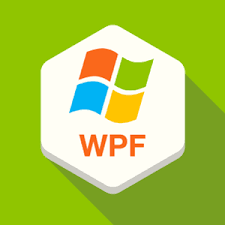
WPF 项目开发入门(三)WPF 窗体与页面
WPF 项目开发入门(一) 安装运行
WPF 项目开发入门(二) WPF 页面布局
WPF 项目开发入门(三)WPF 窗体与页面
WPF 项目开发入门(四) MVVM 模式 与 TreeView树组件
WPF 项目开发入门(五)ListView列表组件 与 Expander组件
WPF 项目开发入门(六)DataGrid组件
WPF 项目开发入门(七) From表单组件
WPF 项目开发入门(八)数据库驱动配置与数据库操作
WPF 项目开发入门(九)数据库连接 NHibernate使用
WPF 项目开发入门(十)DevExpress 插件+NHibernate登录
WPF 项目开发入门(十一)DevExpress 插件 Grid表格应用
在项目中常常会使用到页面跳转功能,在WPF项目的页面跳转中分为窗体跳转和面板跳转两种模式。在WPF中窗体分为三种方式
- Window:桌面程序的窗体。在WPF桌面应用中通常会有一个主窗体,然后将不同的操作单元封装在不同的UserControl中,根据用户的操作展现不同的UserControl。
- Page:Page需要承载在窗体中,通过Navigation进行不同Page的切换;
- UserControl:封装一些可以重复使用的功能。
1 Window窗体页跳转
主窗体打开一个子窗体,两个窗体都是独立的运行程序关系。一般情况是打开子窗体后要关上一个窗体。程序打开了又一个Window窗体组件,这个Window窗体组件是独立的一个窗体。
项目新创建一 zhtapp WPF窗体页面
zhtapp.xaml
<Window x:Class="WpfApp1.zhtapp"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApp1"
mc:Ignorable="d"
Title="zhtapp" Height="850" Width="800">
<Grid>
<Label FontSize="50" Grid.Row="0">我的第一个窗口</Label>
</Grid>
zhtapp.cs 窗体业务逻辑
namespace WpfApp1{
public partial class zhtapp : Window{
public zhtapp(){
InitializeComponent();
}
}
}
2 在 MainWindow.cs 点击事件中调用窗体
// xaml 页面中定义事件
//<Button Name="btnAppBeginInvoke" Click="btnThd_Click" >弹出新页面</Button>
private void btnThd_Click(object sender, RoutedEventArgs e)
{
zhtapp zh= new zhtapp();//直接调用窗体内容
zh.Show();//打开窗体
this.Close(); //关闭当前窗口
}
2 Frame 面板页面跳转
在PWF窗体中定义一个Frame,用它来承载Page页面。肯据业务情况分别定义各种业务(Page)面板,面板在Frame组件中进行页面的迁移功能。
Frame跳转页面中有两个方法Source,Content。
-
Source page项目地址Uri。
Uri uri = new Uri("../Page2.xaml?test_str=" + "开始", UriKind.Relative); framemain.Source = uri
-
Content page实体类对象。
var page=new Page1(); framemain.Content = page// page面板类实例
下面通过设置Frame组件,进行面板页面转发 Page1.xaml、Page2.xaml例子。
- 通过 Frame组件中的Source方法来指定page项目路径转发页面。
Frame框架页面
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="200"></ColumnDefinition>
<ColumnDefinition Width="*"></ColumnDefinition>
</Grid.ColumnDefinitions>
<StackPanel Grid.Row="0" Grid.Column="0">
<Button Click="Button_Click">page1</Button>-------对应c#中Button_Click转发方法
<Button Click="Button_Click1">page2</Button>-------对应c#中Button_Click1转发方法
</StackPanel>
<Frame Name="framemain" NavigationUIVisibility="Hidden" Grid.Row="1" Grid.Column="1"/>
</Grid>
Frame1 C# 业务代码
namespace WpfApp1
{
public partial class Frame1 : Window
{
public Frame1()
{
InitializeComponent();
}
private void Button_Click(object sender, RoutedEventArgs e) {
//获得page1 页面活动面板
Uri uri = new Uri("../Page1.xaml", UriKind.Relative);
framemain.Source = uri;
}
private void Button_Click1(object sender, RoutedEventArgs e)
{
//获得page2 页面活动面板
Uri uri = new Uri("../Page2.xaml", UriKind.Relative);
framemain.Source = uri;
}
}
}
Page1面板页
1 创建 Page1.xaml 设置
<Page x:Class="WpfApp1.Page1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:local="clr-namespace:WpfApp1"
mc:Ignorable="d"
d:DesignHeight="450" d:DesignWidth="800"
Title="Page1">
<Grid>
