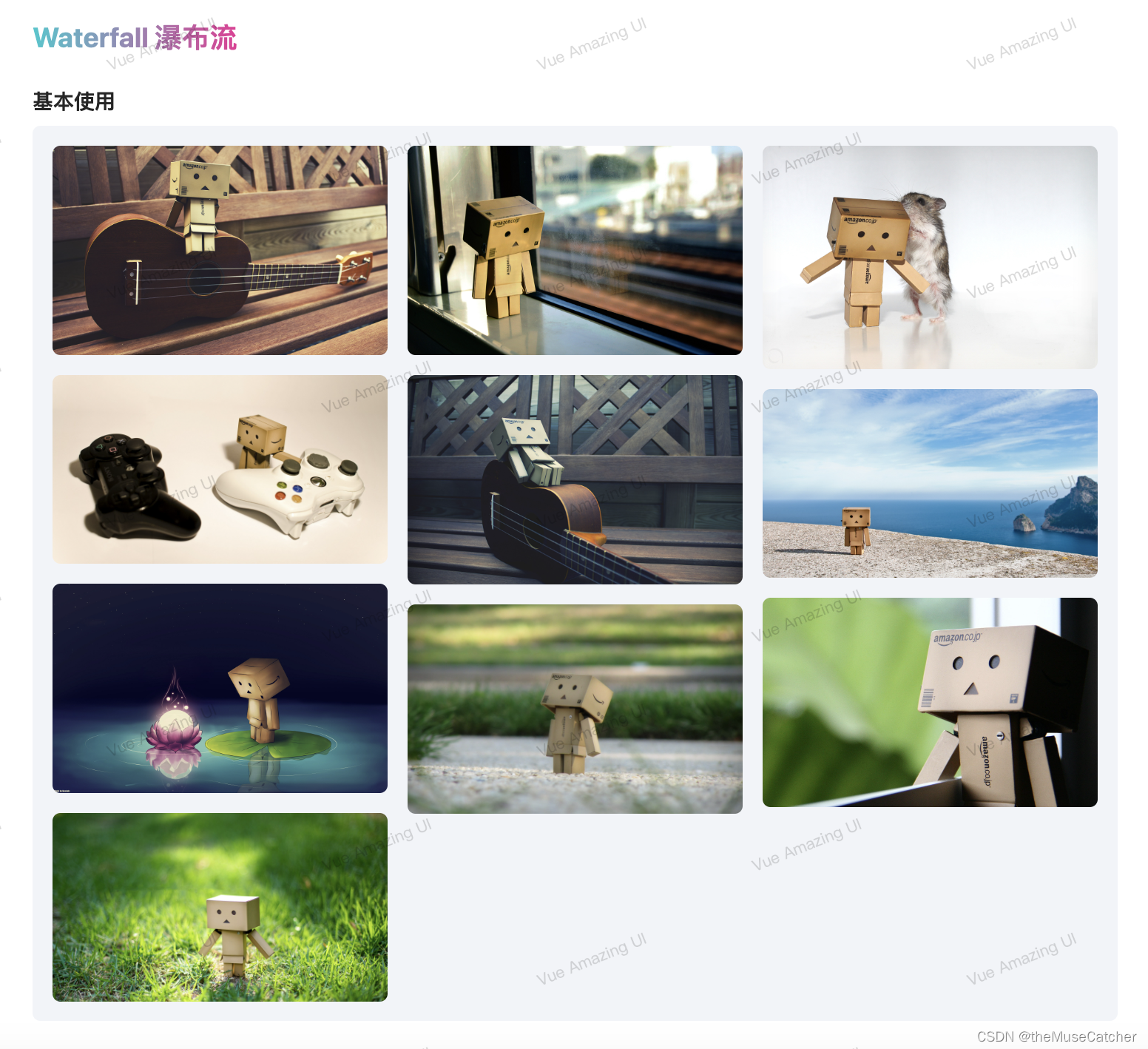
Vue3瀑布流(Waterfall)
Vue3+TS使用JS计算方式实现的瀑布流图片展示组件。

一键AI生成摘要,助你高效阅读
问答
·
可自定义设置以下属性:
-
图片数组(images),类型:Array<{title?: string, src: string}>,默认 []
-
要划分的列数(columnCount),类型:number,默认 3
-
各列之间的间隙(columnGap),类型:number,单位px,默认 30
-
瀑布流区域的总宽度(width),类型:number | string,默认 '100%'
-
瀑布流区域和图片圆角(borderRadius),类型:number,单位px,默认 8
-
瀑布流区域背景填充色(backgroundColor),类型:string,默认 '#F2F4F8'
效果如下图:在线预览
其中引用组件:Vue3加载中(Spin)
①创建瀑布流组件Waterfall.vue:
<script setup lang="ts">
import { ref, shallowRef, computed, watch, watchPostEffect } from 'vue'
import Spin from '../spin'
/*
宽度固定,图片等比例缩放;使用JS获取每张图片宽度和高度,结合 `relative` 和 `absolute` 定位
计算每个图片的位置 `top`,`left`,保证每张新的图片都追加在当前高度最小的那列末尾
*/
interface Image {
src: string // 图片地址
title?: string // 图片名称
}
interface Props {
images: Image[] // 图片数组
columnCount?: number // 要划分的列数
columnGap?: number // 各列之间的间隙,单位px
width?: string|number // 瀑布流区域的总宽度
borderRadius?: number // 瀑布流区域和图片圆角,单位px
backgroundColor?: string // 瀑布流区域背景填充色
}
const props = withDefaults(defineProps<Props>(), {
images: () => [],
columnCount: 3,
columnGap: 20,
width: '100%',
borderRadius: 8,
backgroundColor: '#F2F4F8'
})
const imagesProperty = ref<any[]>([])
const preColumnHeight = ref<number[]>(Array(props.columnCount).fill(0)) // 每列的高度
const waterfall = shallowRef() // ref() 的浅层作用形式
const imageWidth = ref()
const totalWidth = computed(() => {
if (typeof props.width === 'number') {
return props.width + 'px'
} else {
return props.width
}
})
const height = computed(() => {
return Math.max(...preColumnHeight.value) + props.columnGap
})
const len = computed(() => {
return props.images.length
})
const loaded = ref(Array(len.value)) // 图片是否加载完成
const rerender = ref(false)
watch(
() => [props.columnCount, props.columnGap, props.width],
() => {
rerender.value = true
preColumnHeight.value = Array(props.columnCount).fill(0)
onPreload()
},
{
deep: true, // 强制转成深层侦听器
flush: 'post' // 在侦听器回调中访问被 Vue 更新之后的 DOM
}
)
watchPostEffect(() => {
if (props.images.length) {
onPreload()
}
})
async function onPreload () { // 计算图片宽高和位置(top,left)
// 计算每列的图片宽度
imageWidth.value = (waterfall.value.offsetWidth - (props.columnCount + 1) * props.columnGap) / props.columnCount
imagesProperty.value.splice(0)
for (let i = 0; i < len.value; i++) {
await loadImage(props.images[i].src, i)
}
}
function loadImage (url: string, n: number) {
return new Promise((resolve) => {
const image = new Image()
image.src = url
image.onload = function () { // 图片加载完成时执行,此时可通过image.width和image.height获取到图片原始宽高
if (!rerender.value) {
