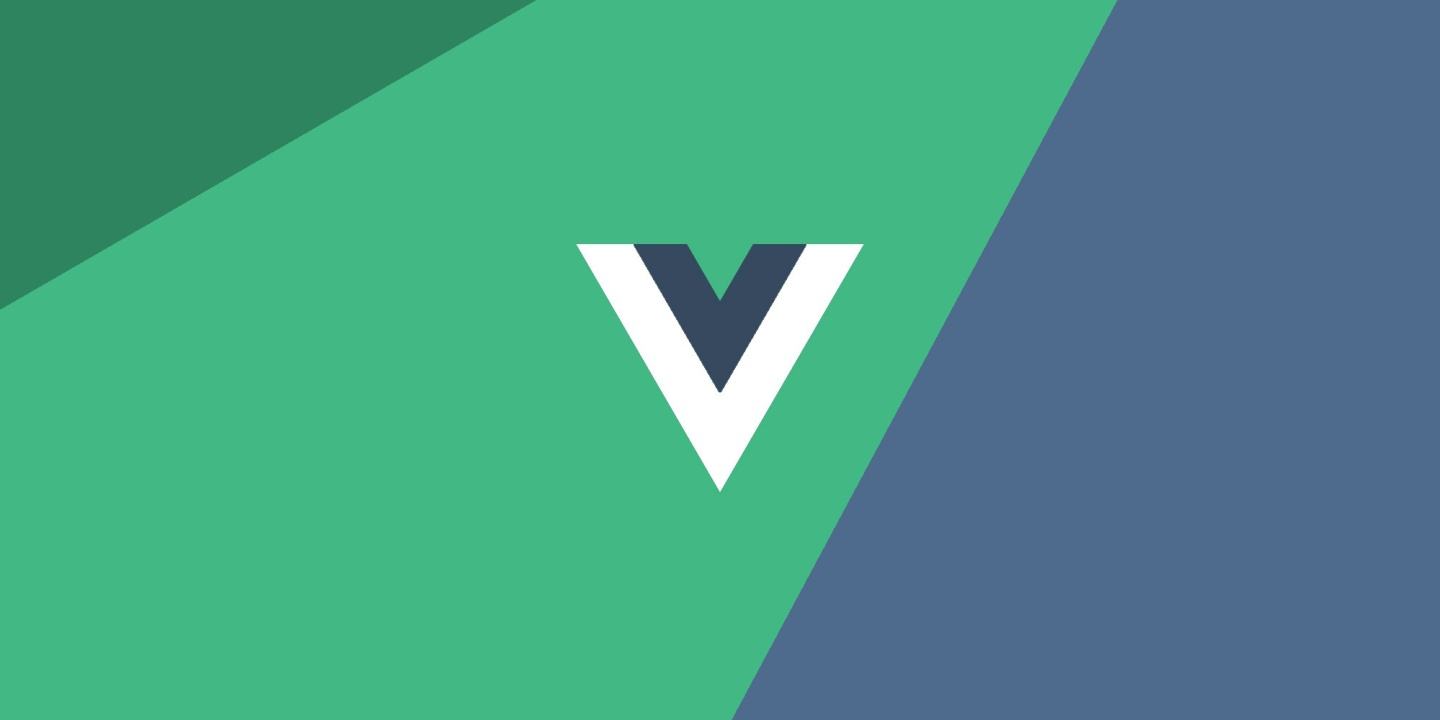
el+vue 实战 ⑧ el-calendar日历组件设置点击事件、el-calendar日历组件设置高度、el-calendar日历组件自定义日历内部内容
·
一、效果图
日历显示内容变为01,02的形式,点击相应的日期后,有一个弹出框显示当天完成的一些内容。
二、前端代码设置
<el-calendar v-model="value">
<div
slot="dateCell"
slot-scope="{ data }"
@click="viewDayWork(data)"
v-popover:popover
>
<p>
{{ data.day.split("-").slice(2).join() }}
</p>
</div>
</el-calendar>
① 其中value在script中定义如下:
<script>
export default {
data() {
return {
value: new Date(),
};
},
};
</script>
② 日历组件的data的结构如下:
③ {{ data.day.split("-").slice(2).join() }}对应输出
// 2022-06-09
console.log(data.day);
// ["2022","06","09"]
console.log(data.day.split("-"));
// ["09"]
console.log(data.day.split("-").slice(2));
// 09
console.log(data.day.split("-").slice(2).join());
④ v-popover:popover绑定一个弹出框
弹出框代码如下,ref绑定的名称同上面命名一样即可。
<el-popover
placement="bottom"
ref="popover"
width="200"
trigger="manual"
v-model="visible"
/>
⑤ 调整日历组件的宽高,样式设置
/deep/.el-calendar-table .el-calendar-day {
height: 50px !important;
}
三、组件整体代码:
<template>
<div>
<!--侧边栏-日历部分 -->
<el-row :span="6" style="margin-top: 1rem">
<el-card>
<el-calendar v-model="value">
<div
slot="dateCell"
slot-scope="{ data }"
@click="viewDayWork(data)"
v-popover:popover
>
<p>
{{ data.day.split("-").slice(2).join()}}
</p>
</div>
</el-calendar>
</el-card>
</el-row>
<!-- 日历部分弹出框 -->
<el-popover
placement="bottom"
ref="popover"
width="200"
trigger="manual"
v-model="visible"
>
<el-card>
<div>日期:{{ this.timeValue }}</div>
<div>
<div>完成任务:</div>
<div v-if="!this.isCalInput">{{ this.timeNote }}</div>
<el-input
v-if="this.isCalInput"
type="textarea"
autosize
placeholder="请输入内容"
v-model="calText"
>
</el-input>
</div>
<el-button
v-if="!this.isCalInput"
type="primary"
icon="el-icon-edit"
circle
size="mini"
class="calendar-edit-bth"
@click="onEditCal"
/>
<el-button
v-if="this.isCalInput"
type="success"
icon="el-icon-check"
circle
size="mini"
class="calendar-edit-bth"
@click="handleCalCommit"
/>
<el-button
v-if="this.isCalInput"
type="warning"
icon="el-icon-refresh-left"
circle
size="mini"
class="calendar-reset-bth"
@click="handleCalReset"
/>
</el-card>
</el-popover>
</div>
</template>
<script>
export default {
data() {
return {
value: new Date(),
timeValue: "",
timeNote: "",
timeId: null,
visible: false,
isCalInput: false,
calText: "",
updateCal: {
id: "",
calendarVal: "",
calendarNote: "",
},
};
},
created() {},
