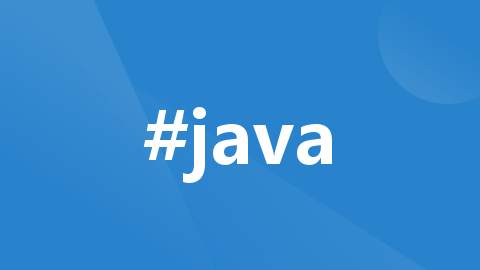
NoSuchMethodException(方法未找到异常) 常出现的原因和解决步骤
NoSuchMethodException
表示在尝试反射调用一个不存在的方法时抛出的异常。以下是 NoSuchMethodException
常见的原因和解决步骤:
常见原因:
-
方法名错误: 尝试调用的方法名与目标类中的方法名不匹配。
javaCopy code
class MyClass { public void myMethod() { // some code } } MyClass obj = new MyClass(); Method method = obj.getClass().getMethod("nonexistentMethod");
解决步骤: 确保方法名的拼写和大小写与目标类中的方法名一致。
javaCopy code
Method method = obj.getClass().getMethod("myMethod");
-
方法参数类型不匹配: 尝试调用的方法存在,但其参数类型与反射中的
getMethod
方法中提供的参数类型不匹配。javaCopy code
class MyClass { public void myMethod(int number) { // some code } } MyClass obj = new MyClass(); Method method = obj.getClass().getMethod("myMethod", String.class);
解决步骤: 确保
getMethod
中提供的参数类型与目标方法的参数类型一致。javaCopy code
Method method = obj.getClass().getMethod("myMethod", int.class);
-
方法不存在: 尝试调用的方法在目标类中不存在。
javaCopy code
class MyClass { // method is missing } MyClass obj = new MyClass(); Method method = obj.getClass().getMethod("nonexistentMethod");
解决步骤: 确保调用的方法确实存在于目标类中。
javaCopy code
class MyClass { public void nonexistentMethod() { // some code } } MyClass obj = new MyClass(); Method method = obj.getClass().getMethod("nonexistentMethod");
预防措施:
-
使用更安全的方法调用: 在反射调用方法之前,可以先检查目标类中是否存在该方法,以避免在运行时抛出
NoSuchMethodException
。javaCopy code
Class<?> clazz = obj.getClass(); try { Method method = clazz.getMethod("myMethod", int.class); // 执行反射调用 } catch (NoSuchMethodException e) { // 处理异常,方法不存在 e.printStackTrace(); }
-
使用更具体的反射方法: 使用
getDeclaredMethod
而不是getMethod
,前者可以获取所有访问级别的方法,而后者只能获取public
方法。javaCopy code
Class<?> clazz = obj.getClass(); try { Method method = clazz.getDeclaredMethod("myMethod", int.class); // 执行反射调用 } catch (NoSuchMethodException e) { // 处理异常,方法不存在 e.printStackTrace(); }
-
使用参数类型数组: 在获取方法时,可以使用参数类型数组,以确保参数类型的匹配。
javaCopy code
Class<?>[] parameterTypes = {int.class}; try { Method method = clazz.getMethod("myMethod", parameterTypes); // 执行反射调用 } catch (NoSuchMethodException e) { // 处理异常,方法不存在 e.printStackTrace(); }
通过采取这些预防措施,可以降低 NoSuchMethodException
的发生概率,提高代码的健壮性。
更多推荐
所有评论(0)