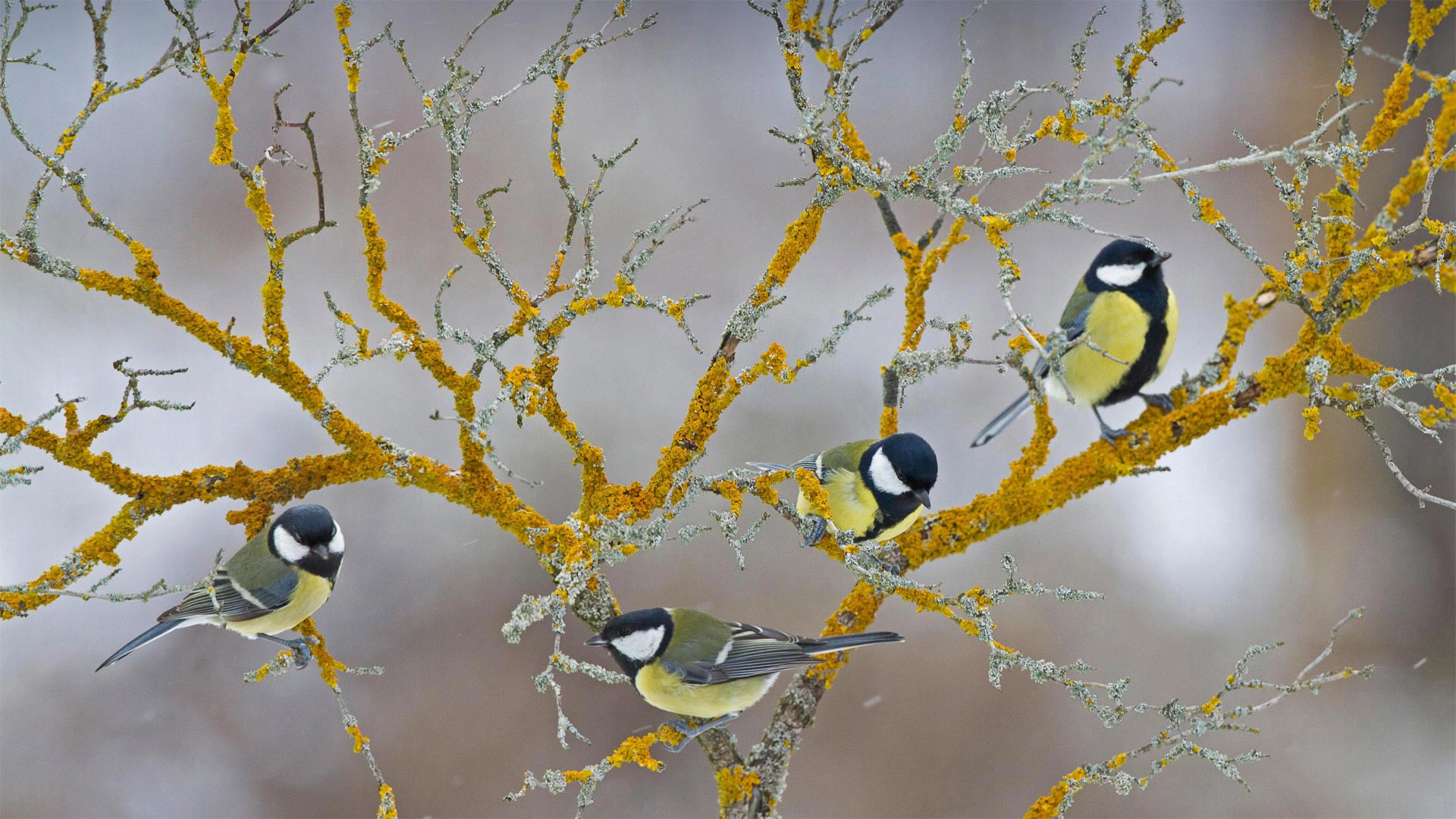
Qt | QTableView的使用方法
·
Qt | QTableView的使用方法
QTableView的数据是基于model显示的,需要先建一个model,然后tableView通过setModel进行模型绑定,后续操作model就可以实现表格数据的读写。
1、基础使用
1、设置模型显示
# 创建3X3模型,如果不指定表格大小,那么他会根据标签的数量自行定义表格大小
model = QStandardItemModel(3, 3, self.__MainWindow)
# 设置行标签
model.setHorizontalHeaderLabels(['编号', '姓名', '年龄'])
# 设置列标签
model.setVerticalHeaderLabels(['一班', '二班', '三班'])
# 设置模型到TabelView上
self.tableView.setModel(model)
2、设置内容显示
data = ['1', '2', '3']
for i in range(len(data)):
item = QStandardItem(data[i])
# 设置内容对齐方式为:水平中心对齐+垂直中心对齐
item.setTextAlignment(Qt.AlignHCenter | Qt.AlignVCenter)
model.setItem(i, i, item)
3、获取表格内容
QString data = model->data(model->index(0, 0)).toString(); // 获取第[0,0]格的数据
4、设置表格之间根据间距调整宽度方式
一共5种显示模式:
- Custom
- Fixed
- Interactive
- ResizeToContents
- Stretch
常用的是根据内容自动调整大小和拉伸
设置方法:
# 设置水平标签重载大小模式为拉伸,也就是铺满整个框,每一列间距是一样的,这样比较好看
self.tableView.horizontalHeader().setSectionResizeMode(QHeaderView.Stretch)
5、隐藏标题栏
tableView->verticalHeader()->hide();
2、表格设置成下拉框形式
QTableView的表格默认显示的是ItemDelegate,双击表格的时候可以实现编辑,如果想要实现双机表格出现一个下拉框,那么就需要将Delegate设置为QComboBox,实现办法是自定义继承自QItemDelegate的ComboDelegate,覆写updateEditorGeometry、setModelData、setEditorData、createEditor这四个函数即可。代码如下:
头文件:
#ifndef COMBODELEGATE_H
#define COMBODELEGATE_H
#include <QItemDelegate>
class ComboDelegate : public QItemDelegate
{
Q_OBJECT
public:
ComboDelegate() = delete;
virtual ~ComboDelegate();
ComboDelegate(const QStringList &items, QObject *parent = 0);
QWidget *createEditor(QWidget *parent, const QStyleOptionViewItem &option, const QModelIndex &index) const;
void setEditorData(QWidget *editor, const QModelIndex &index) const;
void setModelData(QWidget *editor, QAbstractItemModel *model, const QModelIndex &index) const;
void updateEditorGeometry(QWidget *editor, const QStyleOptionViewItem &option, const QModelIndex &index) const;
signals:
