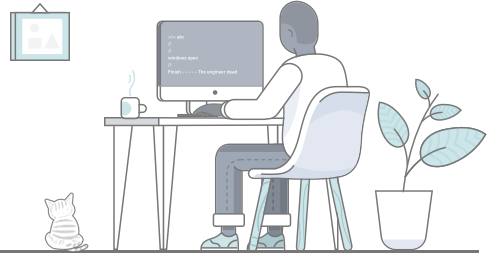
java集合 list转map一些常用的方式(Stream流,,,)
·
Java 集合之间的转换
java集合 list转map一些常用的方式(Stream流,,,)
提示:帮助文档
文章目录
- Java 集合之间的转换
- 前言
- 一、List转换为Map?
- 1.1、**List**<**Object**>**转化为Map<Integer,String>**
- 1.2 、**List**<**Object**>转化为Map<Integer,Object>
- 1.3、**List**<**Object**>转化为Map<Integer,List<**Object**>>
- 1.4 **List**<**Object**>转化为Map<Integer,List<**String**>>
- 1.5、 List<Map<String,Object>> 转化为Map<String,Object>
- 1.6、List<Map<String,String>> 转化为Map<String,Map<String,String>>
- 1.7、List<Map<String,String>> 转化为Map<String,String>
- 总结
前言
这片文章是用来整理开发中经常用到的一些集合之间的转换方法,作为笔记,提高开发效率,有需要的小伙伴可以参考一下,这片文章会慢慢补充完整。
提示:以下是本篇文章正文内容,下面案例可供参考
一、List转换为Map?
示例:代码案例(JAVA版)。
public class People (
private Integer id;
private String name;
private Integer age
private String address;
//TODO 构造方法和get和set方法省略
)
final static List<People> peopleList = new ArrayList<People>();
// 初始化集合数据
static {
People p1 = new People(0001, "张三", 12, "江苏南京");
People p2 = new People(0002, "李四", 14, "上海");
People p3 = new People(0003, "王二", 11, "浙江台州");
People p4 = new People(0004, "李五", 12, "河南郑州");
peopleList.add(p1);
peopleList.add(p2);
peopleList.add(p3);
peopleList.add(p4);
}
1.1、List<Object>转化为Map<Integer,String>
Map<Integer, String> map = peopleList.stream().collect(Collectors.toMap
(People::getId, People::getAddress, (value1, value2) -> value1));
1.2 、List<Object>转化为Map<Integer,Object>
Map<Integer, People> map = peopleList.stream().collect(Collectors.toMap
(People::getId, each -> each, (value1, value2) -> value1));
1.3、List<Object>转化为Map<Integer,List<Object>>
Map<Integer, List<People>> map = peopleList.stream().collect(Collectors
.groupingBy(People::getId));
1.4 List<Object>转化为Map<Integer,List<String>>
Map<Integer, List<String>> map3 = peopleList.stream().collect(Collectors.
toMap(People::getId, each -> Collections.singletonList(each.getName()),
(value1, value2) -> {
List<String> union = new ArrayList<>(value1);
union.addAll(value2);
return union;
}));
1.5、 List<Map<String,Object>> 转化为Map<String,Object>
final static List<Map<String, Object>> mapStudentList = new ArrayList<>();
public static void main(String[] args) {
Map<String, Object> map4 = mapStudentList.stream().collect(Collectors.toMap(each -> Objects.toString(each.get("id"), ""), each -> each.get("student"), (key1, key2) -> key1));
}
/**
* 初始化集合数据
*/
static {
Student stu1 = new Student("0001", "张三", 12, "江苏南京");
Student stu2 = new Student("0002", "李四", 14, "江苏无锡");
Student stu3 = new Student("0003", "王二", 11, "浙江台州");
Student stu4 = new Student("0004", "李五", 12, "浙江温州");
Map<String, Object> map1 = new HashMap<>();
map1.put("id", "0001");
map1.put("student", stu1);
Map<String, Object> map2 = new HashMap<>();
map2.put("id", "0002");
map2.put("student", stu2);
Map<String, Object> map3 = new HashMap<>();
map3.put("id", "0003");
map3.put("student", stu3);
Map<String, Object> map4 = new HashMap<>();
map4.put("id", "0004");
map4.put("student", stu4);
mapStudentList.add(map1);
mapStudentList.add(map2);
mapStudentList.add(map3);
mapStudentList.add(map4);
}
1.6、List<Map<String,String>> 转化为Map<String,Map<String,String>>
final static List<Map<String, String>> listMapList = new ArrayList<>();
public static void main(String[] args) {
Map<String, Map<String, String>> map5 = listMapList.stream().collect(Collectors.toMap(each -> each.get("id"), each -> each, (key1, key2) -> key1));
System.out.println("map5 = " + map5);
}
/**
* 初始化集合数据
*/
static {
Map<String, String> map1 = new HashMap<>();
map1.put("id", "0001");
map1.put("name", "张三");
map1.put("age", "12");
map1.put("address", "江苏南京");
Map<String, String> map2 = new HashMap<>();
map2.put("id", "0002");
map2.put("name", "李四");
map2.put("age", "14");
map2.put("address", "江苏无锡");
Map<String, String> map3 = new HashMap<>();
map3.put("id", "0003");
map3.put("name", "王二");
map3.put("age", "11");
map3.put("address", "浙江台州");
Map<String, String> map4 = new HashMap<>();
map4.put("id", "0004");
map4.put("name", "李五");
map4.put("age", "12");
map4.put("address", "浙江温州");
listMapList.add(map1);
listMapList.add(map2);
listMapList.add(map3);
listMapList.add(map4);
}
1.7、List<Map<String,String>> 转化为Map<String,String>
final static List<Map<String, String>> listmapstringlist = new ArrayList<>();
public static void main(String[] args) {
Map<String, String> map6 = listmapstringlist.stream().collect(Collectors.toMap(each -> each.get("id"), each -> each.get("name"), (key1, key2) -> key1));
}
/**
* 初始化集合数据
*/
static {
Map<String, String> map1 = new HashMap<>();
map1.put("id", "0001");
map1.put("name", "张三");
map1.put("age", "12");
map1.put("address", "江苏南京");
Map<String, String> map2 = new HashMap<>();
map2.put("id", "0002");
map2.put("name", "李四");
map2.put("age", "14");
map2.put("address", "江苏无锡");
Map<String, String> map3 = new HashMap<>();
map3.put("id", "0003");
map3.put("name", "王二");
map3.put("age", "11");
map3.put("address", "浙江台州");
Map<String, String> map4 = new HashMap<>();
map4.put("id", "0004");
map4.put("name", "李五");
map4.put("age", "12");
map4.put("address", "浙江温州");
listmapstringlist.add(map1);
listmapstringlist.add(map2);
listmapstringlist.add(map3);
listmapstringlist.add(map4);
}
总结
先总结这么多吧;
日积月累,小溪汇大海
更多推荐
所有评论(0)