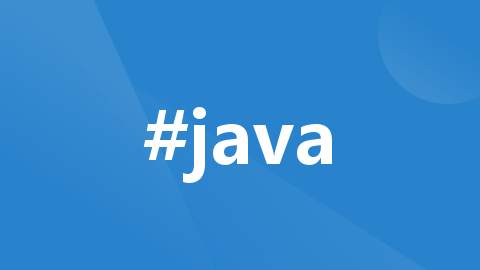
用Java实现图书管理系统
一.前言
大家都用过图书管理系统吧,管理员可以进行查找图书,新增图书,删除图书等一系列的操作,学生可以进行查找图书,借阅图书,归还图书等一系列的操作。那具体怎么实现呢?下面的博客内容带你揭秘!
二.图管的主要结构及具体实现代码
2.1书与书架简述
首先这是一个图书管理系统,我们就肯定要有书,有书就要就有一个书架来存放这些书,这个思路是没有问题的吧,所以我们就先创建两个类,一个是Book类,一个是BookList类,在此之前,我们需要先创建一个Book包来存放这两个类。如下图:
2.2书与书架的具体实现代码
首先我们要在书这个类里设置参数,一个书应该有什么?比如:书的名字?作者?价格?类型?然后我们需要知道这本书此时的状态,是否已经被借出?
所以我们需要五个参数,name,author,type,price ,isBorrowed
代码如下展示:
public class Book{
private String name;
private String author;
private int price;
private String type;
private boolean isBorrowed;
}
我们此时的修饰限定符为什么不用public而用private呢?因为我们想让这些参数私有,而不想任何人都可以调用它。
之后我们写出前四个参数的构造方法及getting和setting方法,而构造方法不需要isBorrowed,因为它的类型是boolean类型,初始一定是false,对应到图管一定是未被借出的,此后我们再重新一下toString方法,但是它最后一句我们需要修改一下使得更符合我们的要求。
下面是书类的代码,展示如下:
public Book(String name,String author,int price,String type){
this.name = name;
this.author = author;
this.price = price;
this.type = type;
}
public String getName(){
return name;
}
public void setName(String name){
this.name = name;
}
public String getAuthor(){
return author;
}
public void setAuthor(String author){
this.author = author;
}
public int getPrice(){
return price;
}
public void setPrice(int price){
this.price = price;
}
public String getType(){
return type;
}
public void setType(String type){
this.type = type;
}
public boolean isBorrowed(){
return isBorrowed;
}
public void isBorrowed(boolean isBorrowed){
isborrowed = Borrowed;
}
@Override
public String toString(){
return "Book{" +
"name='" + name + '\'' +
", author='" + author + '\'' +
", price=" + price +
", type='" + type + '\'' +
( (isBorrowed == true) ? "已经借出" : "未被借出")+
'}';
}
}
下面是书架类的代码,展示如下:
public class BookList{
private Book[] books;
private int usedSize;//记录当前书架上 实际存放的书的 数量 默认为0
private static final int DEFAULT_CAPACITY = 10;
public BookList(){
this.books = new Book[DEFAULT_CAPACITY];
this.book[0] = new Book("三国演义","罗贯中",10,"小说");
this.book[1] = new Book("西游记","吴承恩",9,"小说");
this.book[2] = new Book("红楼梦","曹雪芹",19,"小说");
this.usedSize = 3;
}
public int getUsedSize(){
return usedSize;
}
public void setUsedSize(int usedSize){
this.usedSize = usedSize;
}
public Book getBook(int pos){
return books[pos];
}
public void setBooks(int pos,Book book){
books[pos] = book;
}
public Book[] getBooks(){
return books;
}
}
3.1用户简述
我们图管一共有两种用户:管理员和学生,他们都是用户的支类,所以我们此时创建一个User,作为我们的父类,管理员类AdminUser和学生类NormalUser作为子类,关于父类和子类的概念可以参考我前面的博客,很详细,这三个类都放在user这个包下,如下图展示:
3.2用户的具体实现代码
3.2.1User父类的代码
接下来我们就要在这个user类中设置参数,首先我们肯定需要名字,然后想要进行的操作名称,我们把各种操作封装成一个数组,然后再创建出User的构造方法,之后我们当选择某个操作时,需要调出菜单,所以这个时候设置一个菜单,因为此菜单是抽象方法,所以我们这个User类也要设置为抽象类,然后我们再创建一个做操作的构造方法,当用户选择数字几时,我们调用哪个操作方法,大概准备工作就这些。代码如下展示:
public abstract class User{
protected String name;
protected IOPeration[] ioPerations;
public User(String name){
this.name = name;
}
public abstract int menu();
public void doOperation(int choice,BookList bookList){
ioOperation[choice].work(bookList);
}
}
3.2.2管理员AdminUser子类的代码
接下来我们写管理员AdminUser子类的代码,代码如下:
public class AdminUser extends User{
public AdminUser(String name){
super(name);
this.isOperations = new IOPeration[]{
new ExitOperation(),
new FindOperation(),
new AddOperation(),
new DelOperation(),
new ShowOperation()
};
}
public int menu(){
System.out.println("*********管理员用户******");
