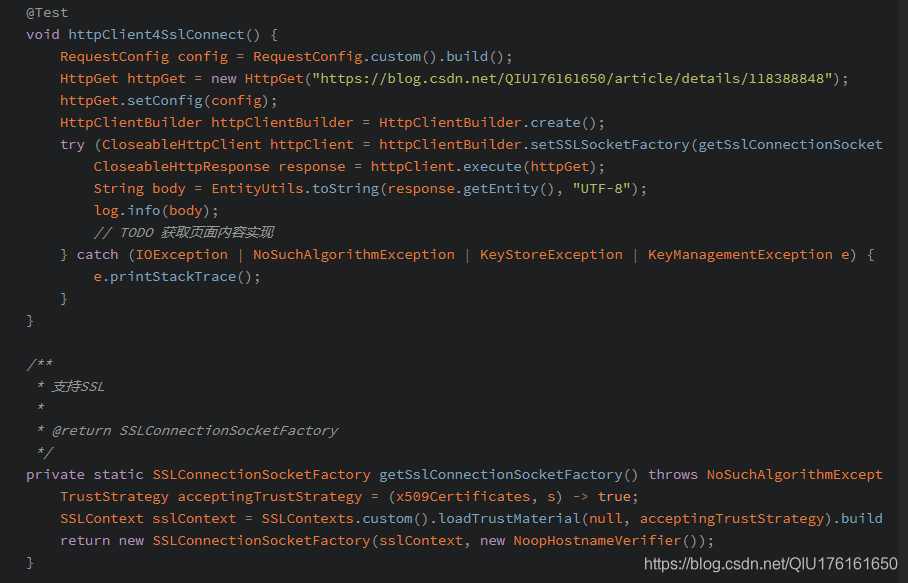
HttpClient支持HTTPS(SSL),忽略安全证书配置,一文搞懂
文章目录问题描述解决方案:HttpClient4.5中支持HTTPS请求处理方法HttpClient5.1中支持HTTPS请求处理方法问题描述最近在学习HttpClient做爬虫,尝试使之能够支持HTTPS请求,忽略安全证书。在网上找了很久的资料,发现这方面的资料很少,而且多数都不准确。故记录一下。解决方案:HttpClient4.x与HttpClient5.x中配置还是有所不同,以下为大家两个版

一键AI生成摘要,助你高效阅读
问答
·
问题描述
最近在学习HttpClient做爬虫,尝试使之能够支持HTTPS请求,忽略安全证书。在网上找了很久的资料,发现这方面的资料很少,而且多数都不准确。故记录一下。
解决方案:
HttpClient4.x与HttpClient5.x中配置还是有所不同,以下为大家两个版本的处理方法。
HttpClient4.5中支持HTTPS请求处理方法
Maven中引入配置:
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
代码如下:
package com.example.demo;
import lombok.extern.slf4j.Slf4j;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.conn.ssl.NoopHostnameVerifier;
import org.apache.http.conn.ssl.SSLConnectionSocketFactory;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.ssl.SSLContexts;
import org.apache.http.ssl.TrustStrategy;
import org.apache.http.util.EntityUtils;
import org.junit.jupiter.api.Test;
import javax.net.ssl.SSLContext;
import java.io.IOException;
import java.security.KeyManagementException;
import java.security.KeyStoreException;
import java.security.NoSuchAlgorithmException;
@Slf4j
public class HttpClientTests {
@Test
void httpClient4SslConnect() {
RequestConfig config = RequestConfig.custom().build();
HttpGet httpGet = new HttpGet("https://blog.csdn.net/QIU176161650/article/details/118388848");
httpGet.setConfig(config);
HttpClientBuilder httpClientBuilder = HttpClientBuilder.create();
try (CloseableHttpClient httpClient = httpClientBuilder.setSSLSocketFactory(getSslConnectionSocketFactory()).build()) {
CloseableHttpResponse response = httpClient.execute(httpGet);
String body = EntityUtils.toString(response.getEntity(), "UTF-8");
log.info(body);
// TODO 获取页面内容实现
} catch (IOException | NoSuchAlgorithmException | KeyStoreException | KeyManagementException e) {
e.printStackTrace();
}
}
/**
* 支持SSL
*
* @return SSLConnectionSocketFactory
*/
private static SSLConnectionSocketFactory getSslConnectionSocketFactory() throws NoSuchAlgorithmException, KeyStoreException, KeyManagementException {
TrustStrategy acceptingTrustStrategy = (x509Certificates, s) -> true;
SSLContext sslContext = SSLContexts.custom().loadTrustMaterial(null, acceptingTrustStrategy).build();
return new SSLConnectionSocketFactory(sslContext, new NoopHostnameVerifier());
}
}
HttpClient5.1中支持HTTPS请求处理方法
Maven中引入配置:
<dependency>
<groupId>org.apache.httpcomponents.client5</groupId>
<artifactId>httpclient5</artifactId>
<version>5.1</version>
</dependency>
代码如下:
import lombok.SneakyThrows;
import lombok.extern.slf4j.Slf4j;
import org.apache.hc.client5.http.classic.methods.HttpGet;
import org.apache.hc.client5.http.config.RequestConfig;
