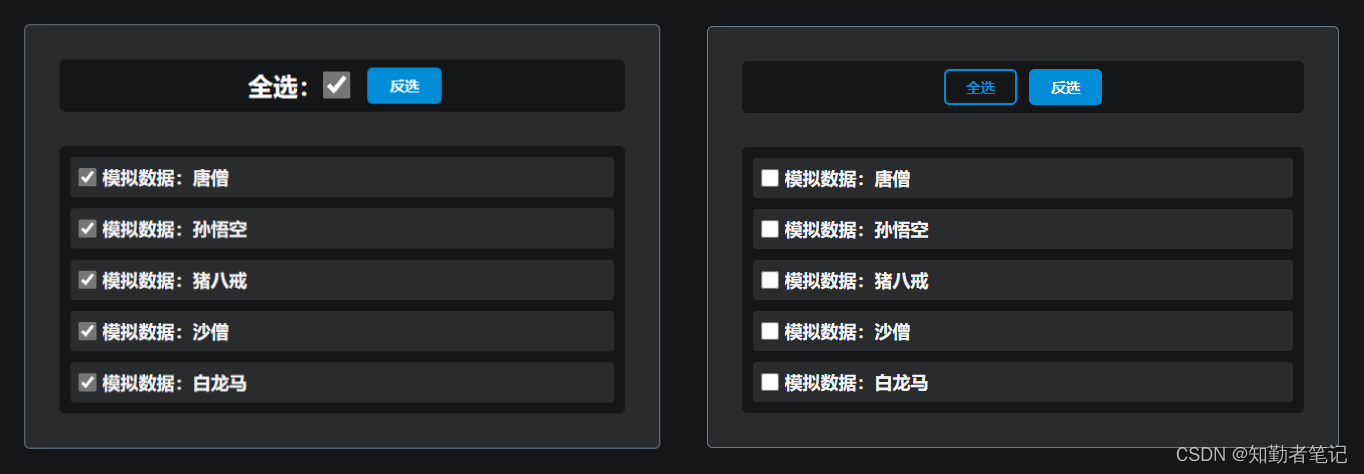
vue实现“全选/全不选/反选”的三个方法
【代码】vue实现“全选/全不选/反选”的三个方法。

一键AI生成摘要,助你高效阅读
问答
·
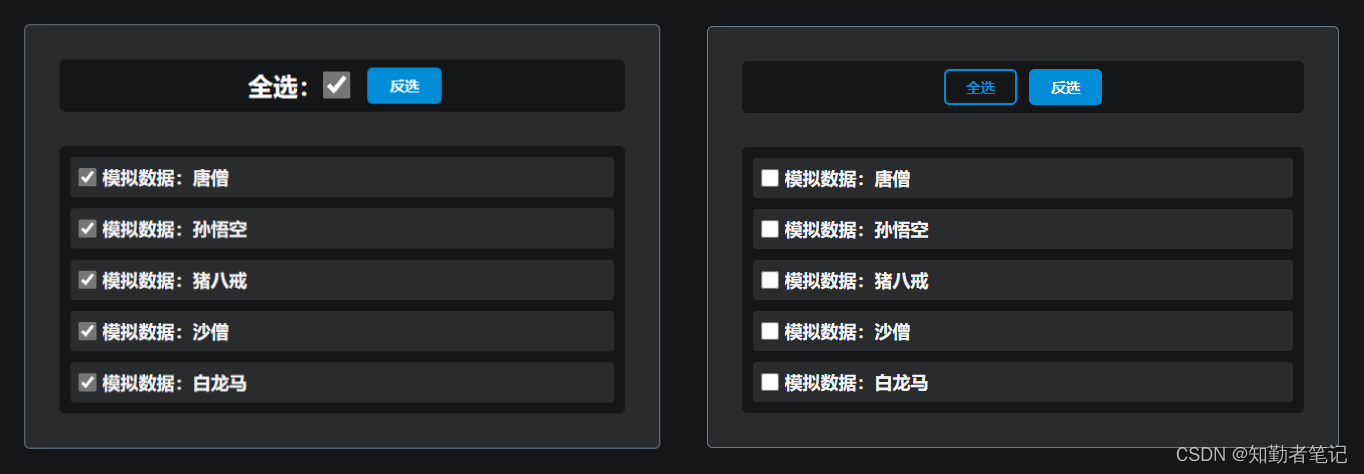
第一个方法:使用vue的计算属性
<template>
<main>
<div class="region">
<div class="regionOperation">
<div class="operationList" :class="{ selectNoneoperationList: isAllSelect }">
<div class="selectAllContainer">
<span>全选:</span>
<input type="checkbox" v-model="isAllSelect">
</div>
<button class="reverseSelectionButton" @click.prevent="ReverseSelection">反选</button>
</div>
</div>
<ul class="optionList">
<li v-for="(value, key) in SimulationData" :key="key">
<input type="checkbox" v-model="value.isSelect" @change="SelectOrCancel">
<span>{{ value.dataContent }}</span>
</li>
</ul>
</div>
</main>
</template>
<style lang="less">
main {
height: 100vh;
display: flex;
align-items: center;
justify-content: center;
background-color: #151617;
.region {
width: 500px;
padding: 30px;
border-radius: 5px;
background-color: #2a2b2c;
border: 1px solid #6a7b8c;
box-shadow: 0px 0px 19px -5px rgb(0 0 0 / 25%);
-webkit-box-shadow: 0px 0px 19px -5px rgb(0 0 0 / 25%);
.regionOperation,
.optionList {
width: 100%;
padding: 10px;
border-radius: 5px;
box-sizing: border-box;
background-color: #151617;
}
.regionOperation {
height: 46px;
.operationList {
width: 200px;
height: 100%;
margin: 0px auto;
display: flex;
align-items: center;
justify-content: center;
.selectAllContainer,
.reverseSelectionButton {
color: #ffffff;
font-weight: bold;
}
.selectAllContainer {
width: 100px;
margin-right: 5px;
font-size: 22px;
display: flex;
align-items: center;
justify-content: center;
background-color: #151617;
input[type='checkbox'] {
width: 25px;
height: 25px;
}
}
.reverseSelectionButton {
outline: none;
font-size: 13px;
cursor: pointer;
margin-left: 5px;
padding: 6px 18px;
border-radius: 5px;
transition: 0.3s ease;
border: 2px solid #008cd6;
background-color: #008cd6;
}
}
}
.optionList {
margin-top: 30px;
li {
width: 100%;
padding: 7px;
display: flex;
border-radius: 3px;
align-items: center;
box-sizing: border-box;
background-color: #2a2b2c;
input[type='checkbox'] {
width: 16px;
height: 16px;
}
span {
margin-left: 5px;
font-weight: bold;
}
}
li+li {
margin-top: 10px;
}
}
}
}
</style>
<script>
export default {
data () {
return {
SimulationData: [
{
dataContent: '模拟数据:唐僧',
isSelect: false
},
{
dataContent: '模拟数据:孙悟空',
isSelect: false
},
{
dataContent: '模拟数据:猪八戒',
isSelect: false
},
{
dataContent: '模拟数据:沙僧',
isSelect: false
},
{
dataContent: '模拟数据:白龙马',
isSelect: false
}
]
}
},
computed: { // 计算属性