System.out.println("1.查找图书");
System.out.println("2.新增图书");
System.out.println("3.删除图书");
System.out.println("4.显示图书");
System.out.println("0.退出系统");
System.out.println("***********************");
Scanner scanner = new Scanner(System.in);
System.out.println("请输入你的操作:");
int choice = scanner.nextInt();
return choice;
}
}
因为管理员AdminUser是User的子类,所以我们要对父类的构造方法进行重载,然后构造出一个菜单,然后返回你的操作的数字标。
3.2.3学生NormalUser子类的代码
接下来我们写学生NormalUser子类的代码,代码和管理员AdminUser子类相似,如下展示:
public class NormalUser extends User{
public NormalUser(String name) {
super(name);
this.ioPerations = new IOPeration[]{
new ExitOperation(),
new FindOperation(),
new BorrowOperation(),
new ReturnOperation()
};
}
public int menu(){
System.out.println("*********普通用户*******");
System.out.println("1.查找图书");
System.out.println("2.借阅图书");
System.out.println("3.归还图书");
System.out.println("0.退出系统");
System.out.println("**********************");
Scanner scanner = new Scanner(System.in);
System.out.println("请输入你的操作:");
int choice = scanner.nextInt();
return choice;
}
}
4.1操作方法简述
我们首先要弄清楚图管的各种方法,管理员有查找图书,新增图书,删除图书,显示图书,退出系统方法等,学生有查找图书,借阅图书,归还图书,退出系统方法等。
首先我们要创建一个operation的包,里面包含这些方法类,展示如下图:
注:请注意里面有一个接口哦!
4.2操作方法的具体实现代码
有细心的小伙伴可能发现这个包中有一个操作接口,这是因为我们无论选择数字几,之后进行的操作都需要提醒一下刚才选择的是什么,如下图所示:
所以此时需要一个接口来完成这一目的。接口代码如下:
public interface IOPeration{
void work(BookList bookList);
}
4.2.1管理员AdminUser类的方法的代码
接下来我们完成管理员AdminUser类的方法的代码,总共有查找图书,新增图书,删除图书,显示图书,退出系统等五个方法,代码如下:
1.查找图书
public class FindOperation implements IOPeration{
@Override
public void work(BookList bookList){
System.out.println("查找图书");
Scanner scanner = new Scanner(System.in);
System.out.println("请输入你要查找的图书:);
String name = scanner.nextLine();
int currentSize = bookList.getUsedSize();
for(int i = 0;i < currentSize; i++){
Book book = bookList.getBook(i);
if(book.getName().equals(name){
System.out.println("找到了这本书,信息如下:");
System.out.println(book);
return;
}
}
System.out.println("没有找到这本书!");
}
}
2.新增图书
public class AddOperation implements IOPeration{
@Override
public void work(BookList bookList){
System.out.println("新增图书!");
Scanner scanner = new Scanner(System.in);
System.out.println("请输入书名:");
String name = scanner.nextLine();
System.out.println("请输入作者:");
String author = scanner.nextLine();
System.out.println("请输入类型:");
String type = scanner.nextLine();
System.out.println("请输入价格:");
int price = scanner.nextInt();
Book book = new Book(name,author,price,type);
//检查数组中有没有这本书
int currentSize = bookList.getUsedSize();
for(int i = 0;i < currentSize;i++){
Book book1 = bookList.getBook(i);
if(book1.getName().equals(name)){
System.out.println("有这本书,不进行存放了!");
return;
}
}
if(currentSize == bookList.getBooks().length){
System.out.println("书架满了!");
}else{
bookList.setBooks(currentSize,book);
bookList.setUsedSize(currentSize+1);
System.out.println("新增图书成功!");
}
}
}
3.删除图书
public class DelOperation implements IOPeration{
@Override
public void work(BookList bookList){
System.out.println("删除图书!");
Scanner scanner = new Scanner(System.in);
System.out.println("请输入你要删除的图书:");
String name = scanner.nextLine();
int pos = -1;
int currentSize = bookList.getUsedSize();
int i = 0;
for(;i<currentSize;i++){
Book book = bookList.getBook(i);
if(book.getName().equals(name)){
pos = i;