<Grid.RowDefinitions>
<RowDefinition />
<RowDefinition Height="50"/>
</Grid.RowDefinitions>
<Label FontSize="50" Grid.Row="0">Page1</Label>
<Button Grid.Row="1" Click="Button_Click">转移页面</Button>
</Grid>
</Page>
2 Page1.cs 使用 NavigationService.Navigate 面板之间跳转方法。
namespace WpfApp1{
public partial class Page1:Page{
public Page1(){
InitializeComponent();
}
private void Button_Click(object sender, RoutedEventArgs e)
{
var page2 = new Page2();//页面迁移功能
NavigationService.Navigate(page2);//跳转面板功能
}
}
}
Page2页面设置
<Page x:Class="WpfApp1.Page2"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:local="clr-namespace:WpfApp1"
mc:Ignorable="d"
d:DesignHeight="450" d:DesignWidth="800"
Title="Page2">
<Grid>
<Label FontSize="50">Page2</Label>
</Grid>
</Page>
Page2.cs 设置
namespace WpfApp1
{
public partial class Page2 : Page
{
public Page2()
{
InitializeComponent();
}
}
}
面板相互跳转效果
面板页面跳转page1–>page2
3 NavigationService 与Navigate 对象
Frame 中面板页面之间的跳转需要使用NavigationService 函数来控制。在PWF中在Frame和Page组件都可以使用NavigationService的对象中的Navigate函数来实现页面之间的迁移功能。
Navigate:Frame 中转发面板功能函数
-
frame.NavigationService.Navigate(new Uri(“Page1.xaml”, UriKind.Relative));
-
frame.NavigationService.Navigate(new Page1());
-
frame.NavigationService.Navigate(new Button());
-
frame.NavigationService.Navigate(“Hello world”);
其他函数
- frame.NavigationService.GoForward(); 前进
- frame.NavigationService.GoBack();回退
- frame.NavigationService.Refresh();刷新
3.1 构造方法转发页面
两个page相互之间和两个窗体之间传递参数,可以使用构造方法来进行传递。
page调用Navigate方法的时候通过构造方法来传递参数。在类中定义需要传递参数,在构造方法中作为参数定义出来。
-
创雅面板构造方法。
-
通过构造方法传递两个page面板之间的参数var page2 = new Page2(str);//创建面板类。
-
通过NavigationService.Navigate(page2);函数转发面板。
-
可以在面板构造方法中来设置面板的初始化参数。
在windon窗体中这个构造方法传递参数同样有效
namespace WpfApp1{
public partial class Page2 : Page{
public Page2(){
InitializeComponent();
}
//定义重其他页面要传递过来的参数
public string page1_value { get; set; }
public Page2(String test_str){//构造方法中获得者个参数
InitializeComponent();
this.test_str = test_str;
MessageBox.Show(this.test_str);
}
}
}
面板页面传参数例子
private void Button_Click(object sender, RoutedEventArgs e){
string str="我看到了";//定义Page2面板的初始化参数
var page2 = new Page2(str);//创建面板类
NavigationService.Navigate(page2);//转移面板页面
}
5.3.2 动态构造转发页面
通过Type获得面板对象所在文件位置,将Type强转成Page面板对象在调佣Navigate函数进行转发页面功能。
- 将面板类路径转成Type对象。Type.GetType(url)
- Type对象通过xaml代码的url路径反射生成类对象。type.Assembly.CreateInstance(url)
- 转发面板页面 NavigationService.Navigate(反射对象);
1 动态例子
<Button Grid.Row="1" Click="Button_Click" Tag="Page2">转移页面</Button>
private void Button_Click(object sender, RoutedEventArgs e){
Button sb = sender as Button;
Type type= Type.GetType("WpfApp1." + sb.Tag.ToString());//通过项目路径后台type对象
if (type!=null) {
//将Type强转成Page面板对象
object ojb = type.Assembly.CreateInstance("WpfApp1." + sb.Tag.ToString());
NavigationService.Navigate(ojb);//反射对象转发面板
MessageBox.Show("1111111111");
}
}
2 动态调用构造方法传参
在page1业务函数中定义一个页面转发事件函数
