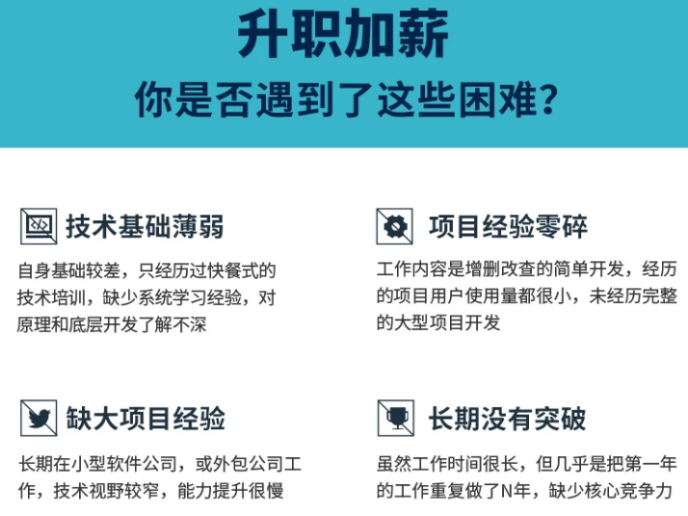
ble兼容Android 4 进行的部分优化,附架构师必备技术详解
点击我的GitHub免费领取获取往期Android高级架构资料、源码、笔记、视频。高级UI、性能优化、架构师课程、NDK、混合式开发(ReactNative+Weex)微信小程序、Flutter全方面的Android进阶实践技术,群内还有技术大牛一起讨论交流解决问题。[外链图片转存中…(img-4IGfJ3tl-1711179273469)]由于文件比较大,这里只是将部分目录截图出来,每个节点里面

1.获取BluetoothManager服务及BluetoothAdapter实例
BluetoothManager是系统管理蓝牙的一种服务,获取了BluetoothManager我们就可以通过其getAdapter()获取蓝牙适配器了。
[java] view plain copy
-
//1.获取蓝牙管理服务及BluetoothAdapter实例
-
mBluetoothManager = (BluetoothManager)getSystemService(Context.BLUETOOTH_SERVICE);
-
mBluetoothAdapter = mBluetoothManager.getAdapter();
-
if (mBluetoothAdapter == null){
-
return;
-
}
//1.获取蓝牙管理服务及BluetoothAdapter实例
mBluetoothManager = (BluetoothManager)getSystemService(Context.BLUETOOTH_SERVICE);
mBluetoothAdapter = mBluetoothManager.getAdapter();
if (mBluetoothAdapter == null){
return;
}
2.打开蓝牙
接下来调用BluetoothAdapter的isEnabled()判断蓝牙是否打开,未打开我们可以发送一个action为BluetoothAdapter.ACTION_REQUEST_ENABLE的intent来打开蓝牙。
[java] view plain copy
-
//2. 判断蓝牙是否打开,没有打开就打开
-
if (!mBluetoothAdapter.isEnabled()){
-
Intent intent = newIntent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
-
startActivityForResult(intent,REQUEST_ENABLE_BT);
-
}
//2. 判断蓝牙是否打开,没有打开就打开
if (!mBluetoothAdapter.isEnabled()){
Intent intent = newIntent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(intent,REQUEST_ENABLE_BT);
}
这时界面上回弹出一个对话框提示我们打开蓝牙,打开成功后会回调Activity的onActivityResult(int requestCode, int resultCode, Intent data)方法,我们可以在里面提示一些信息。比如提示蓝牙已经打开等。
[java] view plain copy
-
@Override
-
protected void onActivityResult(int requestCode, int resultCode, Intentdata) {
-
super.onActivityResult(requestCode, resultCode, data);
-
if (resultCode != RESULT_OK){
-
return;
-
}
-
if (requestCode == REQUEST_ENABLE_BT){
-
Toast.makeText(this,“蓝牙已开启”,Toast.LENGTH_LONG).show();
-
}
-
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intentdata) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode != RESULT_OK){
return;
}
if (requestCode == REQUEST_ENABLE_BT){
Toast.makeText(this,“蓝牙已开启”,Toast.LENGTH_LONG).show();
}
}
3.扫描周围的BLE蓝牙设备
扫描BLE蓝牙设备,对于4.3以上的系统,直接调用startLeScan(BluetoothAdapter.LeScanCallbackcallback)即可扫描出BLE设备,在callback中会回调。但是对于5.0以上的系统,android添加了新的API,原有的startLeScan(BluetoothAdapter.LeScanCallback callback)已经被废弃,在5.0以上的系统中是使用BluetoothLeScanner的startScan(ScanCallbackcallback),回调也是ScanCallback了。
[java] view plain copy
-
/**
-
* 扫描Bluetooth LE
-
* @param enable
-
*/
-
private void scanBleDevice(boolean enable){
-
//android 5.0 以前
-
if (Build.VERSION.SDK_INT < 21){
-
if (enable){
-
mHandler.postDelayed(new Runnable() {
-
@Override
-
public void run() {
-
mScaning = false;
-
mBluetoothAdapter.stopLeScan(mLeScanCallback);
-
}
-
},SCAN_SECOND);
-
mScaning = true;
-
mBluetoothAdapter.startLeScan(mLeScanCallback);
-
} else {
-
mScaning = false;
-
mBluetoothAdapter.stopLeScan(mLeScanCallback);
-
}
-
} else {
-
scanner = mBluetoothAdapter.getBluetoothLeScanner();
