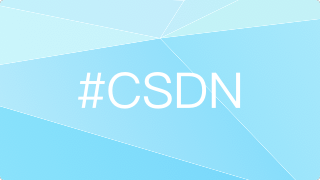
基于Python和Selenium的大麦网自动抢票脚本
自动化抢票的脚本(优化版本)

一键AI生成摘要,助你高效阅读
问答
·
自动化抢票的脚本,需通过Selenium库来实现自动化操作。(学习参考)
# 导入必要的库
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
import time
# 设置浏览器参数
options = webdriver.ChromeOptions()
options.add_argument("--incognito")
options.add_argument("--start-maximized")
# 创建浏览器对象
browser = webdriver.Chrome(options=options)
# 访问大麦网登录页面
browser.get('https://passport.damai.cn/login?ru=https%3A%2F%2Fwww.damai.cn%2F')
# 等待登录页面加载完成
WebDriverWait(browser, 10).until(EC.presence_of_element_located((By.XPATH, '//div[@class="login-title"]')))
# 输入账号密码并点击登录
username = 'your_username' # 替换为自己的账号
password = 'your_password' # 替换为自己的密码
browser.find_element_by_xpath('//input[@name="username"]').send_keys(username)
browser.find_element_by_xpath('//input[@name="password"]').send_keys(password)
browser.find_element_by_xpath('//button[text()="登录"]').click()
# 等待登录成功并跳转到抢票页面
WebDriverWait(browser, 10).until(EC.presence_of_element_located((By.XPATH, '//div[@class="page__title"]')))
browser.get('https://detail.damai.cn/item.htm?id=123456') # 替换为自己要抢票的演出链接
# 等待抢票页面加载完成
WebDriverWait(browser, 10).until(EC.presence_of_element_located((By.XPATH, '//div[@class="buybtn"]')))
# 开始不断刷新页面,直到出现“立即购买”按钮
while True:
try:
browser.refresh()
buy_btn = browser.find_element_by_xpath('//div[@class="buybtn"]')
if buy_btn.text == '立即购买':
break
except:
pass
time.sleep(1)
# 点击“立即购买”按钮
buy_btn.click()
# 等待选择票框加载完成
WebDriverWait(browser, 10).until(EC.presence_of_element_located((By.XPATH, '//div[@class="select_right"]')))
# 选择票框、票价和数量(这里只选择一张最便宜的票)
browser.find_element_by_xpath('//span[text()="选择票框"]').click()
WebDriverWait(browser, 10).until(EC.presence_of_element_located((By.XPATH, '//ul[@class="perform__select__list"]')))
select_list = browser.find_elements_by_xpath('//ul[@class="perform__select__list"]//li')
select_list[0].click()
WebDriverWait(browser, 10).until(EC.presence_of_element_located((By.XPATH, '//span[@class="select_right_list_content_price_text"]')))
select_price = browser.find_elements_by_xpath('//span[@class="select_right_list_content_price_text"]')[0]
select_price.click()
WebDriverWait(browser, 10).until(EC.presence_of_element_located((By.XPATH, '//div[@class
下面是注释
# 初始化浏览器
browser = webdriver.Chrome()
# 找到包含选项的元素列表
select_list = browser.find_elements_by_xpath('//ul[@class="perform__select__list"]//li')
# 点击第一个选项
select_list[0].click()
# 等待价格元素出现
WebDriverWait(browser, 10).until(EC.presence_of_element_located((By.XPATH, '//span[@class="select_right_list_content_price_text"]')))
# 找到价格元素
select_price = browser.find_elements_by_xpath('//span[@class="select_right_list_content_price_text"]')[0]
# 点击价格元素
select_price.click()
# 等待某个元素出现
WebDriverWait(browser, 10).until(EC.presence_of_element_located((By.XPATH, '//div[@class="some_class"]')))
优化后的完整代码:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
import time
def login_and_buy_ticket(username, password, performance_link):
# 设置浏览器参数
options = webdriver.ChromeOptions()
options.add_argument("--incognito")
options.add_argument("--start-maximized")
# 创建浏览器对象
with webdriver.Chrome(options=options) as browser:
# 访问大麦网登录页面
browser.get('https://passport.damai.cn/login?ru=https%3A%2F%2Fwww.damai.cn%2F')
# 等待登录页面加载完成并输入账号密码
try:
WebDriverWait(browser, 10).until(EC.presence_of_element_located((By.XPATH, '//div[@class="login-title"]')))
browser.find_element_by_xpath('//input[@name="username"]').send_keys(username)
browser.find_element_by_xpath('//input[@name="password"]').send_keys(password)
browser.find_element_by_xpath('//button[text()="登录"]').click()
except Exception as e:
print(f"Error during login: {e}")
return
# 等待登录成功并跳转到抢票页面
try:
WebDriverWait(browser, 10).until(EC.presence_of_element_located((By.XPATH, '//div[@class="page__title"]')))
browser.get(performance_link)
except Exception as e:
print(f"Error navigating to performance page: {e}")
return
# 等待抢票页面加载完成并开始不断刷新页面,直到出现“立即购买”按钮
while True:
try:
browser.refresh()
buy_btn = WebDriverWait(browser, 10).until(EC.presence_of_element_located((By.XPATH, '//div[@class="buybtn"]')))
if buy_btn.text == '立即购买':
buy_btn.click()
break
except Exception as e:
print(f"Error while waiting for buy button: {e}")
time.sleep(2)
# 选择票框、票价和数量
try:
WebDriverWait(browser, 10).until(EC.presence_of_element_located((By.XPATH, '//div[@class="select_right"]')))
browser.find_element_by_xpath('//span[text()="选择票框"]').click()
select_list = WebDriverWait(browser, 10).until(EC.presence_of_all_elements_located((By.XPATH, '//ul[@class="perform__select__list"]//li')))
select_list[0].click()
select_price = WebDriverWait(browser, 10).until(EC.presence_of_all_elements_located((By.XPATH, '//span[@class="select_right_list_content_price_text"]')))[0]
select_price.click()
except Exception as e:
print(f"Error while selecting tickets: {e}")
# 调用函数
login_and_buy_ticket('your_username', 'your_password', 'https://detail.damai.cn/item.htm?id=123456')
需要注意的:
- 我用了
with
语句保证浏览器实例在函数结束后正确关闭。 - 我用了几个
try-except
块处理在各个步骤可能出现的异常,它可以在异常的时候打印出错误的信息。 - 我把大部分代码封装在一个函数中,你如果需要,就可以轻轻松松再次调用该函数或者用作其他脚本的部分。
更多推荐
所有评论(0)