loaded.value[n] = false
}
var height = image.height / (image.width / imageWidth.value)
imagesProperty.value[n] = { // 存储图片宽高和位置信息
width: imageWidth.value,
height: height,
...getPosition(n, height)
}
resolve('load')
}
})
}
function getPosition (i: number, height: number) { // 获取图片位置信息(top,left)
if (i < props.columnCount) {
preColumnHeight.value[i] = props.columnGap + height
return {
top: props.columnGap,
left: (imageWidth.value + props.columnGap) * i + props.columnGap
}
} else {
const top = Math.min(...preColumnHeight.value)
let index = 0
for (let n = 0; n < props.columnCount; n++) {
if (preColumnHeight.value[n] === top) {
index = n
break
}
}
preColumnHeight.value[index] = top + props.columnGap + height
return {
top: top + props.columnGap,
left: (imageWidth.value + props.columnGap) * index + props.columnGap
}
}
}
function onLoaded (index: number) {
loaded.value[index] = true
}
</script>
<template>
<div class="m-waterfall" ref="waterfall" :style="`--borderRadius: ${borderRadius}px; background-color: ${backgroundColor}; width: ${totalWidth}; height: ${height}px;`">
<Spin
v-show="loaded[index]!==undefined"
class="m-image"
:style="`width: ${property.width}px; height: ${property.height}px; top: ${property && property.top}px; left: ${property && property.left}px;`"
:spinning="!loaded[index]"
size="small"
indicator="dynamic-circle"
v-for="(property, index) in imagesProperty" :key="index">
<img
class="u-image"
:src="images[index].src"
:alt="images[index].title"
@load="onLoaded(index)" />
</Spin>
</div>
</template>
<style lang="less" scoped>
.m-waterfall {
position: relative;
border-radius: var(--borderRadius);
.m-image {
position: absolute;
.u-image {
width: 100%;
height: 100%;
border-radius: var(--borderRadius);
display: inline-block;
vertical-align: bottom;
}
}
}
</style>
②在要使用的页面引入:
<script setup lang="ts">
import Waterfall from './Waterfall.vue'
import { ref, onBeforeMount } from 'vue'
import { getImageUrl } from '@/utils/util'
const images = ref<any[]>([])
function loadImages () {
for (let i = 1; i <= 10; i++) {
images.value.push({
title: `image-${i}`,
link: '',
src: `https://cdn.jsdelivr.net/gh/themusecatcher/resources@0.0.5/${i}.jpg`
})
}
}
onBeforeMount(() => { // 组件已完成响应式状态设置,但未创建DOM节点
loadImages()
})
</script>
<template>
<div>
<h1>Waterfall 瀑布流</h1>
<h2 class="mt30 mb10">基本使用</h2>
<Waterfall :images="images" :width="1100" />
<h2 class="mt30 mb10">自定义展示</h2>
<Waterfall
:images="images"
:width="1100"
:column-count="4"
:column-gap="10"
background-color="#e1faeb"
:border-radius="6" />
</div>
</template>
更多推荐
所有评论(0)