<Button Grid.Row="1" Click="Button_Click" Tag="Page2">转移页面</Button>
private void Button_Click(object sender, RoutedEventArgs e){
Type type = Type.GetType("WpfApp1.Page2");
//object[]参数放在数组中,进行动态构造
object[] par = { "我是参数" };
//指定要显示的page面板类和面板类的参数
object ojb = Activator.CreateInstance(type.Assembly.GetType("WpfApp1.Page2"),par);
NavigationService.Navigate(ojb);
}
page1 页面
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="200"></ColumnDefinition>
<ColumnDefinition Width="*"></ColumnDefinition>
</Grid.ColumnDefinitions>
<StackPanel Grid.Row="0" Grid.Column="0">
<Button Click="Button_Click">page</Button>-------对应c#中Button_Click转发方法
</StackPanel>
</Grid>
page2 页面
<Grid>
<StackPanel>
<Label FontSize="50">Page2</Label>
</StackPanel>
</Grid>
page2 代码
创建一个面板,定义一个带参数的构造方法获得参数。
public partial class Page2 : Page{
public string test_str { get; set; }
public Page2(){
InitializeComponent();
MessageBox.Show(this.test_str);
}
public Page2(String test_str){//定义一个带参数的构造方法
InitializeComponent();
this.test_str = test_str;
MessageBox.Show(this.test_str);
}
}
}
3.2 LoadCompleted事件传参
Page与Page页面转发需要传参时候,可以使用NavigationService.LoadCompleted函数事件来传参数,在page2页面中目标页面中定义NavigationService_LoadCompleted(object sender, NavigationEventArgs e)函数事件(页面转发事件初始化),在page1中转发时候将this.NavigationService.LoadCompleted += page2.NavigationService_LoadCompleted;两个事件绑定,通过NavigationService_LoadCompleted函数来完成参数传递。 在使用 NavigationService.Navigate(page2, “我是参数”)第二参数来完成参数设置。
- 目标页面中定义 NavigationService_LoadCompleted 事件
- 发送页面中绑定 this.NavigationService.LoadCompleted += page2.NavigationService_LoadCompleted;事件
- NavigationService.Navigate(page2, “我是参数”);设置参数
Page1 cs中页面转发事件
private void Button_Click(object sender, RoutedEventArgs e){
var page2 = new Page2();
//页面转发初始化事件绑定
this.NavigationService.LoadCompleted += page2.NavigationService_LoadCompleted;
//Navigate 第二个参数为 page传递的参数内容
NavigationService.Navigate(page2, "我是参数");
}
Page2 cs
namespace WpfApp1{
public partial class Page2 : Page{
public string test_str { get; set; }
public Page2(){
InitializeComponent();
MessageBox.Show(test_str);
}
// 设置页面初始化事件
//page1 页面中this.NavigationService.LoadCompleted调用的页面初始化事件
public void NavigationService_LoadCompleted(object sender, NavigationEventArgs e){
if (e.ExtraData!=null) {
//获得 NavigationService.Navigate(page2, "我是参数");中的参数
//参数是 this.test_str=我是参数
string str = (string)e.ExtraData;
this.test_str = str;
}
}
}
}
4 NavigationWindow 弹出页面
NavigationWindow是一种顶层浏览器窗体,它与Frame 区别在Frame 是局部刷新page面板,NavigationWindow是全局刷新面板。
NavigationWindow窗体不用直接使用,需要定义Source它承载的面板页面内容。
<NavigationWindow x:Class="WpfApp1.布局.NavigationWindow1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfApp1.布局"
mc:Ignorable="d" ShowsNavigationUI="False" Source="../Page1.xaml"
Title="NavigationWindow1" Height="450" Width="800">
</NavigationWindow>
访问窗体方法
NavigationWindow1 win1 = new NavigationWindow1();
win1.Show();
NavigationWindow 重载方法