void currentIndexChange(int row, int col) const; //定义消息必须有const
private slots :
private:
QStringList items;
};
#endif // COMBODELEGATE_H
源文件:
#include "ComboDelegate.h"
#include <QtWidgets/QComboBox>
#include <QDebug>
ComboDelegate::ComboDelegate(const QStringList &items, QObject *parent) : QItemDelegate(parent)
{
this->items = items;
}
ComboDelegate::~ComboDelegate()
{
}
QWidget *ComboDelegate::createEditor(QWidget *parent, const QStyleOptionViewItem &, const QModelIndex &) const
{
QComboBox *editor = new QComboBox(parent);
editor->addItems(items);
editor->setEditable(false); // 不可编辑
return editor;
}
void ComboDelegate::setEditorData(QWidget *editor, const QModelIndex &index) const
{
QString value = index.model()->data(index, Qt::EditRole).toString();
QComboBox *comboBox = static_cast<QComboBox*>(editor);
int currIndex = comboBox->findText(value);
comboBox->setCurrentIndex(currIndex);
}
void ComboDelegate::setModelData(QWidget *editor, QAbstractItemModel *model, const QModelIndex &index) const
{
QComboBox *comboBox = static_cast<QComboBox*>(editor);
QString value = comboBox->currentText();
model->setData(index, value, Qt::EditRole);
emit currentIndexChange(index.row(), index.column()); // 发送信号,实现其它联动更新
}
void ComboDelegate::updateEditorGeometry(QWidget *editor,const QStyleOptionViewItem &option, const QModelIndex &index) const
{
(void)index; // 防止编译警告
editor->setGeometry(option.rect);
QComboBox *comboBox = static_cast<QComboBox*>(editor);
comboBox->showPopup(); // 直接弹出下拉框,没有这一句双击后需要再点一次Combox才会弹出下拉框
}
使用方法:
QStringList sensorTypes;
sensorTypes << ""
<< "颗粒物"
<< "火灾"
<< "烟感"
<< "水浸"
<< "温度"
<< "湿度"
<< "温湿度"
<< "自定义1"
<< "自定义2"
<< "自定义3"
<< "自定义4"
<< "自定义5"
<< "自定义6"
<< "自定义7"
<< "自定义8"
<< "自定义9"
<< "自定义10";
ComboDelegate* delegateSensorTypes = new ComboDelegate(sensorTypes, this);
ui->tableView->setItemDelegateForColumn(0, delegateSensorTypes); // 第0列表格设置为下拉框形式
效果图:
ends…
更多推荐
所有评论(0)