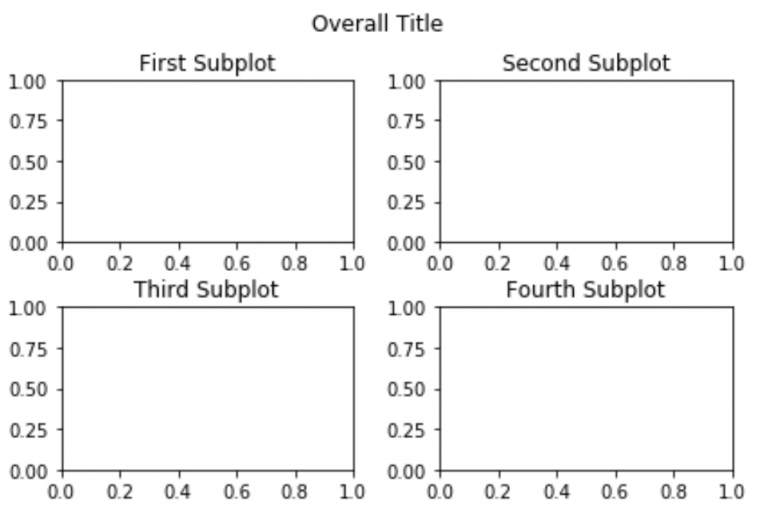
Matplotlib解决多子图时的间距问题
Matplotlib解决多子图时的间距问题
在Matplotlib中,经常使用子图功能,在同一个界面中并排显示多幅图。然而,使用默认设置的话,这些子图往往会相互重叠。
Often you may use subplots to display multiple plots alongside each
other in Matplotlib. Unfortunately, these subplots tend to overlap
each other by default.
解决这个问题的最简单方法是使用Matplotlib
的tight_layout()
函数。本教程解释如何在实践中使用这个函数。
The easiest way to resolve this issue is by using the Matplotlib
tight_layout() function. This tutorial explains how to use this
function in practice.
创建子图
Create Subplots
假设我们需要2列2行,共4个子图:
Consider the following arrangement of 4 subplots in 2 columns and 2
rows:
import matplotlib.pyplot as plt
#define subplots
fig, ax = plt.subplots(2, 2)
#display subplots
plt.show()
可以看出这些子图有一点重叠。
Notice how the subplots overlap each other a bit.
使用tight_layout()调整子图间距
Adjust Spacing of Subplots Using tight_layout()
要想解决子图重叠问题,最简单的办法就是使用tight_layout()
函数:
The easiest way to resolve this overlapping issue is by using the
Matplotlib tight_layout() function:
import matplotlib.pyplot as plt
#define subplots
fig, ax = plt.subplots(2, 2)
fig.tight_layout()
#display subplots
plt.show()
调整子图标题间距
Adjust Spacing of Subplot Titles
当我们给每个子图都添加标题之后,即使是使用了tight_layout()
也没法阻止重叠问题的出现:
In some cases you may also have titles for each of your subplots.
Unfortunately even the tight_layout() function tends to cause the
subplot titles to overlap:
import matplotlib.pyplot as plt
#define subplots
fig, ax = plt.subplots(2, 2)
fig.tight_layout()
#define subplot titles
ax[0, 0].set_title('First Subplot')
ax[0, 1].set_title('Second Subplot')
ax[1, 0].set_title('Third Subplot')
ax[1, 1].set_title('Fourth Subplot')
#display subplots
plt.show()
解决这个问题的方法是使用h_pad
参数来增加子图间距:
The way to resolve this issue is by increasing the height padding
between subplots using the h_pad argument:
import matplotlib.pyplot as plt
#define subplots
fig, ax = plt.subplots(2, 2)
fig.tight_layout(h_pad=2)
#define subplot titles
ax[0, 0].set_title('First Subplot')
ax[0, 1].set_title('Second Subplot')
ax[1, 0].set_title('Third Subplot')
ax[1, 1].set_title('Fourth Subplot')
#display subplots
plt.show()
调整总标题的间距
Adjust Spacing of Overall Title
如果想要避免总标题和子图标题重叠,subplots_adjust()
函数是个不错的选择:
If you have an overall title, you can use the subplots_adjust()
function to ensure that it doesn’t overlap with the subplot titles:
import matplotlib.pyplot as plt
#define subplots
fig, ax = plt.subplots(2, 2)
fig.tight_layout(h_pad=2)
#define subplot titles
ax[0, 0].set_title('First Subplot')
ax[0, 1].set_title('Second Subplot')
ax[1, 0].set_title('Third Subplot')
ax[1, 1].set_title('Fourth Subplot')
#add overall title and adjust it so that it doesn't overlap with subplot titles
fig.suptitle('Overall Title')
plt.subplots_adjust(top=0.85)
#display subplots
plt.show()
点击这里探索更多Matplotlib教程
You can find more Matplotlib tutorials here.
参考链接
更多推荐
所有评论(0)