// isAllSelect: function () {
// // 作用:监听选项框状态 -> 全选状态
// // every() 查找数组里不符合条件的数据,如果有查找到直接返回 false
// return this.SimulationData.every(SimulationDataAllSelect => SimulationDataAllSelect.isSelect === true)
// }
isAllSelect: {
set (value) {
// 作用:获取全选框的状态(true/false),然后修改选项框状态
this.SimulationData.forEach(SimulationDataAllSelect => { SimulationDataAllSelect.isSelect = value })
},
get () {
// 作用:监听选项框状态 -> 全选状态
// every() 查找数组里不符合条件的数据,如果有查找到直接返回 false
return this.SimulationData.every(SimulationDataAllSelect => SimulationDataAllSelect.isSelect === true)
}
}
},
methods: {
ReverseSelection: function () {
this.SimulationData.forEach(SimulationDataAllSelect => {
SimulationDataAllSelect.isSelect = !SimulationDataAllSelect.isSelect
})
}
}
}
</script>
第二个方法:使用every方法
<template>
<main>
<div class="region">
<div class="regionOperation">
<div class="buttonList" :class="{ selectNoneButtonList: isAllSelect }">
<button class="selectAllButton" :class="{ selectNoneButton: isAllSelect }"
@click.prevent="SelectAllOrCancelAll">
<span v-show="!isAllSelect">全选</span>
<span v-show="isAllSelect">全不选</span>
</button>
<button class="reverseSelectionButton" @click.prevent="ReverseSelection">反选</button>
</div>
</div>
<ul class="optionList">
<li v-for="(value, key) in SimulationData" :key="key">
<input type="checkbox" v-model="value.isSelect" @change="SelectOrCancel">
<span>{{ value.dataContent }}</span>
</li>
</ul>
</div>
</main>
</template>
<style lang="less">
main {
height: 100vh;
display: flex;
align-items: center;
justify-content: center;
background-color: #151617;
.region {
width: 500px;
padding: 30px;
border-radius: 5px;
background-color: #2a2b2c;
border: 1px solid #6a7b8c;
box-shadow: 0px 0px 19px -5px rgb(0 0 0 / 25%);
-webkit-box-shadow: 0px 0px 19px -5px rgb(0 0 0 / 25%);
.regionOperation,
.optionList {
width: 100%;
padding: 10px;
border-radius: 5px;
box-sizing: border-box;
background-color: #151617;
}
.regionOperation {
height: 46px;
.buttonList {
width: 150px;
height: 100%;
margin: 0px auto;
display: flex;
align-items: center;
justify-content: center;
.selectAllButton,
.reverseSelectionButton {
outline: none;
cursor: pointer;
font-size: 13px;
font-weight: bold;
padding: 6px 18px;
border-radius: 5px;
transition: 0.3s ease;
border: 2px solid #008cd6;
}
.selectAllButton {
color: #008cd6;
margin-right: 5px;
background-color: #151617;
}
.selectNoneButton,
.reverseSelectionButton {
color: #ffffff;
margin-left: 5px;
background-color: #008cd6;
}
}
.selectNoneButtonList {
width: 161px;
}
}
.optionList {
