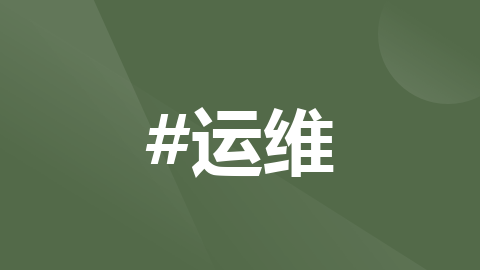
ClassCastException(类转换异常)可能原因和解决方法
运算符进行类型检查,避免直接进行不安全的强制类型转换。此外,注意在泛型代码中使用泛型的参数类型,以避免由于类型擦除而导致的问题。在使用反射时,确保进行类型转换的对象与目标类型是兼容的。(类转换异常)通常发生在试图将一个对象强制转换成它不是实际类型的类型时。这个异常表明程序在运行时遇到了类型不兼容的强制转换。时,关键是了解为什么发生了类型不匹配,然后采取相应的措施确保类型转换是安全的。如果有疑虑,可

ClassCastException
(类转换异常)通常发生在试图将一个对象强制转换成它不是实际类型的类型时。这个异常表明程序在运行时遇到了类型不兼容的强制转换。以下是可能导致ClassCastException
异常的一些常见原因和解决方法:
-
错误的强制类型转换:
- 可能原因: 尝试将一个对象强制转换为它不是实际类型的类型。
- 解决方法: 在进行强制类型转换之前,先使用
instanceof
运算符检查对象的实际类型,以确保转换是安全的。
javaCopy code
Object obj = // some object; // Incorrect: assuming obj is of type String String str = (String) obj; // This may throw ClassCastException // Correct: checking the type before casting if (obj instanceof String) { String str = (String) obj; // Continue with operations on str } else { // Handle the case when obj is not of type String }
-
泛型类型转换问题:
- 可能原因: 在泛型代码中,由于类型擦除,尝试将一个对象转换为其泛型参数类型时发生问题。
- 解决方法: 避免在泛型代码中进行不安全的类型转换。如果必须进行转换,请使用带有泛型参数的构造函数或方法,并确保进行合适的类型检查。
javaCopy code
// Incorrect: unsafe type cast in generic code List<Integer> integerList = (List<Integer>) someObject; // This may throw ClassCastException // Correct: using a constructor or method with appropriate type check List<Integer> integerList = convertToIntegerList(someObject);
-
对象序列化和反序列化问题:
- 可能原因: 在对象进行序列化和反序列化时,类型信息可能会丢失,导致在反序列化时发生
ClassCastException
。 - 解决方法: 在进行反序列化时,确保类型信息得以保留,以便正确地还原对象。
javaCopy code
// Incorrect: deserialization without type information Object deserializedObject = deserialize(someSerializedData); // This may throw ClassCastException // Correct: deserialization with type information SomeClass deserializedObject = deserializeWithType(someSerializedData, SomeClass.class);
- 可能原因: 在对象进行序列化和反序列化时,类型信息可能会丢失,导致在反序列化时发生
-
使用原始类型转换:
- 可能原因: 在使用泛型时,尝试将泛型类型转换为原始类型。
- 解决方法: 在使用泛型时,尽量避免使用原始类型,而是使用泛型的参数类型。
javaCopy code
// Incorrect: using raw type List list = (List) someObject; // This may throw ClassCastException // Correct: using parameterized type List<String> stringList = (List<String>) someObject;
-
Class Loader问题:
- 可能原因: 类加载器加载了两个不同的类,即使它们的名称相同,也可能导致
ClassCastException
。 - 解决方法: 确保类加载器加载的类是同一个,或者考虑使用相同的类加载器加载这两个类。
javaCopy code
ClassLoader classLoader1 = // some class loader; ClassLoader classLoader2 = // another class loader; Class<?> clazz1 = classLoader1.loadClass("com.example.MyClass"); Class<?> clazz2 = classLoader2.loadClass("com.example.MyClass"); // Incorrect: objects of clazz1 and clazz2 are not assignable MyClass obj1 = (MyClass) clazz1.newInstance(); // This may throw ClassCastException MyClass obj2 = (MyClass) clazz2.newInstance(); // This may throw ClassCastException // Correct: ensure both classes are loaded by the same class loader ClassLoader commonClassLoader = // a common class loader; Class<?> commonClazz1 = commonClassLoader.loadClass("com.example.MyClass"); Class<?> commonClazz2 = commonClassLoader.loadClass("com.example.MyClass"); MyClass commonObj1 = (MyClass) commonClazz1.newInstance(); // This is safe MyClass commonObj2 = (MyClass) commonClazz2.newInstance(); // This is safe
- 可能原因: 类加载器加载了两个不同的类,即使它们的名称相同,也可能导致
在处理ClassCastException
时,关键是了解为什么发生了类型不匹配,然后采取相应的措施确保类型转换是安全的。如果有疑虑,可以使用instanceof
运算符进行类型检查,并根据需要进行处理。
-
基本数据类型的包装类问题:
- 可能原因: 在使用基本数据类型的包装类时,可能会发生不同包装类之间的转换问题。
- 解决方法: 使用适当的方法将基本数据类型的包装类转换为另一个包装类,而不是直接进行强制类型转换。
javaCopy code
Integer intValue = 42; // Incorrect: direct type cast from Integer to Long Long longValue = (Long) intValue; // This may throw ClassCastException // Correct: using appropriate conversion method Long longValueCorrect = intValue.longValue(); // This is safe
-
在同一继承层次内的不兼容类型:
- 可能原因: 尝试将同一继承层次内的不兼容类型进行强制转换。
- 解决方法: 确保进行类型转换的对象实际上是目标类型或其子类型。
javaCopy code
class Animal {} class Dog extends Animal {} Animal animal = new Animal(); // Incorrect: trying to cast Animal to Dog Dog dog = (Dog) animal; // This may throw ClassCastException // Correct: ensure the object is actually a Dog if (animal instanceof Dog) { Dog dogCorrect = (Dog) animal; // This is safe }
-
使用反射进行类型转换:
- 可能原因: 在使用反射时,尝试将一个对象转换为与其实际类型不兼容的类型。
- 解决方法: 在使用反射进行类型转换时,确保转换是合理的,否则会导致
ClassCastException
。
javaCopy code
Class<?> targetClass = // target class; Object obj = // some object; // Incorrect: trying to cast obj to targetClass Object castObject = targetClass.cast(obj); // This may throw ClassCastException // Correct: ensure obj is assignable to targetClass if (targetClass.isInstance(obj)) { Object castObjectCorrect = targetClass.cast(obj); // This is safe }
总体而言,要避免ClassCastException
,首先要理解对象的实际类型。使用instanceof
运算符进行类型检查,避免直接进行不安全的强制类型转换。此外,注意在泛型代码中使用泛型的参数类型,以避免由于类型擦除而导致的问题。在使用反射时,确保进行类型转换的对象与目标类型是兼容的。
更多推荐
所有评论(0)