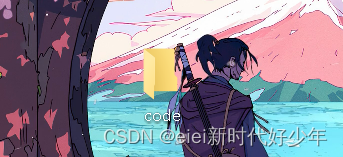
【Anime.js】——JavaScript动画库:Anime.js——学习笔记
目录
官网定义:
anime.js 是一个简便的JS动画库,用法简单而且适用范围广,涵盖CSS,DOM,SVG还有JS的对象,各种带数值属性的东西都可以动起来。
一、搭建开发环境
1、新建一个文件夹 ,用vs code打开,将其添加到工作区。
2、打开终端
在终端输入:
npm i animejs --save
3、创建文件夹,使用anime.js包
位置如图。
4、实现一个简易的动画样式
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div{
width: 100px;
height: 100px;
background-color: brown;
}
</style>
</head>
<body>
<div></div>
<script src="node_modules/animejs/lib/anime.min.js"></script>
<script>
anime({
targets:'div',
translateX:'400px',
easing:'linear',
duration:2000
})
</script>
</body>
</html>
就能实现一个方块匀速运动的效果了。
二、基本功能和使用
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div{
width: 100px;
height: 100px;
background-color: blueviolet;
}
</style>
</head>
<body>
<div id = 'first'></div>
<div class = 'second'></div>
<div class = 'second'></div>
<div class = 'second'></div>
<script src="../node_modules/animejs/lib//anime.min.js"></script>
<script>
anime({
targets:'#first',
translateX:'400px'
})
</script>
</body>
</html>
第一个div动:
如果把代码改成这样:
<script>
anime({
targets:'.second',
translateX:'400px'
})
</script>
可以看到,targets用来作为选择器来选择移动元素。
开始制作动画
anime({
targets: 'div',
translateX: [
{ value: 100, duration: 1200 },
{ value: 0, duration: 800 }
],
rotate: '1turn',
backgroundColor: '#FFF',
duration: 2000,
loop: true
});
targets属性定义了制作动画的元素或js对象:
- css选择器 如:'div','#el path';
- DOM元素 如: document.querySelector('.item')
- 节点列表 如: document.querySelectorAll('.item')
- 对象
- 数组 如:['div', '.item', domNode]
动画属性
1、CSS:
- opacity 透明度 0~1
- backgroundColor
- fontSize
- borderRadius
- backgroundColor
2、transforms变换:
- translateX x轴的值
- translateY y轴的值
- retate 旋转
- scale 大小变换 例:scale:2 scale:0.5
- rotate 旋转 例:rotate:'1turn'(旋转一周)
3、对象属性
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div {
width: 100px;
height: 100px;
background-color: cadetblue;
}
</style>
</head>
<body>
<div id="JSobjectProp">
<span>{"myProperty":"0"}</span>
</div>
<script src="../node_modules/animejs/lib//anime.min.js"></script>
<script>
var myObject = {
prop1: 0,
prop2: '0%'
}
var JSobjectProp = anime({
targets: myObject,
prop1: 50,
prop2: '100%',
easing: 'linear',
round: 1,
update: function () {
var el = document.querySelector('#JSobjectProp span');
el.innerHTML = JSON.stringify(myObject);
}
});
</script>
</body>
</html>
开始:
变为:
4、DOM属性
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
</style>
</head>
<body>
<input class="text-output" value="0">
<script src="../node_modules/animejs/lib//anime.min.js"></script>
<script>
var domAttributes = anime({
targets: 'input',
value: 1000, // value变化为1000
round: 100, //表示小数,把1分成了100份,也就是展示两位小数点
easing: 'easeInOutExpo'
});
</script>
</body>
</html>
5、给每个属性单独设置
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div {
width: 100px;
height: 100px;
background-color: cadetblue;
}
</style>
</head>
<body>
<div></div>
<script src="../node_modules/animejs/lib//anime.min.js"></script>
<script>
anime({
targets: 'div',
translateX: {
value: 250,//通过value来设置值
duration: 800 //表示延长动画效果的时间
},
rotate: {
value: 360,
duration: 1800,
easing: 'easeInOutSine'
},
scale: {
value: 2,
duration: 1600,
delay: 800,
easing: 'easeInOutQuart'
},
delay: 250 // All properties except 'scale' inherit 250ms delay
});
</script>
</body>
</html>
6、属性参数
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
div {
width: 100px;
height: 100px;
background-color: cadetblue;
}
</style>
</head>
<body>
<div></div>
<script src="../node_modules/animejs/lib//anime.min.js"></script>
<script>
anime({
targets: 'div',
translateX: 270,
direction: 'alternate',
loop: true,
// 接收三个参数:
// el:表示当前目标元素
// i: 表示当前目标元素下标
// l:表示目标元素的总长度
delay: function (el, i, l) {
return i * 100;
},
endDelay: function (el, i, l) {
return (l - i) * 100;
}
});
</script>
</body>
</html>
三、anime.stagger——交错动画
1、
anime.stagger(value, options)
//它针对多个元素。
//第一个参数接收:Number, String, Array
//第二个参数接收:Object
anime({
targets: '.basic-staggering-demo .el',
translateX: 270,
delay: anime.stagger(100) // increase delay by 100ms for each elements.
