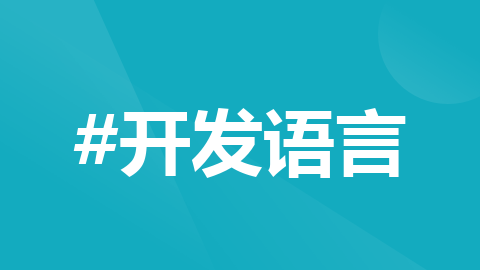
输入三个字符串,按由小到大的顺序输出。(C++程序设计-第四版)习题6.2(用指针或引用方法处理)
输入三个字符串,按由小到大的顺序输出。(C++程序设计-第四版)习题6.2(用指针或引用方法处理)

一键AI生成摘要,助你高效阅读
问答
·
在看本文时,需要提前了解:
1、strcpy(数组复制函数)在目前较新版本的编译器中已经失效;
2、strcpy_s(str1,str2)中第一个参数str1需要是常量。
本文用四种方法实现:
- 字符数组的指针方法处理;
//---------字符数组--指针方法---------- #include<iostream> #include<string> using namespace std; int main() { void sort(char*, char*, char*); char a[20], b[20], c[20]; char* p1, * p2, * p3; p1 = a, p2 = b, p3 = c; cout << "请输入3个字符串:" << endl; cout << "请输入字符串a:"; cin >> a; cout << "请输入字符串b:"; cin >> b; cout << "请输入字符串c:"; cin >> c; sort(p1, p2, p3); cout << "3个字符串由小到大排序为:" << endl; cout << a << " " << b << " " << c << endl; return 0; } void sort(char* p1, char* p2,char *p3)//排序函数; { void swap(char*, char*); if (strcmp(p1, p2) > 0)swap(p1, p2); if (strcmp(p1, p3) > 0)swap(p1, p3); if (strcmp(p2, p3) > 0)swap(p2, p3); } void swap(char* p1, char* p2)//****复制函数*****因为strcpy_s函数的第一个参数需要是常量,用在排序函数中是在进行strcpy_s(p1,p2)时无法处理,所以需要自建复制函数 { char t; if (strlen(p1) > strlen(p2))//进行复制时的两个字符串长度不同时,要分情况进行复制 { for (; *p2 != '\0'; p1++, p2++)//先按较短的字符串长度和另外一个字符串等长度互换 { t = *p2; *p2 = *p1; *p1 = t; } for (; *p1 != 0; p1++,p2++)//接着再将较长的字符串剩下的字符复制到较短字符数组中,原本较长的数组后续的字符则均变为空字符'\0' { *p2 = *p1; *p1 = '\0'; } *p2 = '\0'; } else { for (; *p1 != '\0'; p1++, p2++)//同上 { t = *p1; *p1 = *p2; *p2 = t; } for (; *p2 != 0; p1++,p2++) { *p1 = *p2; *p2 = '\0'; } *p1 = '\0'; } }
运行结果如下:
- 字符串的指针方法处理;
#include<iostream> #include<string> using namespace std; int main() { void swap(string*, string*); string a, b, c; string* p1, * p2, * p3; cout << "请输入3个字符串:" << endl; cout << "请输入字符串a:"; cin >> a; cout << "请输入字符串b:"; cin >> b; cout << "请输入字符串c:"; cin >> c; p1 = &a; p2 = &b; p3 = &c; if (a > b)swap(p1, p2); if (a > c)swap(p1, p3); if (b > c)swap(p2, p3); cout << "3个字符串由小到大排序为:" << endl; cout << a << " " << b << " " << c << endl; return 0; } void swap(string* p1, string* p2) { string temp; temp = *p2; *p2 = *p1; *p1 = temp; }
运行结果如下:
- 字符数组的引用方法处理;
/--------------------字符数组--引用方法------------- //---------------注意!!!-----不能建立引用的数组!但是可以建立数组的引用------------------ #include<iostream> #include<string> using namespace std; int main() { void sort(char(&p1)[20], char(&p2)[20], char(&p3)[20]);//形参改成数组的引用,编译器会传递数组的本身; char a[20], b[20], c[20]; cout << "请输入3个字符串:" << endl; cout << "请输入字符串a:"; cin >> a; cout << "请输入字符串b:"; cin >> b; cout << "请输入字符串c:"; cin >> c; sort(a, b, c); cout << "3个字符串按由小到大排序为:" << endl; cout << a << " " << b << " " << c << endl; } void sort(char(&p1)[20], char(&p2)[20], char(&p3)[20]) { char t[20]; if (strcmp(p1, p2) > 0) { strcpy_s(t, p1); strcpy_s(p1, p2); strcpy_s(p2, t); } if (strcmp(p1, p3) > 0) { strcpy_s(t, p1); strcpy_s(p1, p3); strcpy_s(p3, t); } if (strcmp(p2, p3) > 0) { strcpy_s(t, p2); strcpy_s(p2, p3); strcpy_s(p3, t); } }
- 字符串的引用方法处理。
//----------------字符串--引用方法------------- #include<iostream> #include<string> using namespace std; int main() { void sort(string &p1, string &p2, string &p3); string a, b, c; cout << "请输入3个字符串:" << endl; cout << "请输入字符串a:"; cin >> a; cout << "请输入字符串b:"; cin >> b; cout << "请输入字符串c:"; cin >> c; sort(a, b, c); cout << "字符串按由小到大排序为:" << endl; cout << a << " " << b << " " << c << endl; return 0; } void sort(string &p1, string &p2, string &p3) { string t; if (p1 > p2) { t = p2; p2 = p1; p1 = t; } if (p1 > p3) { t = p3; p3 = p1; p1 = t; } if (p2 > p3) { t = p3; p3 = p2; p2 = t; } }
对于C++程序设计——第四版的课后习题有疑问,均可通过QQ或e-mail与我进行探讨。
QQ:1033536725
e-mail:1033536725@qq.com
非诚勿扰!
更多推荐
所有评论(0)