- Navigate(Uri)
- Navigate(Uri, Object)
5 StaticResource 静态资源
在 WPF中多个 UI 元素之间共享一个对象或者资源的情况。比如你有多个按钮,每个按钮设置了相同的背景颜色,如果每次改变背景颜色都重新设置所有按钮的背景颜色。现在定义了一个资源,这个资源可以被多个控件共享,所以如果发生变化,你只需要更改一个地方,它就会反映在所有控件中。StaticResource 用于设置这些静态资源元素。
StaticResource 分为三种,窗体资源和面板资源,全局资源。
- Window.Resources
- Page.Resources
- Application.Resources
Resources 位子
Resources一定要定义在布局组件上边。
<Window x:Class="WpfApp1.MainWindow"
Title="MainWindow" Height="450" Width="800">
<Window.Resources>
//资源部分内容
</Window.Resources>
<Grid>
</Grid>
</Window>
Resources设置
将资源定义在 Window.Resources 中,在SolidColorBrush元素中描述定义的内容。例如:要定义颜色,请为 SolidColorBrush 设置 Key 和 Color
<Window.Resources>
<SolidColorBrush x:Key="ButtonBackColor" Color="DarkBlue"/>
<SolidColorBrush x:Key="ButtonForeColor" Color="White"/>
</Window.Resources>
Resource 在组件中的引用方式
使用资源关键字StaticResource来指定资源的key值
Background="{StaticResource ButtonBackColor}"---ButtonBackColor 对应资源key
Resource使用例子
Resource使用的时候一定要分清楚使用所在的对象是Window 还是page,引用的方式是不一样的。
<Window x:Class="MainWindow"
........
Title="MainWindow" Height="300" Width="300"
<Window.Resources>--------------窗体中使用
<SolidColorBrush x:Key="ButtonBackColor" Color="DarkBlue"/>
<SolidColorBrush x:Key="ButtonForeColor" Color="White"/>
</Window.Resources>
<StackPanel>
<Label x:Name="lblHello" >欢迎你光临韬哥WPF代码世界!</Label>
<Button Name="btnThd1"
Click="Frame_Click"
Background="{StaticResource ButtonBackColor}"-------引用Resources静态资源
Foreground="{StaticResource ButtonForeColor}">
Frame测试1</Button>
</StackPanel>
</Window>
Page面板中使用
<Page x:Class="MainWindow"
........
Title="MainWindow" Height="300" Width="300"
<Window.Page>--------------面板中使用
<SolidColorBrush x:Key="ButtonBackColor" Color="DarkBlue"/>
<SolidColorBrush x:Key="ButtonForeColor" Color="White"/>
</Window.Page>
<StackPanel>
<Label x:Name="lblHello" >欢迎你光临韬哥WPF代码世界!</Label>
<Button Name="btnThd1"
Click="Frame_Click"
Background="{StaticResource ButtonBackColor}"-------引用Resources静态资源
Foreground="{StaticResource ButtonForeColor}">
Frame测试1</Button>
</StackPanel>
</Page>
效果图
全局资源
在App.xaml文件的Application.Resources区域定义资源。
在项目工程中的用App.xaml定义资源
<Application x:Class="WPF006_2.App"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:WPF006_2"
StartupUri="MainWindow.xaml">
<Application.Resources>
<SolidColorBrush x:Key="backColor" Color="Orange"/>
</Application.Resources>
</Application>
在项目中的其他窗体与面板组件中都可以使用到这个资源。
<Window x:Class="MainWindow"
......
Title="MainWindow" Height="300" Width="300">
<Window.Resources>
</Window.Resources>
<Grid>
<StackPanel>
<Button Content="mainWindow"
FontSize="40"
Background="{StaticResource backColor}"-----------在对应的窗体和面板中引入资源
/>
</StackPanel>
</Grid>
</Window>
6 资源 Style
资源 Style 样式分类2个类型定义属性和事件。包含在
- 属性:
- 事件:
<Style TargetType="Button">
<Style.Triggers>
<Setter Property="ToolTip" Value="这是一个可见按钮"></Setter>
<EventSetter Event="Click" Handler="ButtonOnClick" />
</Style.Triggers>
</Style>
更多推荐
所有评论(0)