});
效果:
2、
anime.stagger(value, {start: startValue}) //在value后面添加对象属性
anime({
targets: '.staggering-start-value-demo .el',
translateX: 270,
delay: anime.stagger(100, {start: 500}) // delay starts at 500ms then increase by 100ms for each elements.
});
3、
anime.stagger([startValue, endValue]) //作为rotate旋转的属性值
anime({
targets: '.range-value-staggering-demo .el',
translateX: 270,
rotate: anime.stagger([-360, 360]), // rotation will be distributed from -360deg to 360deg evenly between all elements
easing: 'easeInOutQuad'
});
4、
anime.stagger(value, {from: startingPosition}) //从哪个位置依次延迟
anime({
targets: '.staggering-from-demo .el',
translateX: 270,
delay: anime.stagger(100, {from: 'center'})
});
5、
anime.stagger(value, {direction: 'reverse'}) //反向延迟
anime({
targets: '.staggering-direction-demo .el',
translateX: 270,
delay: anime.stagger(100, {direction: 'reverse'})
});
6、
anime.stagger(value, {grid: [rows, columns]})
anime({
targets: '.staggering-grid-demo .el',
scale: [
{value: .1, easing: 'easeOutSine', duration: 500},
{value: 1, easing: 'easeInOutQuad', duration: 1200}
],
//grid: [14, 5]表示14行5列
//from 表示从哪里开始
delay: anime.stagger(200, {grid: [14, 5], from: 'center'})
});
7、
anime.stagger(value, {grid: [rows, columns], axis: 'x'})
//axis设置为x,表示将一整行设置为整体
//设为y,会将一整列设置为整体
anime({
targets: '.staggering-axis-grid-demo .el',
translateX: anime.stagger(10, {grid: [14, 5], axis: 'x'})
})
四、timeline——时间轴
接下来学习官网的时间轴模块。
这个模块告诉我们可以创建一个时间轴,在这个时间轴上可以创建多个动画,并且添加在时间轴上的每一个动画都会在上一个动画结束之后开始执行。
1、创建时间轴
创建一个时间轴:
//调用该方法:
var myTimeline = anime.timeline(parameters);
如何通过这个时间轴调用对象:
myTimeline.add(parameters, offset);
//parameters 动画相关的参数
//offset 时间偏移量
实例:
// 创建了一个时间轴对象
var tl = anime.timeline({
easing: 'easeOutExpo',
duration: 750 // 持续时间是750ms
});
// 时间轴上分别创建了三个动画
tl
.add({
targets: '.basic-timeline-demo .el.square', //方形
translateX: 250, // 平移250px
})
.add({
targets: '.basic-timeline-demo .el.circle', //圆形
translateX: 250,
})
.add({
targets: '.basic-timeline-demo .el.triangle', //三角形
translateX: 250,
});
会等到上一个方块执行完,才会执行圆。
2、时间偏移量
时间偏移量是我们创建时间轴的第二个参数。
默认情况下,每一个动画都会在上一个动画结束之后开始执行,通过时间偏移量就可以改变每个动画开始执行的时间。
如下图可以看到,时间偏移量可以有两种数据类型:String字符串、Number数字
字符串是相对于上一个动画的设置的,比如:
'+=200'表示:是上一个动画执行结束完200ms后再执行当前的动画
'-=200'表示:是上一个动画执行结束前200ms后执行当前的动画
数字偏移量是相对于整个时间轴的绝对偏移量。
200表示:当前时间轴开始200ms后执行。
// Create a timeline with default parameters
var tl = anime.timeline({
easing: 'easeOutExpo',
duration: 750
});
tl
.add({
targets: '.offsets-demo .el.square',
translateX: 250,
})
.add({
targets: '.offsets-demo .el.circle',
translateX: 250,
}, '-=600') // 相对偏移量————字符创
.add({
targets: '.offsets-demo .el.triangle',
translateX: 250,
}, 400); // 绝对偏移量————数字
3、参数继承
添加在时间轴中的动画,都可以继承表格里的这些属性 |
targets |
easing |
duration |
delay |
endDelay |
round |
注意:
direction 和 loop 是不可以被继承的。
演示:(继承targets属性)
五、控制、回调与助手
一、控制
页面中需要的元素:
1、动画上的属性
animation.play(); //开始
animation.pause(); //暂停
animation.restart(); //重新开始
animation.reverse(); //反向移动
// seek 当前元素显示在具体时间的某一帧
// timeStamp:跳到具体的时间
animation.seek(timeStamp);
// 通过滚动条来控制动画
// scrollPercent / 100:求滚动条的百分比
// animation.duration:当前动画的时间
// (scrollPercent / 100) * animation.duration:具体跳到的时间值
animation.seek((scrollPercent / 100) * animation.duration);
2、时间轴上的属性
// 控制时间轴上的动画:
timeline.play();
timeline.pause();
timeline.restart();
timeline.seek(timeStamp);
二、回调
1、UPDATE:每更新一帧就会调用一次
var updates = 0;
anime({
targets: '.update-demo .el',
translateX: 270,
delay: 1000,
direction: 'alternate',
loop: 3,
easing: 'easeInOutCirc',
// anim当前动画的实例
update: function(anim) {
updates++;
// anim.progress 当前动画的进度
progressLogEl.value = 'progress : '+Math.round(anim.progress)+'%';
updateLogEl.value = 'updates : '+updates;
}
});
示例:
表示更新了58帧。
2、BEGIN & COMPLETE
BEGIN:动画开始执行前触发一次
COMPLETE:动画结束时触发一次
这两个属性是布尔类型
示例:
注意箭头处的写法是不一样的!!!
