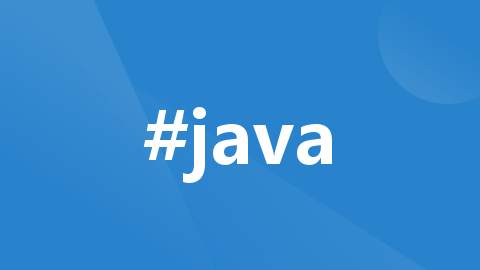
TimeoutException(超时异常)可能的原因和解决方法
TimeoutException
通常表示一个操作在规定的时间内没有完成。以下是可能导致 TimeoutException
的一些常见原因以及相应的解决方法:
-
网络连接超时:
- 可能原因: 尝试与远程主机建立网络连接时,连接超过了指定的时间。
- 解决方法: 增加连接的超时时间,以便等待更长的时间。检查网络连接,确保网络正常。
javaCopy code
try (Socket socket = new Socket()) { socket.connect(new InetSocketAddress("example.com", 8080), 5000); // 5000 milliseconds timeout // Perform socket operations } catch (TimeoutException e) { e.printStackTrace(); // Handle connection timeout issue } catch (IOException e) { e.printStackTrace(); // Handle other IO exceptions }
-
等待任务完成超时:
- 可能原因: 在多线程或并发编程中,等待某个任务的完成超过了规定的时间。
- 解决方法: 使用合适的同步机制,确保线程能够及时完成任务。可以考虑使用
ExecutorService
和Future
进行任务执行和等待。
javaCopy code
ExecutorService executorService = Executors.newSingleThreadExecutor(); try { Future<String> future = executorService.submit(() -> { // Perform some time-consuming task return "Task result"; }); String result = future.get(5000, TimeUnit.MILLISECONDS); // 5000 milliseconds timeout // Process the result } catch (TimeoutException e) { e.printStackTrace(); // Handle task timeout issue } catch (InterruptedException | ExecutionException e) { e.printStackTrace(); // Handle other exceptions } finally { executorService.shutdown(); }
-
等待资源或锁超时:
- 可能原因: 在并发编程中,等待获取某个资源或锁的操作超过了规定的时间。
- 解决方法: 使用适当的同步机制,确保能够及时获取所需的资源或锁。可以使用
ReentrantLock
等。
javaCopy code
ReentrantLock lock = new ReentrantLock(); try { if (lock.tryLock(5000, TimeUnit.MILLISECONDS)) { // 5000 milliseconds timeout try { // Perform operations requiring the lock } finally { lock.unlock(); } } else { // Handle inability to acquire the lock within the timeout } } catch (InterruptedException e) { e.printStackTrace(); // Handle interrupted exception }
-
等待IO操作完成超时:
- 可能原因: 在进行某些IO操作(如读取或写入)时,操作超过了规定的时间。
- 解决方法: 设置适当的超时时间,或使用非阻塞IO。对于阻塞IO,可以使用
Socket.setSoTimeout()
等。
javaCopy code
try (Socket socket = new Socket()) { socket.setSoTimeout(5000); // 5000 milliseconds timeout // Perform socket IO operations } catch (TimeoutException e) { e.printStackTrace(); // Handle IO timeout issue } catch (IOException e) { e.printStackTrace(); // Handle other IO exceptions }
-
数据库操作超时:
- 可能原因: 在进行数据库操作时,操作超过了规定的时间。
- 解决方法: 针对数据库连接、查询或事务等设置适当的超时时间。使用数据库连接池时,检查连接池配置。
javaCopy code
try (Connection connection = DriverManager.getConnection("jdbc:mysql://example.com:3306/mydatabase", "user", "password")) { Statement statement = connection.createStatement(); statement.setQueryTimeout(5); // 5 seconds query timeout // Perform database operations } catch (TimeoutException e) { e.printStackTrace(); // Handle database operation timeout issue } catch (SQLException e) { e.printStackTrace(); // Handle other SQL exceptions }
确保在处理 TimeoutException
时,适当地检查异常的类型,并采取适当的处理措施。详细的错误日志和异常堆栈信息对于定位和解决问题非常有帮助。
-
Web请求超时:
- 可能原因: 在进行Web请求时,等待响应的时间超过了规定的时间。
- 解决方法: 设置适当的超时时间,使用异步请求或者考虑实现超时机制。
javaCopy code