break;
}
}
if(i == currentSize){
System.out.println("没有你要删除的图书!");
return;
}
//开始删除
int j = pos;
for(;j<currentSize-1;j++){
//[j] = [j+1]
Book book = bookList.getBook(j+1);
bookList.setBooks(j,book);
}
bookList.setBooks(j,null);
booksList.setUsedSize(currentSize-1);
System.out.println("删除图书成功!");
}
}
4.显示图书
public class ShowOperation implements IOPeration{
@Override
public void work(BookList bookList){
System.out.println("显示图书!");
int currentSize = bookList.getUsedSize();
for(int i = 0;i<currentSize;i++){
Book book = booksList.getBook(i);
System.out.println(book);
}
}
}
5.退出系统
public class ExitOperation implements IOPeration{
@Override
public void work(BookList bookList){
System.out.println("退出系统");
System.exit(0);
}
}
4.2.2学生NormalUser类的方法的代码
在完成管理员AdminUser类的方法的代码后,接下来我们完成学生NormalUser类的方法的代码,总共有查找图书,借阅图书,归还图书,退出系统等四个方法,代码如下:
1.查找图书
public void work(BookList bookList){
System.out.println("查找图书!");
Scanner scanner = new Scanner(System.in);
System.out.println("请输入你要查找的图书:");
String name = scanner.nextLine;
int currentSize = bookList.getUsedSize();
for(int i = 0;i < currentSize;i++){
Book book = bookList.getBook(i);
if(book.getName().equals(name)){
System.out.println("找到了这本书,信息如下:");
System.out.println(book);
return;
}
}
System.out.println("没有找到这本书!");
}
}
2.借阅图书
public class BorrowOperation implements IOPeration{
System.out.println("借阅图书!");
//你要借哪本书
//你借阅的书有没有
//借阅的方式是什么? -》isBorrowed = ture
Scanner scanner = new Scanner(System.in);
System.out.println("请输入你要借阅的图书:");
String name = scanner.nextLine();
int currentSize = bookList.getUsedSize();
for(int i = 0;i < currentSize;i++){
Book book = bookList.getBook(i);
if(book.getName().equals(name)){
book.setBorrowed(ture);
System.out.println("借阅成功!");
System.out.println(book);
return;
}
}
System.out.println("你借阅的图书不存在!");
}
}
3.归还图书
public class ReturnOperation implements IOPeration{
@Override
public class work(BookList bookList){
System.out.println("归还图书!");
Scanner scanner = new Scanner(System.in);
System,out.println("请输入你要借阅的图书:");
String name = scanner.nextLine();
int currentSize = bookList.getUsedSize();
for(int i = 0; i<currentSize; i++){
Book book = bookList.getBook(i);
if(book.getName().equals(name){
book.setBorrowed(false);
System.out.println("归还成功!");
System.out.println(book);
return;
}
}
System.out.println("你归还的图书不存在!");
}
}
4.退出系统
public class ExitOperation implements IOPeration{
@Override
public class work(BookList bookList);{
System.out.println("退出系统!");
System.exit(0);
}
}
这就是管理员类和学生类的方法的全部代码啦~
5.1主函数简述
首先我们对书架进行创建和初始化,然后写一个登录方法,因为我们不止一次进行选择,所以在后面我们要设置一个循环,在循环里我们调用之前我们的选择及方法,代码如下
5.2主函数的具体实现代码
public class Main{
public static User login(){
System.out.println("请输入你的姓名:");
Scanner scanner = new Scanner(System.in);
String name = scanner.nextLine();
System.out.println("请输入你的身份:1:管理员 2.普通用户 -》");
int choice = scanner.nextInt();
if(choice == 1){
//管理员
return new AdminUser(name);
}else{
//普通用户
return new NormalUser(name);
}
}
public static void main(String[] args){
BookList bookList = new BookList();//书架的创建及初始化
//user 指向哪个对象 就看返回值是什么
User user = login();//登录
while(ture){
int choice = user.menu();
//根据choice的选择 来决定调用哪个方法
user.doOperation(choice,bookList);
}
}
}
三.尾语
这篇博客到这里就结束啦,希望大家都可以写出来自己的图书管理系统呦~~~
更多推荐
所有评论(0)