margin-top: 30px;
li {
width: 100%;
padding: 7px;
display: flex;
border-radius: 3px;
align-items: center;
box-sizing: border-box;
background-color: #2a2b2c;
input[type='checkbox'] {
width: 16px;
height: 16px;
}
span {
margin-left: 5px;
font-weight: bold;
}
}
li+li {
margin-top: 10px;
}
}
}
}
</style>
<script>
export default {
data () {
return {
SimulationData: [
{
dataContent: '模拟数据:唐僧',
isSelect: false
},
{
dataContent: '模拟数据:孙悟空',
isSelect: false
},
{
dataContent: '模拟数据:猪八戒',
isSelect: false
},
{
dataContent: '模拟数据:沙僧',
isSelect: false
},
{
dataContent: '模拟数据:白龙马',
isSelect: false
}
],
isAllSelect: false
}
},
methods: {
SelectOrCancel: function (itme) {
// itme.isSelect = !itme.isSelect // input标签不需要这句
this.isAllSelect = this.SimulationData.every(item => item.isSelect)
},
SelectAllOrCancelAll: function () {
// 优化前
// if (this.isAllSelect === true) {
// this.SimulationData.forEach(SimulationDataAllSelect => { SimulationDataAllSelect.isSelect = false })
// this.isAllSelect = false
// } else {
// this.SimulationData.forEach(SimulationDataAllSelect => { SimulationDataAllSelect.isSelect = true })
// this.isAllSelect = true
// }
// 优化后
this.SimulationData.forEach(SimulationDataAllSelect => {
SimulationDataAllSelect.isSelect = !this.isAllSelect
})
this.isAllSelect = !this.isAllSelect
},
ReverseSelection: function () {
this.SimulationData.forEach(SimulationDataAllSelect => {
SimulationDataAllSelect.isSelect = !SimulationDataAllSelect.isSelect
this.SelectOrCancel(SimulationDataAllSelect)
})
}
}
}
</script>
第三个方法:使用filter方法
<template>
<main>
<div class="region">
<div class="regionOperation">
<div class="buttonList" :class="{ selectNoneButtonList: isAllSelect }">
<button class="selectAllButton" :class="{ selectNoneButton: isAllSelect }"
@click.prevent="SelectAllOrCancelAll">
<span v-show="!isAllSelect">全选</span>
<span v-show="isAllSelect">全不选</span>
</button>
<button class="reverseSelectionButton" @click.prevent="ReverseSelection">反选</button>
</div>
</div>
<ul class="optionList">
<li v-for="(value, key) in SimulationData" :key="key">
<input type="checkbox" v-model="value.isSelect" @change="SelectOrCancel">
<span>{{ value.dataContent }}</span>
</li>
</ul>
</div>
</main>
</template>
<style lang="less">
main {
height: 100vh;
display: flex;
align-items: center;
justify-content: center;
background-color: #151617;
.region {
width: 500px;
padding: 30px;
border-radius: 5px;
background-color: #2a2b2c;
border: 1px solid #6a7b8c;
box-shadow: 0px 0px 19px -5px rgb(0 0 0 / 25%);
-webkit-box-shadow: 0px 0px 19px -5px rgb(0 0 0 / 25%);
