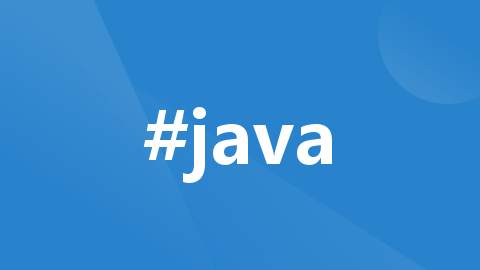
Maven 多仓库配置和优先级下载
在使用 Maven 下载依赖项时,有时需要配置多个镜像仓库或私有仓库,以满足按照仓库顺序下载依赖的需求,即当一个仓库找不到所需依赖项时,自动尝试从另一个仓库下载。本文将介绍几种方法来实现这一目标。
方法一:使用配置文件激活实现多仓库下载
在 Maven 配置文件中配置的 <mirrors>
部分定义了 Maven 镜像的设置。镜像的作用是在构建过程中,将对中央仓库(Central Repository)的访问请求重定向到镜像仓库,从而加速依赖项的下载过程,减轻中央仓库的负载,以及提供备用的下载源。例如:
<mirrors>
<mirror>
<id>repo2</id>
<mirrorOf>*</mirrorOf>
<name>Human Readable Name for this Mirror.</name>
<url>http://your-private-repo-url/repo</url>
</mirror>
<mirror>
<id>tencent</id>
<name>tencent maven mirror</name>
<url>https://mirrors.tencent.com/nexus/repository/maven-public/</url>
<mirrorOf>*</mirrorOf>
</mirror>
<mirror>
<id>alimaven</id>
<name>aliyun maven</name>
<url>http://maven.aliyun.com/nexus/content/groups/public/</url>
<mirrorOf>central</mirrorOf>
</mirror>
-
repo2 镜像:
<id>repo2</id>
: 这个镜像的唯一标识符,可以在 Maven 设置中引用它。<mirrorOf>*</mirrorOf>
:*
表示所有的仓库请求都会被重定向到这个镜像。<url>
http://your-private-repo-url/repo</url>
: 这是私有 Maven 仓库的地址,项目可以从这里下载依赖项。
-
aliyun 镜像:
<id>alimaven</id>
: 这个镜像的唯一标识符。<name>aliyun maven</name>
: 镜像的名称。<url>http://maven.aliyun.com/nexus/content/groups/public/</url>
: 这是阿里云的公共 Maven 镜像,用于加速从中央仓库下载依赖项。但这个镜像只会重定向对中央仓库的请求。
通过配置这些镜像,可以在构建项目时加速依赖项的下载。当 Maven 需要下载依赖项时,它会首先尝试从 repo2 镜像获取,如果 repo2 镜像没有所需的依赖项,那么 Maven 会尝试从 aliyun 镜像获取。这可以提高构建的效率,并且减少了对中央仓库的直接访问。
在 Maven 中,当配置了多个仓库地址时,默认情况下它会按照 <mirrors>
配置文件中的顺序尝试从这些仓库下载依赖项。一旦在某个仓库中找到所需的依赖项,Maven 就会停止搜索并下载依赖项。这意味着如果首选仓库(如阿里云的仓库)包含所需的依赖项,Maven 就不会尝试从其他仓库(如私有仓库)下载同一依赖项。
如果希望在首选仓库没有所需依赖项时尝试从备用仓库下载,可以使用 Maven 的 <profiles>
和 <repositories>
配置。以下是一种方式来实现这一目标:
1.在的 settings.xml
中,配置多个 <profiles>
,每个 <profile>
针对不同的仓库。例如:
<profiles>
<profile>
<id>repo2-profile</id>
<repositories>
<repository>
<id>repo2</id>
<url>http://your-private-repo-url/repo</url>
</repository>
</repositories>
</profile>
<profile>
<id>aliyun-profile</id>
<repositories>
<repository>
<id>alimaven</id>
<url>http://maven.aliyun.com/nexus/content/groups/public/</url>
</repository>
</repositories>
</profile>
</profiles>
2.在项目的 pom.xml
文件中,根据需要引入这些 <profiles>
。例如:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
<executions>
<execution>
<id>default-compile</id>
<phase>none</phase>
</execution>
<execution>
<id>default-testCompile</id>
<phase>none</phase>
</execution>
</executions>
</plugin>
</plugins>
</build>
<profiles>
<profile>
<id>repo2-profile</id>
<activation>
<activeByDefault>true</activeByDefault>
</activation>
<repositories>
<repository>
<id>repo2</id>
<url>http://your-private-repo-url/repo</url>
</repository>
</repositories>
</profile>
<profile>
<id>aliyun-profile</id>
<activation>
<activeByDefault>false</activeByDefault>
</activation>
<repositories>
<repository>
<id>alimaven</id>
<url>http://maven.aliyun.com/nexus/content/groups/public/</url>
</repository>
</repositories>
</profile>
</profiles>
上述配置中,<profiles>
部分定义了两个不同的配置文件,分别指向不同的仓库地址。然后,在项目的 pom.xml
文件中,使用 <activation>
来指定哪个 <profile>
应该被激活。在上面的例子中,repo2-profile
被设置为默认激活的 <profile>
,这意味着首选仓库是私有仓库。如果私有仓库中找不到依赖项,可以通过在 <profiles>
中将 aliyun-profile
激活来尝试从阿里云仓库下载。
可以根据实际需求和项目配置来调整这些配置。这种方式可以在多个仓库之间进行切换,以满足不同的依赖项需求。
方法二:在项目的 pom.xml 文件中明确指定私有仓库的配置
在项目的 pom.xml
文件中配置私有仓库的配置是一种简单有效的方式。可以在 <repositories>
部分中明确指定私有仓库的 URL 和其他信息。示例如下:
<repositories>
<repository>
<id>repo2</id>
<url>http://your-private-repo-url/repo</url>
</repository>
</repositories>
这将告诉 Maven 在构建项目时从指定的私有仓库中查找依赖项。如果私有仓库中找不到依赖项,Maven 会尝试从其他配置的仓库中下载。
方法三:使用 Maven 的 <profiles> 和 <activation> 配置
另一种方法是在 settings.xml
配置文件中使用 Maven 的 <profiles>
和 <activation>
配置,以为所有项目设置默认的仓库优先级。这样可以确保所有项目在使用相同的配置文件时具有相同的行为。
在 settings.xml
文件中定义多个 <profile>
,每个 <profile>
代表不同的仓库。示例如下:
<profiles>
<profile>
<id>repo2-profile</id>
<repositories>
<repository>
<id>repo2</id>
<url>http://your-private-repo-url/repo</url>
</repository>
</repositories>
</profile>
<profile>
<id>aliyun-profile</id>
<repositories>
<repository>
<id>alimaven</id>
<url>http://maven.aliyun.com/nexus/content/groups/public/</url>
</repository>
</repositories>
</profile>
</profiles>
在 settings.xml
中使用 <activeProfiles>
部分指定默认激活的仓库配置。示例如下:
<activeProfiles>
<!-- 指定默认的仓库优先级 -->
<activeProfile>repo2-profile</activeProfile>
</activeProfiles>
更多推荐
所有评论(0)