methods: {
// 日历-时间格式转换【没用到】
timeFormateChange(value) {
let time;
let yy = value.getFullYear();
let mm = value.getMonth() + 1;
let dd = value.getDate();
time = yy + "-" + mm + "-" + dd;
this.timeValue = time;
},
// 日历-处理点击查看日历日期事件
async viewDayWork(data) {
// 日历事件和ID清零,日期选择为选择的日期
this.timeNote = "";
this.timeValue = data.day;
this.timeId = null;
// 点击之前状态为没查看
if (!this.visible) {
// 后端url地址
let url = "后端接口地址";
// 携带当前日期查询
const res = await this.$http.get(url, {
params: {
calendarVal: this.timeValue,
},
});
// 接取后端返回的当前日期的事件
if (res.data.data[0] != null) {
this.timeNote = res.data.data[0].calendarNote;
this.timeId = res.data.data[0].id;
}
}
// 事件点击状态改变
this.visible = !this.visible;
// 如果点击编辑后再点退出,则编辑状态需要回退
if (this.isCalInput) {
this.isCalInput = false;
}
},
// 日历-日历点击编辑事件
onEditCal() {
// 输入框显示
this.isCalInput = true;
// 当前输入框内容和原来日历部分内容相同
this.calText = this.timeNote;
},
// 日历-处理日历编辑事件
async handleCalCommit() {
// timeId不为空【数据库中存在这天的数据】,执行更新操作
if (this.timeId != null) {
// 处理传入后端的数据
this.updateCal.calendarVal = this.timeValue;
this.updateCal.calendarNote = this.calText;
this.updateCal.id = this.timeId;
// 后端接口地址
let url = "后端接口地址";
// 接收接口返回信息
const res = await this.$http.post(url, this.updateCal);
if (res.data.code == 200) {
this.$message({
message: "更新任务成功!",
type: "success",
});
}
// 显示当前日历任务信息
this.timeNote = res.data.data.calendarNote;
// 清空传入后端的数据对象
this.updateCal = this.$options.data().updateCal;
}
// timeId为空【数据库中不存在这天的数据】,执行新增操作
else {
// 放入待新增的日历日期和任务
this.updateCal.calendarVal = this.timeValue;
this.updateCal.calendarNote = this.calText;
// 后端接口地址
let url = "后端接口地址";
// 接收处理后端返回的信息
const res = await this.$http.post(url, this.updateCal);
if (res.data.code == 200) {
this.$message({
message: "新增任务成功!",
type: "success",
});
}
// 返回的信息接取
this.timeNote = res.data.data.calendarNote;
// 清空传入的数组
this.updateCal = this.$options.data().updateCal;
//
this.timeId = null;
}
// 更改正在编辑的状态
this.isCalInput = false;
},
// 处理日历取消编辑事件
handleCalReset() {
this.isCalInput = false;
},
},
watch: {},
};
</script>
<style scoped lang="less">
/deep/.el-calendar-table .el-calendar-day {
height: 50px !important;
}
.calendar-edit-bth {
margin-top: 3px;
float: right;
margin-bottom: 2px;
}
.calendar-reset-bth {
margin-top: 3px;
float: right;
margin-bottom: 2px;
margin-right: 4px;
}
</style>
四、总结
后面这个再慢慢优化吧,前端属实写着比后端有趣,但是后端提升空间大,钱更多😭
更多推荐
所有评论(0)