while (true) {
//循环
int pc = frame.nextPC();
thread.setPC(pc);
//decode
reader.reset(byteCode, pc);
byte opcode = reader.readByte();
Instruction inst = Factory.newInstruction(opcode);
if (null == inst) {
System.out.println("寄存器(指令)尚未实现 " + byteToHexString(new byte[]{opcode}));
break;
}
inst.fetchOperands(reader);
frame.setNextPC(reader.pc());
System.out.println(“寄存器(指令):” + byteToHexString(new byte[]{opcode}) + " -> " + inst.getClass().getSimpleName() + " => 局部变量表:" + JSON.toJSONString(frame.operandStack().getSlots()) + " 操作数栈:" + JSON.toJSONString(frame.operandStack().getSlots())); //exec
inst.execute(frame);
}
}
private static String byteToHexString(byte[] codes) {
StringBuilder sb = new StringBuilder();
sb.append(“0x”);
for (byte b : codes) {
int value = b & 0xFF;
String strHex = Integer.toHexString(value);
if (strHex.length() < 2) {
strHex = “0” + strHex;
}
sb.append(strHex);
}
return sb.toString();
}
}
HelloWorld.java
package org.itstack.demo.test;
public class HelloWorld {
public static void main(String[] args) {
int sum = 0;
for (int i = 1; i <= 100; i++) {
sum += i;
}
System.out.println(sum);
}
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数Java工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
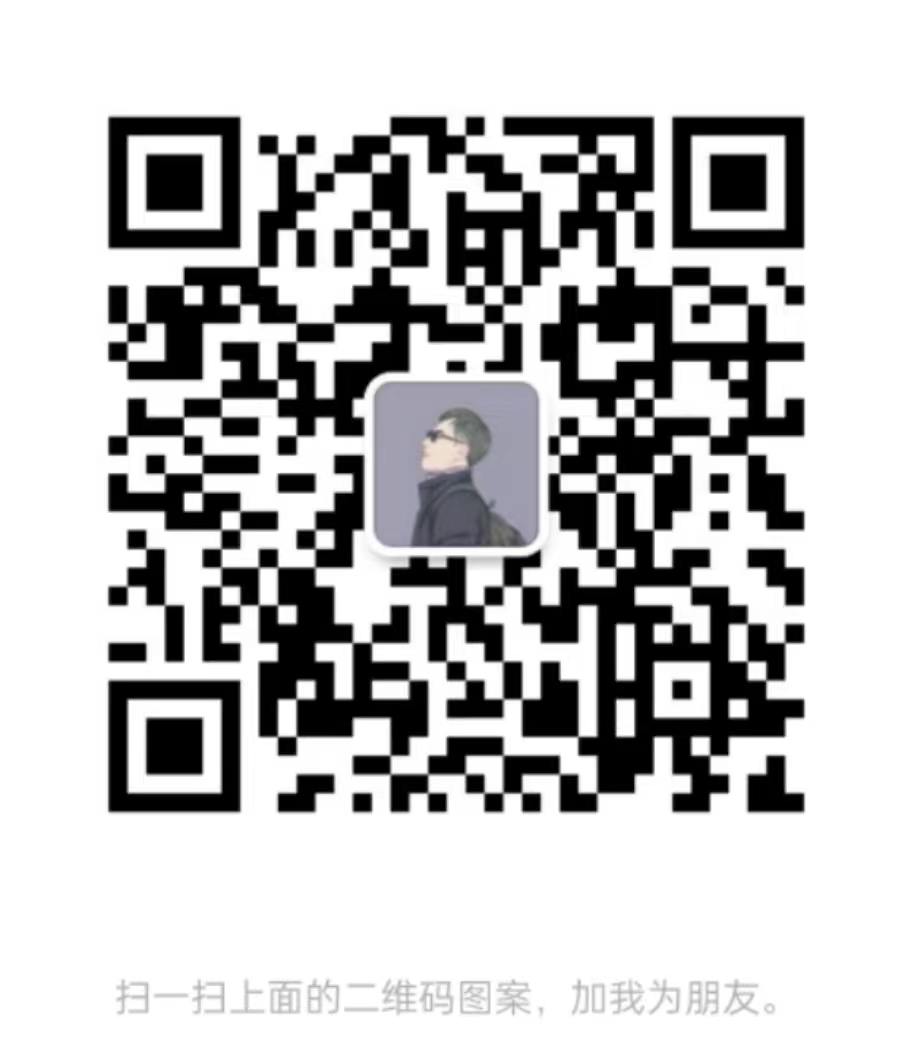
最后总结
搞定算法,面试字节再不怕,有需要文章中分享的这些二叉树、链表、字符串、栈和队列等等各大面试高频知识点及解析
最后再分享一份终极手撕架构的大礼包(学习笔记):分布式+微服务+开源框架+性能优化
《一线大厂Java面试题解析+核心总结学习笔记+最新讲解视频+实战项目源码》,点击传送门即可获取!