import org.apache.hc.client5.http.impl.DefaultSchemePortResolver;
import org.apache.hc.client5.http.impl.classic.CloseableHttpClient;
import org.apache.hc.client5.http.impl.classic.CloseableHttpResponse;
import org.apache.hc.client5.http.impl.classic.HttpClientBuilder;
import org.apache.hc.client5.http.impl.io.PoolingHttpClientConnectionManagerBuilder;
import org.apache.hc.client5.http.impl.routing.DefaultRoutePlanner;
import org.apache.hc.client5.http.io.HttpClientConnectionManager;
import org.apache.hc.client5.http.ssl.NoopHostnameVerifier;
import org.apache.hc.client5.http.ssl.SSLConnectionSocketFactory;
import org.apache.hc.core5.http.HttpHost;
import org.apache.hc.core5.http.ParseException;
import org.apache.hc.core5.http.io.entity.EntityUtils;
import org.apache.hc.core5.http.protocol.HttpContext;
import org.apache.hc.core5.ssl.SSLContexts;
import org.apache.hc.core5.ssl.TrustStrategy;
import org.junit.jupiter.api.Test;
import javax.net.ssl.SSLContext;
import java.io.IOException;
import java.net.InetAddress;
import java.security.KeyManagementException;
import java.security.KeyStoreException;
import java.security.NoSuchAlgorithmException;
@Slf4j
public class HttpClient5Test {
@Test
void httpClient5SslConnect() {
RequestConfig config = RequestConfig.custom().build();
HttpGet httpGet = new HttpGet("https://blog.csdn.net/QIU176161650/article/details/118388848");
httpGet.setConfig(config);
HttpClientBuilder httpClientBuilder = HttpClientBuilder.create();
try (CloseableHttpClient httpClient = httpClientBuilder.setConnectionManager(getHttpClientConnectionManager()).build()) {
CloseableHttpResponse response = httpClient.execute(httpGet);
String body = EntityUtils.toString(response.getEntity(), "UTF-8");
log.info(body);
// TODO 获取页面内容实现
} catch (IOException | ParseException | NoSuchAlgorithmException | KeyStoreException | KeyManagementException e) {
e.printStackTrace();
}
}
private static HttpClientConnectionManager getHttpClientConnectionManager() throws NoSuchAlgorithmException, KeyStoreException, KeyManagementException {
return PoolingHttpClientConnectionManagerBuilder.create()
.setSSLSocketFactory(getSslConnectionSocketFactory())
.build();
}
/**
* 支持SSL
*
* @return SSLConnectionSocketFactory
*/
private static SSLConnectionSocketFactory getSslConnectionSocketFactory() throws NoSuchAlgorithmException, KeyStoreException, KeyManagementException {
TrustStrategy acceptingTrustStrategy = (x509Certificates, s) -> true;
SSLContext sslContext = SSLContexts.custom().loadTrustMaterial(null, acceptingTrustStrategy).build();
return new SSLConnectionSocketFactory(sslContext, new NoopHostnameVerifier());
}
}
更多推荐
所有评论(0)