效果:
3、LOOPBEGIN & LOOPCOMPLETE
LOOPBEGIN:循环开始时就会触发一次
LOOPCOMPLETE:循环结束时就会触发一次
注意:动画一定是循环的动画
var loopBegan = 0;
var loopCompleted = 0;
anime({
targets: '.loopBegin-loopComplete-demo .el',
translateX: 240,
loop: true,
direction: 'alternate',
easing: 'easeInOutCirc',
loopBegin: function(anim) {
loopBegan++;
beginLogEl.value = 'loop began : ' + loopBegan;
},
loopComplete: function(anim) {
loopCompleted++;
completeLogEl.value = 'loop completed : ' + loopCompleted;
}
});
4、CHANGE:动画元素每更新一次就调用一次
与update的区别:change在delay和endDelay期间是不更新的,update反之。
var changes = 0;
anime({
targets: '.change-demo .el',
translateX: 270,
delay: 1000,
endDelay: 1000,
direction: 'alternate',
loop: true,
easing: 'easeInOutCirc',
update: function(anim) {
progressLogEl.value = 'progress : '+Math.round(anim.progress)+'%';
},
change: function() {
changes++;
changeLogEl.value = 'changes : ' + changes;
}
});
5、FINISHED PROMISE:每一个动画实例完成都会返回一个promise
// animation表示创建的动画实例
animation.finished.then(function() {
// Do things...
});
var progressLogEl = document.querySelector('.promise-demo .progress-log');
var promiseEl = document.querySelector('.promise-demo .el');
var finishedLogEl = document.querySelector('.promise-demo .finished-log');
var demoPromiseResetTimeout;
function logFinished() {
anime.set(finishedLogEl, {value: 'Promise resolved'});
anime.set(promiseEl, {backgroundColor: '#18FF92'});
}
var animation = anime.timeline({
targets: promiseEl,
delay: 400,
duration: 500,
endDelay: 400,
easing: 'easeInOutSine',
update: function(anim) {
progressLogEl.value = 'progress : '+Math.round(anim.progress)+'%';
}
}).add({
translateX: 250
}).add({
scale: 2
}).add({
translateX: 0
});
animation.finished.then(logFinished);
三、助手
1、anime.remove
// remove 将某个targets对象移出
anime.remove(targets);
animation.remove(targets);
var animation = anime({
targets: '.remove-demo .el',
translateX: 270,
direction: 'alternate',
loop: true,
easing: 'easeInOutQuad'
});
document.querySelector('.remove-el-button').addEventListener('click', function() {
animation.remove('.remove-demo .line:nth-child(2) .el');
});
2、anime.get
// get 获取元素的信息
// target 获取的元素
// propertyName 获取的元素的属性
// unit 单位
anime.get(target, propertyName, unit);
var logEl = document.querySelector('.get-value-demo-log');
var el = document.querySelector('.get-value-demo .el');
logEl.innerHTML = '';
logEl.innerHTML += '".el" width is :<br>';
logEl.innerHTML += '"' + anime.get(el, 'width', 'px') + '"';
logEl.innerHTML += ' or "' + anime.get(el, 'width', 'rem') + 'rem"'
3、anime.set
// set 设置元素的属性值是什么
// {property: value} :是一个对象,{属性:值}
// target(s) 类型是'string', var
// value 类型是object
anime.set(targets, {property: value});
anime.set('.set-value-demo .el', {
translateX: function() { return anime.random(50, 250); },
rotate: function() { return anime.random(0, 360); },
});
4、anime.random
// random 返回一个随机数
anime.random(minValue, maxValue);
function randomValues() {
anime({
targets: '.random-demo .el',
translateX: function() {
return anime.random(0, 270);
},
easing: 'easeInOutQuad',
duration: 750,
complete: randomValues