.regionOperation,
.optionList {
width: 100%;
padding: 10px;
border-radius: 5px;
box-sizing: border-box;
background-color: #151617;
}
.regionOperation {
height: 46px;
.buttonList {
width: 150px;
height: 100%;
margin: 0px auto;
display: flex;
align-items: center;
justify-content: center;
.selectAllButton,
.reverseSelectionButton {
outline: none;
cursor: pointer;
font-size: 13px;
font-weight: bold;
padding: 6px 18px;
border-radius: 5px;
transition: 0.3s ease;
border: 2px solid #008cd6;
}
.selectAllButton {
color: #008cd6;
margin-right: 5px;
background-color: #151617;
}
.selectNoneButton,
.reverseSelectionButton {
color: #ffffff;
margin-left: 5px;
background-color: #008cd6;
}
}
.selectNoneButtonList {
width: 161px;
}
}
.optionList {
margin-top: 30px;
li {
width: 100%;
padding: 7px;
display: flex;
border-radius: 3px;
align-items: center;
box-sizing: border-box;
background-color: #2a2b2c;
input[type='checkbox'] {
width: 16px;
height: 16px;
}
span {
margin-left: 5px;
font-weight: bold;
}
}
li+li {
margin-top: 10px;
}
}
}
}
</style>
<script>
export default {
data () {
return {
SimulationData: [
{
dataContent: '模拟数据:唐僧',
isSelect: false
},
{
dataContent: '模拟数据:孙悟空',
isSelect: false
},
{
dataContent: '模拟数据:猪八戒',
isSelect: false
},
{
dataContent: '模拟数据:沙僧',
isSelect: false
},
{
dataContent: '模拟数据:白龙马',
isSelect: false
}
],
isAllSelect: false
}
},
methods: {
SelectOrCancel: function (itme) {
// itme.isSelect = !itme.isSelect // input标签不需要这句
const SelectedQuantity = this.SimulationData.filter((itme) => { return itme.isSelect }).length
// 优化前
// if (SelectedQuantity === this.SimulationData.length) {
// this.isAllSelect = true
// } else {
// this.isAllSelect = false
// }
// 优化后
this.isAllSelect = (SelectedQuantity === this.SimulationData.length)
},
SelectAllOrCancelAll: function () {
// 优化前
// if (this.isAllSelect === true) {
// this.SimulationData.forEach(SimulationDataAllSelect => { SimulationDataAllSelect.isSelect = false })
// this.isAllSelect = false
// } else {
// this.SimulationData.forEach(SimulationDataAllSelect => { SimulationDataAllSelect.isSelect = true })
// this.isAllSelect = true
// }
// 优化后
this.SimulationData.forEach(SimulationDataAllSelect => {
SimulationDataAllSelect.isSelect = !this.isAllSelect
})
this.isAllSelect = !this.isAllSelect
},
ReverseSelection: function () {
this.SimulationData.forEach(SimulationDataAllSelect => {
SimulationDataAllSelect.isSelect = !SimulationDataAllSelect.isSelect
this.SelectOrCancel(SimulationDataAllSelect)
})
}
}
}
</script>
更多推荐
所有评论(0)