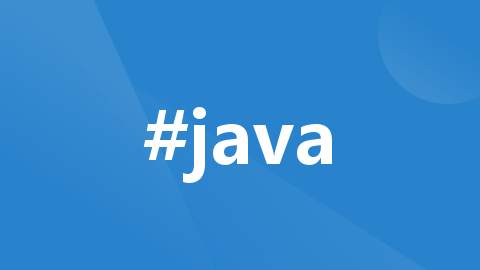
Stream——替换集合中的某些值
·
简单类型集合修改值
准备一个简单的数据集合,如:List<String>
,如下所示:
List<String> stringList = Arrays.asList("2023-01-08",
"2023-02-08",
"2023-03-08",
"2023-04-08",
"2023-05-08");
需要实现的功能:
将
yyyy-MM-dd
转换为yyyy/MM/dd
的样式。
此时使用stream
,则可以采取map()
进行操作,如下所示:
List<String> stringList = Arrays.asList("2023-01-08", "2023-02-08", "2023-03-08", "2023-04-08","2023-05-08");
List<String> collect = stringList.stream().filter(x -> x != null && x != "").map(x -> {
DateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
try {
Date date = dateFormat.parse(x);
DateFormat format = new SimpleDateFormat("yyyy/MM/dd");
return format.format(date);
} catch (ParseException e) {
e.printStackTrace();
}
return x;
}).collect(Collectors.toList());
collect.stream().forEach(e-> System.out.println(e));
数据打印后,效果如下所示:
2023/01/08
2023/02/08
2023/03/08
2023/04/08
2023/05/08
当然,也可以采取peek()
方式,如下所示:
List<String> stringList = Arrays.asList("2023-01-08",
"2023-02-08",
"2023-03-08",
"2023-04-08",
"2023-05-08");
List<String> collect = stringList.stream().filter(x -> x != null && x != "").peek(x -> {
DateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
try {
Date date = dateFormat.parse(x);
DateFormat format = new SimpleDateFormat("yyyy/MM/dd");
format.format(date);
} catch (ParseException e) {
e.printStackTrace();
}
}).collect(Collectors.toList());
collect.stream().forEach(e-> System.out.println(e));
对象集合,修改对象中的某个属性
依旧准备一个对象集合,不过对象很简单,如下所示:
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
@Data
@AllArgsConstructor
@NoArgsConstructor
class User {
private String dates;
}
List<User> users = Arrays.asList(new User("2023-01-08")
,new User("2023-02-08")
,new User("2023-03-08")
,new User("2023-04-08")
,new User("2023-05-08"));
依旧是按照上面的格式进行转换,此时需要使用到peek
,如下所示:
List<User> users = Arrays.asList(new User("2023-01-08")
,new User("2023-02-08")
,new User("2023-03-08")
,new User("2023-04-08")
,new User("2023-05-08"));
List<User> collect = users.stream().filter(x -> x.getDates() != null && x.getDates() != "").peek(x -> {
String dates = x.getDates();
DateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
Date parse = null;
try {
parse = dateFormat.parse(dates);
DateFormat format = new SimpleDateFormat("yyyy/MM/dd");
x.setDates(format.format(parse));
} catch (ParseException e) {
e.printStackTrace();
}
}).collect(Collectors.toList());
collect.stream().forEach(e-> System.out.println(e));
执行后,打印效果如下所示:
User(dates=2023/01/08)
User(dates=2023/02/08)
User(dates=2023/03/08)
User(dates=2023/04/08)
User(dates=2023/05/08)
如果使用map()
呢?可以如下面这种方式做:
List<User> users = Arrays.asList(new User("2023-01-08")
,new User("2023-02-08")
,new User("2023-03-08")
,new User("2023-04-08")
,new User("2023-05-08"));
List<User> collect = users.stream().filter(x -> x.getDates() != null && x.getDates() != "").map(x -> {
String dates = x.getDates();
DateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
Date parse = null;
try {
parse = dateFormat.parse(dates);
DateFormat format = new SimpleDateFormat("yyyy/MM/dd");
x.setDates(format.format(parse));
} catch (ParseException e) {
e.printStackTrace();
}
return x;
}).collect(Collectors.toList());
collect.stream().forEach(e-> System.out.println(e));
map 与 peek 的区别
从方法结构上看,map有一个返回类型,peek为void,只操作原stream中的各个元数据对象。
- map
Stream map(Function<? super T, ? extends R> mapper);
- peek
Stream peek(Consumer<? super T> action);
更多推荐
所有评论(0)