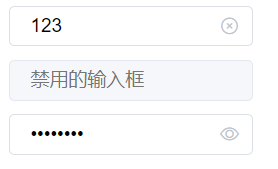
Vue组件封装 ——input 输入框组件
·
一、基础准备工作
1、创建一个基础的vue项目包
2、创建components文件夹,用于存放组件,新建input.vue组件,可以自己取个名字
<script>
export default {
name: "CatInput",
};
</script>
3、在main.js中引入组件
import CatInput from "./components/input.vue";
Vue.component(CatInput.name, CatInput);
4、App.vue中使用组件
二、input组件结构搭建
主要功能为:输入框类型切换、双向数据绑定、清空输入框、密码明文显示切换
输入框需要绑定v-model,实际上是一个语法糖,等价于:
:value="uname"
@input="uname=$event.target.value"
需要传入的参数:
placeholder:字段预期值的提示信息
type:文本框类型
name:name
disabled:是否禁用
value:值
clearable:是否显示清空按钮
showPassword:密码显示
data中,添加字段 passwordVisiable,控制是否明文显示密码,因为子组件无法直接修改父组件传入的type值
页面效果:
input.vue 具体代码如下,style样式太多,不逐一列出了,可根据需求自己定义:
<template>
<div class="cat-input" :class="{ 'cat-input--suffix': showSuffix }">
<!-- type:先判断是否有传入显示密码,控制输入框类型是文本/密码,然后是type传入的值 -->
<input
:type="showPassword ? (passwordVisiable ? 'text' : 'password') : type"
class="cat-input__inner"
:placeholder="placeholder"
:name="name"
:disabled="disabled"
:class="{ 'is-disabled': disabled }"
:value="value"
@input="handleinput"
/>
<span class="cat-input__suffix" v-if="showSuffix">
<i
class="cat-input__icon cat-icon-circle-close"
v-if="clearable && value"
@click="clear"
></i>
<i
class="cat-input__icon cat-icon-view"
:class="{ 'cat-icon-view-active': passwordVisiable }"
v-if="showPassword"
@click="handlepwd"
></i>
</span>
</div>
</template>
<script>
export default {
name: "CatInput",
props: {
placeholder: {
type: String,
default: "",
},
type: {
type: String,
default: "text",
},
name: {
type: String,
default: "",
},
disabled: {
type: Boolean,
default: false,
},
value: {
type: String,
default: "",
},
clearable: {
type: Boolean,
default: false,
},
showPassword: {
type: Boolean,
default: false,
},
},
data() {
return {
passwordVisiable: false, //控制是否显示密码
};
},
methods: {
handleinput(event) {
//父组件在绑定v-model时,其实就绑定的input事件,因此父组件不需要再声明事件了
this.$emit("input", event.target.value);
},
clear() {
this.$emit("input", "");
},
handlepwd() {
this.passwordVisiable = !this.passwordVisiable;
},
},
computed: {
//有清空/显示密码,添加类名、显示span
showSuffix() {
return this.clearable || this.showPassword;
},
},
};
</script>
App.vue使用input组件:
<template>
<!--
输入框需要绑定v-model,实际上是一个语法糖,等价于:
:value="uname"
@input="uname=$event.target.value"
-->
<div>
<Cat-input
placeholder="请输入用户名"
type="text"
v-model="uname"
clearable
></Cat-input>
<br />
<Cat-input placeholder="禁用的输入框" disabled></Cat-input>
<br />
<Cat-input
placeholder="请输入密码"
v-model="upwd"
show-password
></Cat-input>
</div>
</template>
<script>
export default {
data() {
return {
uname: "",
upwd: "",
};
},
};
</script>
<style lang="scss" scoped>
.cat-input {
margin-bottom: 10px;
}
</style>
更多推荐
所有评论(0)