RequestConfig requestConfig = RequestConfig.custom() .setSocketTimeout(5000) // 5000 milliseconds timeout for socket .setConnectTimeout(5000) // 5000 milliseconds timeout for connection .build(); CloseableHttpClient httpClient = HttpClients.custom() .setDefaultRequestConfig(requestConfig) .build(); HttpGet httpGet = new HttpGet("http://example.com/resource"); try (CloseableHttpResponse response = httpClient.execute(httpGet)) { // Perform operations with the response } catch (TimeoutException e) { e.printStackTrace(); // Handle web request timeout issue } catch (IOException e) { e.printStackTrace(); // Handle other IO exceptions }
-
异步操作超时:
- 可能原因: 在进行异步操作时,等待结果的时间超过了规定的时间。
- 解决方法: 设置适当的超时时间,使用
CompletableFuture
或类似的异步机制,并在获取结果时设定超时。
javaCopy code
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> { // Perform asynchronous task return "Task result"; }); try { String result = future.get(5000, TimeUnit.MILLISECONDS); // 5000 milliseconds timeout // Process the result } catch (TimeoutException e) { e.printStackTrace(); // Handle asynchronous operation timeout issue } catch (InterruptedException | ExecutionException e) { e.printStackTrace(); // Handle other exceptions }
-
JDBC查询超时:
- 可能原因: 在进行 JDBC 查询时,等待查询结果的时间超过了规定的时间。
- 解决方法: 设置适当的查询超时时间,使用
Statement.setQueryTimeout()
。
javaCopy code
try (Connection connection = DriverManager.getConnection("jdbc:mysql://example.com:3306/mydatabase", "user", "password")) { Statement statement = connection.createStatement(); statement.setQueryTimeout(5); // 5 seconds query timeout // Perform JDBC operations } catch (TimeoutException e) { e.printStackTrace(); // Handle JDBC query timeout issue } catch (SQLException e) { e.printStackTrace(); // Handle other SQL exceptions }
-
RMI操作超时:
- 可能原因: 在进行 RMI(远程方法调用)操作时,等待远程方法调用结果的时间超过了规定的时间。
- 解决方法: 设置适当的超时时间,或者在远程方法调用时考虑异步调用和超时机制。
javaCopy code
try { RemoteService remoteService = (RemoteService) Naming.lookup("rmi://example.com/RemoteService"); String result = remoteService.invokeMethodWithTimeout(5000); // 5000 milliseconds timeout // Process the result } catch (TimeoutException e) { e.printStackTrace(); // Handle RMI operation timeout issue } catch (RemoteException | NotBoundException | MalformedURLException e) { e.printStackTrace(); // Handle other RMI exceptions }
-
远程调用超时:
- 可能原因: 在进行远程调用(如 HTTP、gRPC 等)时,等待调用结果的时间超过了规定的时间。
- 解决方法: 设置适当的超时时间,使用异步调用或考虑实现超时机制。
javaCopy code
OkHttpClient client = new OkHttpClient.Builder() .connectTimeout(5, TimeUnit.SECONDS) // 5 seconds connection timeout .readTimeout(5, TimeUnit.SECONDS) // 5 seconds read timeout .build(); Request request = new Request.Builder() .url("http://example.com/resource") .build(); try (Response response = client.newCall(request).execute()) { // Perform operations with the response } catch (TimeoutException e) { e.printStackTrace(); // Handle remote call timeout issue } catch (IOException e) { e.printStackTrace(); // Handle other IO exceptions }
确保在处理 TimeoutException
时,适当地检查异常的类型,并采取适当的处理措施
更多推荐
所有评论(0)