});
}
randomValues();
5、anime.running
//running 表示动画实例,其长度代表动画个数
anime.running
var runninLogEl = document.querySelector('.running-log');
anime({
targets: '.running-demo .square.el',
translateX: 270,
direction: 'alternate',
loop: true,
easing: 'linear'
});
anime({
targets: '.running-demo .circle.el',
translateX: 270,
direction: 'alternate',
loop: true,
easing: 'easeInOutCirc'
});
anime({
targets: '.running-demo .triangle.el',
translateX: 270,
direction: 'alternate',
easing: 'easeInOutQuad',
loop: true,
update: function() {
runninLogEl.innerHTML = 'there are currently ' + anime.running.length + ' instances running';
}
});
六、easings——动画运动曲线
1、使用
// linear表示线性
easing: 'linear'
2、方法
IN | OUT | IN-OUT | OUT-IN |
'easeInQuad' | 'easeOutQuad' | 'easeInOutQuad' | 'easeOutInQuad' |
'easeInCubic' | 'easeOutCubic' | 'easeInOutCubic' | 'easeOutInCubic' |
'easeInQuart' | 'easeOutQuart' | 'easeInOutQuart' | 'easeOutInQuart' |
'easeInQuint' | 'easeOutQuint' | 'easeInOutQuint' | 'easeOutInQuint' |
'easeInSine' | 'easeOutSine' | 'easeInOutSine' | 'easeOutInSine' |
'easeInExpo' | 'easeOutExpo' | 'easeInOutExpo' | 'easeOutInExpo' |
'easeInCirc' | 'easeOutCirc' | 'easeInOutCirc' | 'easeOutInCirc' |
'easeInBack' | 'easeOutBack' | 'easeInOutBack' | 'easeOutInBack' |
'easeInBounce' | 'easeOutBounce' | 'easeInOutBounce' | 'easeOutInBounce' |
举例:
3、BÉZIER曲线和spring弹簧
二者都是参数改变变化的曲线
// 方法接收4个参数,通过参数来改变运动曲线
easing: 'cubicBezier(.5, .05, .1, .3)'
// mass 质量
// stiffness 高度
// damping 阻尼
// velocity 速度
easing: 'spring(mass, stiffness, damping, velocity)'
属性 | 默认值 | MIN | MAX |
Mass | 1 | 0 | 100 |
Stiffness | 100 | 0 | 100 |
Damping | 10 | 0 | 100 |
Velocity | 0 | 0 | 100 |
4、ELASTIC
// amplitude 振幅
// period 来回的次数
// 也是表示弹簧,只不过弹的方向不一样
easing: 'easeOutElastic(amplitude, period)'
IN | OUT | IN-OUT | OUT-IN |
'easeInElastic' | 'easeOutElastic' | 'easeInOutElastic' | 'easeOutInElastic' |
属性 | 默认值 | MIN | MAX | |
Amplitude | 1 | 1 | 10 | |
Period | .5 | 0.1 | 2 |
5、跳动
// numberOfSteps跳动的步数
easing: 'steps(numberOfSteps)'
属性 | 默认值 | MIN | MAX |
Number of steps | 10 | 1 | ∞ |
6、自定义函数
easing: function() { return function(time) { return time * i} }
注意返回的一定是个函数
anime({
targets: '.custom-easing-demo .el',
translateX: 270,
direction: 'alternate',
loop: true,
duration: 2000,
easing: function(el, i, total) {
return function(t) {
return Math.pow(Math.sin(t * (i + 1)), total);
}
}
});
七、SVG动画
1、运动
// svg path 表示svg的路径
var myPath = anime.path('svg path');
// myPath 接收三个参数
// myPath('x'),传入的是svg path中 x 的值
// myPath('y'),传入的是svg path中 y 的值
// myPath('angle'),传入的是svg path中 角度 的值
var path = anime.path('.motion-path-demo path');
anime({
targets: '.motion-path-demo .el',
translateX: path('x'),
translateY: path('y'),
rotate: path('angle'),
easing: 'linear',
duration: 2000,
loop: true
});
示例:
效果:
2、变形
给大家分享一个svg的绘制编辑器网页:
效果:就可以在页面看到动态的五边形
3、划线
首先要有一个画好的svg的线,然后通过:
strokeDashoffset: [anime.setDashoffset, 0]
来实现划线。
效果:
笔记参考:https://www.jianshu.com/p/39fc8a837b31
官方文档:https://animejs.com/documentation/#functionBasedParameters
更多推荐
所有评论(0)