RhZhF-1712490261908)]
[外链图片转存中…(img-1fbjex56-1712490261908)]
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
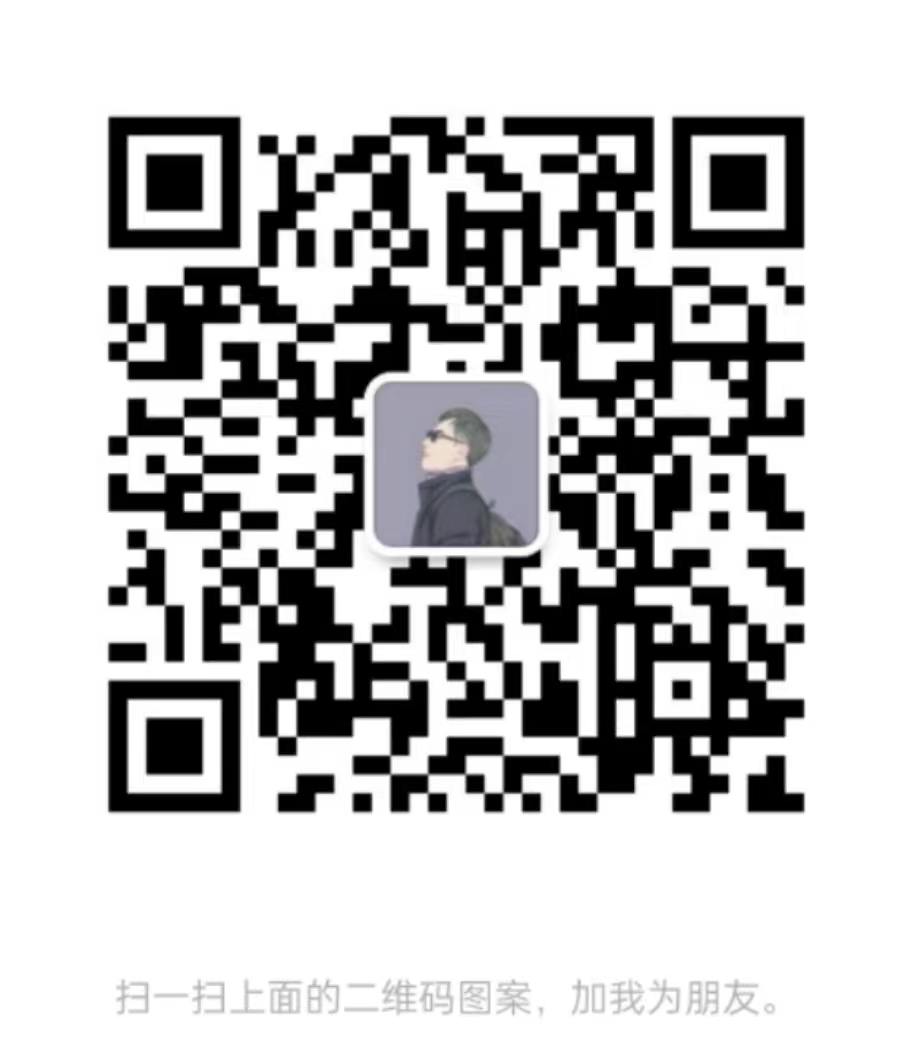
最后总结
搞定算法,面试字节再不怕,有需要文章中分享的这些二叉树、链表、字符串、栈和队列等等各大面试高频知识点及解析
最后再分享一份终极手撕架构的大礼包(学习笔记):分布式+微服务+开源框架+性能优化
[外链图片转存中…(img-GV2uCBJN-1712490261908)]
《一线大厂Java面试题解析+核心总结学习笔记+最新讲解视频+实战项目源码》,点击传送门即可获取!
更多推荐
所有评论(0)