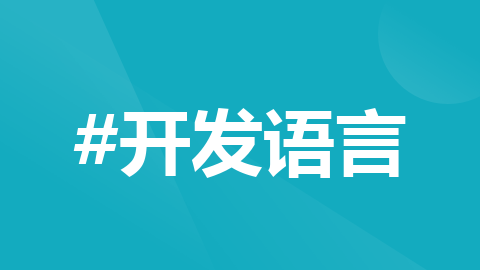
SocketException(Socket异常)可能的原因和解决方法
时,适当地检查异常的类型,并采取适当的处理措施。详细的错误日志和异常堆栈信息对于定位和解决问题非常有帮助。时,适当地检查异常的类型,并采取适当的处理措施。详细的错误日志和异常堆栈信息对于定位和解决问题非常有帮助。表示在使用套接字时可能发生的异常。

SocketException
表示在使用套接字时可能发生的异常。以下是可能导致 SocketException
的一些原因以及相应的解决方法:
-
连接被拒绝(Connection Refused):
- 可能原因: 尝试连接到远程主机的套接字,但远程主机拒绝了连接。
- 解决方法: 确保远程主机正在运行,并且目标端口处于监听状态。检查网络连接和防火墙设置,确保允许连接到目标主机的指定端口。
javaCopy code
try (Socket socket = new Socket("example.com", 8080)) { // Perform socket operations } catch (SocketException e) { e.printStackTrace(); // Handle connection refused issue } catch (IOException e) { e.printStackTrace(); // Handle other IO exceptions }
-
连接超时:
- 可能原因: 尝试连接到远程主机的套接字,但连接超时。
- 解决方法: 增加连接的超时时间,以便等待更长的时间,或者检查网络连接,确保网络正常。
javaCopy code
try (Socket socket = new Socket()) { socket.connect(new InetSocketAddress("example.com", 8080), 5000); // 5000 milliseconds timeout // Perform socket operations } catch (SocketException e) { e.printStackTrace(); // Handle connection timeout issue } catch (IOException e) { e.printStackTrace(); // Handle other IO exceptions }
-
网络不可达:
- 可能原因: 尝试连接到远程主机的套接字,但网络不可达。
- 解决方法: 检查网络连接,确保网络可达。检查目标主机是否正确配置,并检查防火墙设置。
javaCopy code
try (Socket socket = new Socket("example.com", 8080)) { // Perform socket operations } catch (SocketException e) { e.printStackTrace(); // Handle network unreachable issue } catch (IOException e) { e.printStackTrace(); // Handle other IO exceptions }
-
套接字关闭:
- 可能原因: 尝试在已关闭的套接字上执行操作。
- 解决方法: 在执行套接字操作之前,确保套接字处于打开状态。
javaCopy code
try (Socket socket = new Socket("example.com", 8080)) { // Perform socket operations } catch (SocketException e) { if (e.getMessage().equals("Socket closed")) { // Handle closed socket issue } else { e.printStackTrace(); // Handle other socket exceptions } } catch (IOException e) { e.printStackTrace(); // Handle other IO exceptions }
-
无法绑定地址或端口:
- 可能原因: 尝试在已被其他套接字占用的地址或端口上绑定套接字。
- 解决方法: 使用一个未被占用的地址和端口,或者等待其他套接字释放该地址和端口。
javaCopy code
try (ServerSocket serverSocket = new ServerSocket(8080)) { // Perform server socket operations } catch (SocketException e) { e.printStackTrace(); // Handle bind address/port issue } catch (IOException e) { e.printStackTrace(); // Handle other IO exceptions }
-
Socket关闭后继续使用:
- 可能原因: 在关闭套接字后尝试继续使用它。
- 解决方法: 在关闭套接字后,不要再尝试使用它。如果需要再次进行通信,创建一个新的套接字。
javaCopy code
Socket socket = new Socket("example.com", 8080); socket.close(); // Don't perform operations on the closed socket // Instead, create a new socket for further communication
确保在处理 SocketException
时,适当地检查异常的类型,并采取适当的处理措施。详细的错误日志和异常堆栈信息对于定位和解决问题非常有帮助。
-
TCP Reset(RST):
- 可能原因: 在尝试与远程主机通信时,收到 TCP Reset(RST)信号,表示连接被远程主机中止。
- 解决方法: 可能是远程主机意外关闭了连接,或者存在网络问题。检查网络连接、防火墙设置,确保远程主机正常运行。
javaCopy code
try (Socket socket = new Socket("example.com", 8080)) { // Perform socket operations } catch (SocketException e) { if (e.getMessage().contains("Connection reset")) { // Handle connection reset issue } else { e.printStackTrace(); // Handle other socket exceptions } } catch (IOException e) { e.printStackTrace(); // Handle other IO exceptions }
-
多次绑定同一端口:
- 可能原因: 尝试在同一端口上多次绑定套接字。
- 解决方法: 确保套接字在绑定之前是未绑定的。在关闭套接字后,如果需要再次使用相同的端口,请确保先解除绑定。
javaCopy code
ServerSocket serverSocket = new ServerSocket(8080); // Perform server socket operations serverSocket.close(); // Close the server socket // Perform other operations // If needed to bind again to the same port, create a new ServerSocket ServerSocket newServerSocket = new ServerSocket(8080); // Perform new server socket operations
-
套接字操作超时:
- 可能原因: 在进行套接字操作时,操作超时。
- 解决方法: 增加适当的超时时间,以便等待更长的时间,或检查网络连接,确保网络正常。
javaCopy code
try (Socket socket = new Socket("example.com", 8080)) { socket.setSoTimeout(5000); // 5000 milliseconds timeout for socket operations // Perform socket operations } catch (SocketTimeoutException e) { e.printStackTrace(); // Handle socket operation timeout issue } catch (SocketException e) { e.printStackTrace(); // Handle other socket exceptions } catch (IOException e) { e.printStackTrace(); // Handle other IO exceptions }
-
关闭输入流或输出流导致 Socket 关闭:
- 可能原因: 在关闭套接字关联的输入流或输出流时,导致套接字关闭。
- 解决方法: 在关闭输入流或输出流之前,确保不会关闭底层的套接字。
javaCopy code
try (Socket socket = new Socket("example.com", 8080); OutputStream outputStream = socket.getOutputStream()) { // Perform operations with the output stream // Don't close the socket here, only close the output stream outputStream.close(); } catch (SocketException e) { e.printStackTrace(); // Handle socket-related issues } catch (IOException e) { e.printStackTrace(); // Handle other IO exceptions }
-
IPv6和IPv4冲突:
- 可能原因: 在使用套接字时,IPv6和IPv4配置存在冲突。
- 解决方法: 明确指定使用IPv4或IPv6。可以通过使用
Inet4Address
或Inet6Address
的相应方法来指定地址。
javaCopy code
try (Socket socket = new Socket(Inet4Address.getByName("example.com"), 8080)) { // Perform socket operations } catch (SocketException e) { e.printStackTrace(); // Handle socket-related issues } catch (IOException e) { e.printStackTrace(); // Handle other IO exceptions }
确保在处理 SocketException
时,适当地检查异常的类型,并采取适当的处理措施。详细的错误日志和异常堆栈信息对于定位和解决问题非常有帮助
更多推荐
所有评论(0)