-
scanner.startScan(mScanCallback);
-
mHandler.postDelayed(new Runnable() {
-
@Override
-
public void run() {
-
scanner.stopScan(mScanCallback);
-
}
-
},SCAN_SECOND);
-
}
-
}
/**
-
扫描Bluetooth LE
-
@param enable
*/
private void scanBleDevice(boolean enable){
//android 5.0 以前
if (Build.VERSION.SDK_INT < 21){
if (enable){
mHandler.postDelayed(new Runnable() {
@Override
public void run() {
mScaning = false;
mBluetoothAdapter.stopLeScan(mLeScanCallback);
}
},SCAN_SECOND);
mScaning = true;
mBluetoothAdapter.startLeScan(mLeScanCallback);
} else {
mScaning = false;
mBluetoothAdapter.stopLeScan(mLeScanCallback);
}
} else {
scanner = mBluetoothAdapter.getBluetoothLeScanner();
scanner.startScan(mScanCallback);
mHandler.postDelayed(new Runnable() {
@Override
public void run() {
scanner.stopScan(mScanCallback);
}
},SCAN_SECOND);
}
}
扫描的回调如下:
[java] view plain copy
-
//sacn扫描回调 5.0以上用
-
private ScanCallback mScanCallback = new ScanCallback() {
-
@Override
-
public void onScanResult(int callbackType, ScanResult result) {
-
BluetoothDevice device =result.getDevice();
-
if (device != null){
-
//过滤掉其他设备
-
if (device.getName() != null&& device.getName().startsWith(“WINPOS”)){
-
BLEDevice bleDevice = newBLEDevice(device.getName(),device.getAddress());
-
if(!mBLEDeviceList.contains(bleDevice)){
-
mBLEDeviceList.add(bleDevice);
-
showBluetoothLeDevice(bleDevice);
-
}
-
}
-
}
-
}
-
@Override
-
public void onBatchScanResults(List results) {
-
Log.d(TAG,”onBatchScanResults”);
-
}
-
@Override
-
public void onScanFailed(int errorCode) {
-
Log.d(TAG,”onScanFailed”);
-
}
-
};
-
//4.3以上
-
private BluetoothAdapter.LeScanCallback mLeScanCallback = newBluetoothAdapter.LeScanCallback() {
-
@Override
-
public void onLeScan(final BluetoothDevice bluetoothDevice, int i,byte[] bytes) {
-
if (bluetoothDevice != null){
-
//过滤掉其他设备
-
if (bluetoothDevice.getName()!= null && bluetoothDevice.getName().startsWith(“WINPOS”)){
-
BLEDevice bleDevice = newBLEDevice(bluetoothDevice.getName(),bluetoothDevice.getAddress());
-
if(!mBLEDeviceList.contains(bleDevice)){
-
mBLEDeviceList.add(bleDevice);
-
showBluetoothLeDevice(bleDevice);
-
}
-
}
-
}
-
}
-
};
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数初中级安卓工程师,想要提升技能,往往是自己摸索成长,但自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年最新Android移动开发全套学习资料》送给大家,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频
如果你觉得这些内容对你有帮助,可以添加下面V无偿领取!(备注Android)
最后相关架构及资料领取方式:
点击我的GitHub免费领取获取往期Android高级架构资料、源码、笔记、视频。高级UI、性能优化、架构师课程、NDK、混合式开发(ReactNative+Weex)微信小程序、Flutter全方面的Android进阶实践技术,群内还有技术大牛一起讨论交流解决问题。
…(img-VdJQnTMD-1711179273469)]
[外链图片转存中…(img-4IGfJ3tl-1711179273469)]
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频
如果你觉得这些内容对你有帮助,可以添加下面V无偿领取!(备注Android)
[外链图片转存中…(img-ZCbzXdVW-1711179273470)]
最后相关架构及资料领取方式:
点击我的GitHub免费领取获取往期Android高级架构资料、源码、笔记、视频。高级UI、性能优化、架构师课程、NDK、混合式开发(ReactNative+Weex)微信小程序、Flutter全方面的Android进阶实践技术,群内还有技术大牛一起讨论交流解决问题。
更多推荐
所有评论(0)